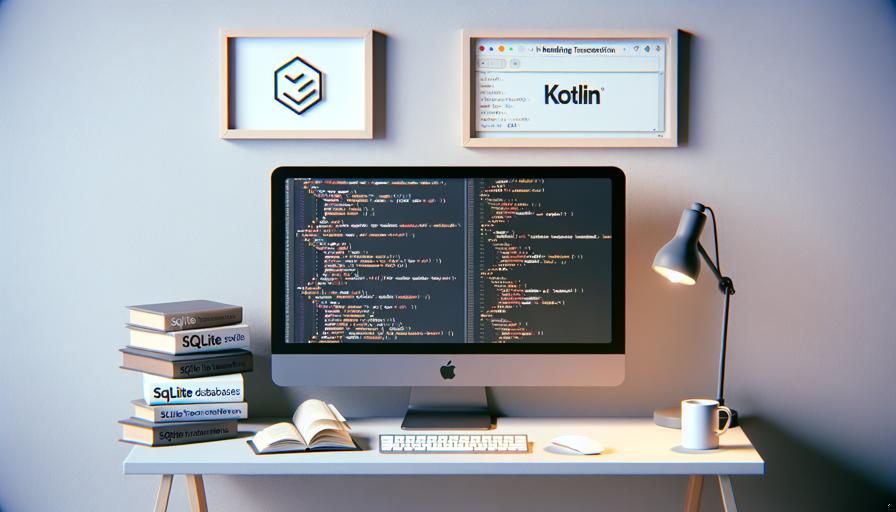
28.8. Working with SQLite Databases: Handling Transactions in SQLite
Page 36 | Listen in audio
```html
When developing Android applications, managing data efficiently is crucial for maintaining performance and ensuring data integrity. SQLite, a lightweight, disk-based database, is often the go-to choice for local data storage in Android apps. One of the most powerful features of SQLite is its support for transactions, which allows developers to execute a series of database operations atomically. This means that all operations in a transaction are completed successfully, or none of them are applied, ensuring data consistency even in the event of an error or system crash.
Understanding how to handle transactions effectively in SQLite is essential for any Android developer. Transactions can be particularly useful when you're performing multiple related operations that must succeed or fail as a unit. For example, when transferring money between bank accounts, you need to ensure that the withdrawal from one account and the deposit into another both occur, or neither do. This is where transactions come into play.
Initiating a Transaction
To start a transaction in SQLite, you use the BEGIN TRANSACTION
statement. In Android, this is typically done using the SQLiteDatabase
class. The beginTransaction()
method marks the start of a transaction. Here’s how you can initiate a transaction:
SQLiteDatabase db = this.getWritableDatabase();
db.beginTransaction();
try {
// Your database operations here
} finally {
db.endTransaction();
}
It's crucial to ensure that endTransaction()
is always called to avoid leaving the database in a locked state. Typically, this is done in a finally
block to guarantee execution even if an exception occurs.
Committing a Transaction
Once all operations within a transaction have been executed successfully, you can commit the transaction using the setTransactionSuccessful()
method. This method must be called before endTransaction()
. If setTransactionSuccessful()
is not called, the transaction will be rolled back when endTransaction()
is called.
db.beginTransaction();
try {
// Execute your database operations
db.setTransactionSuccessful(); // Marks the transaction as successful
} finally {
db.endTransaction(); // Commits the transaction if successful, otherwise rolls back
}
By marking the transaction as successful, you ensure that all operations are committed to the database. If an error occurs and setTransactionSuccessful()
is not called, the transaction will be automatically rolled back, undoing all changes made during the transaction.
Rolling Back a Transaction
Rolling back a transaction is an integral part of handling transactions, especially when an error occurs during one of the operations. If any operation within the transaction fails, you can simply not call setTransactionSuccessful()
, and the transaction will be rolled back automatically when endTransaction()
is called. This ensures that no partial changes are left in the database, maintaining data integrity.
Consider the following scenario:
db.beginTransaction();
try {
// Perform operation 1
// Perform operation 2
if (someConditionFails) {
throw new RuntimeException("Transaction failed");
}
db.setTransactionSuccessful();
} catch (Exception e) {
// Handle the exception
} finally {
db.endTransaction();
}
In this example, if someConditionFails
evaluates to true, an exception is thrown, and setTransactionSuccessful()
is not called. As a result, when endTransaction()
is executed, the transaction is rolled back, and the database remains unchanged.
Nesting Transactions
SQLite supports nesting transactions, which can be useful in complex operations where multiple transactions are required. However, nested transactions in SQLite are handled using savepoints rather than true nested transactions. When you begin a transaction while another transaction is active, SQLite creates a savepoint instead.
Here’s how you can implement nested transactions:
db.beginTransaction();
try {
// Perform outer transaction operations
db.beginTransaction(); // This creates a savepoint
try {
// Perform inner transaction operations
db.setTransactionSuccessful(); // Marks the inner transaction as successful
} finally {
db.endTransaction(); // Ends the inner transaction
}
db.setTransactionSuccessful(); // Marks the outer transaction as successful
} finally {
db.endTransaction(); // Ends the outer transaction
}
In this setup, if the inner transaction fails, only its operations are rolled back to the savepoint, while the outer transaction can still succeed if marked as successful. However, if the outer transaction fails, both the outer and inner transactions are rolled back entirely.
Best Practices for Handling Transactions
- Keep Transactions Short: Transactions should be as short as possible to reduce the time the database is locked, improving concurrency and performance.
- Handle Exceptions: Always handle exceptions within transactions to ensure that
endTransaction()
is called, preventing the database from being locked indefinitely. - Use Transactions Judiciously: Not all operations need to be wrapped in a transaction. Use them when you need atomicity across multiple operations.
- Test Thoroughly: Test your transaction logic under various conditions to ensure that it behaves correctly, especially in edge cases.
By understanding and implementing these principles, you can effectively manage SQLite transactions in your Android app, ensuring data consistency and reliability. Transactions are a powerful tool in your database management arsenal, providing the means to execute complex operations safely and efficiently.
```Now answer the exercise about the content:
What is the primary benefit of using transactions in SQLite when developing Android applications?
You are right! Congratulations, now go to the next page
You missed! Try again.
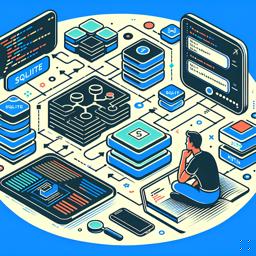
Next page of the Free Ebook: