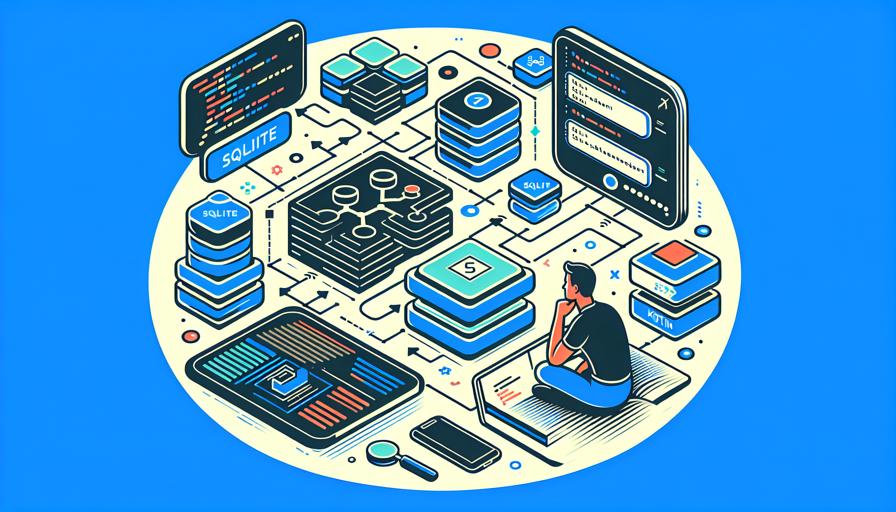
28.9. Working with SQLite Databases: Database Schema Design
Page 37 | Listen in audio
When developing Android applications, managing data efficiently is crucial, and SQLite databases offer a robust solution for local data storage. SQLite is a lightweight, serverless database engine that is embedded into Android, making it an ideal choice for apps that require persistent data storage. In this section, we’ll delve into the intricacies of working with SQLite databases, with a particular focus on database schema design.
Designing a database schema is a critical step in the development of an application. It involves defining how data is organized and how the relationships between different data elements are managed. A well-designed schema can improve the performance of your application, make it easier to maintain, and ensure data integrity.
Understanding Database Schema
A database schema is essentially a blueprint of how the database is structured. It defines the tables, fields, relationships, views, indexes, and other elements. The schema is pivotal as it dictates how data is stored, retrieved, and manipulated. In SQLite, the schema is defined using SQL (Structured Query Language) statements such as CREATE TABLE
, ALTER TABLE
, and CREATE INDEX
.
Key Components of a Database Schema
- Tables: These are the fundamental structures that hold data. Each table consists of rows and columns, where each column represents a data field, and each row represents a data record.
- Fields: Also known as columns, these define the type of data that can be stored in each table. Common data types include
INTEGER
,TEXT
,REAL
,BLOB
, andNULL
. - Primary Keys: A primary key is a unique identifier for each record in a table. It ensures that each record can be uniquely identified, which is essential for data integrity.
- Foreign Keys: These are used to establish relationships between tables. A foreign key in one table points to a primary key in another table, creating a link between the two tables.
- Indexes: Indexes are used to speed up the retrieval of data. They are particularly useful for columns that are frequently searched or sorted.
Designing a Database Schema
When designing a database schema, several considerations must be taken into account to ensure that the database is efficient, scalable, and maintainable. Here are some best practices:
1. Normalize Your Data
Normalization is the process of organizing data to minimize redundancy and improve data integrity. It involves dividing large tables into smaller, related tables and defining relationships between them. The goal is to ensure that each piece of data is stored only once, which reduces the risk of data anomalies.
2. Define Clear Relationships
Clearly defining relationships between tables is crucial for maintaining data integrity. Use foreign keys to establish these relationships, and ensure that the database enforces referential integrity. This means that any foreign key field must agree with the primary key that is referenced by the foreign key.
3. Choose Appropriate Data Types
Choosing the right data types for your fields can significantly impact the performance and storage requirements of your database. Use data types that closely match the nature of the data you are storing. For example, use INTEGER
for whole numbers and TEXT
for strings.
4. Index Strategically
Indexes can greatly enhance the performance of your database, especially for queries that involve searching or sorting. However, they also consume additional storage space and can slow down write operations. Therefore, it’s important to strike a balance and only index columns that are frequently used in queries.
5. Plan for Scalability
Consider the future growth of your application and design your schema to accommodate increased data volumes. This might involve partitioning tables, optimizing queries, and ensuring that the schema can be easily extended without significant refactoring.
Implementing the Schema in SQLite
Once you have designed your database schema, the next step is to implement it in SQLite. This involves writing SQL statements to create tables, define relationships, and set up indexes. Here’s a basic example of how to create a simple database schema in SQLite:
-- Create a table for users
CREATE TABLE Users (
UserID INTEGER PRIMARY KEY AUTOINCREMENT,
Username TEXT NOT NULL,
Email TEXT NOT NULL UNIQUE,
Password TEXT NOT NULL
);
-- Create a table for user profiles
CREATE TABLE UserProfiles (
ProfileID INTEGER PRIMARY KEY AUTOINCREMENT,
UserID INTEGER NOT NULL,
FirstName TEXT,
LastName TEXT,
FOREIGN KEY(UserID) REFERENCES Users(UserID)
);
-- Create an index on the Username column
CREATE INDEX idx_username ON Users(Username);
In this example, we have two tables: Users
and UserProfiles
. The Users
table stores information about each user, while the UserProfiles
table stores additional profile information. The UserID
field in the UserProfiles
table is a foreign key that references the UserID
field in the Users
table, establishing a relationship between the two tables.
Managing Schema Changes
As your application evolves, you may need to modify the database schema to accommodate new features or changes in requirements. Managing schema changes can be challenging, especially if your application is already in production. Here are some strategies to handle schema changes effectively:
1. Use Versioning
SQLite supports database versioning, which allows you to track changes to the schema over time. When you make changes to the schema, increment the database version number and implement logic to migrate the existing data to the new schema. This ensures that your application can upgrade the database without losing data.
2. Write Migration Scripts
Migration scripts are SQL scripts that define the changes needed to transition from one schema version to another. These scripts can include commands to add or remove tables, alter existing tables, and update data. By automating the migration process, you can reduce the risk of errors and ensure a smooth transition.
3. Test Thoroughly
Before deploying schema changes to production, thoroughly test the migration process in a development environment. This includes verifying that data integrity is maintained and that the application functions correctly with the new schema.
Conclusion
Designing and managing a database schema in SQLite is a fundamental aspect of Android app development. A well-designed schema not only enhances the performance and scalability of your application but also ensures data integrity and ease of maintenance. By following best practices for schema design and effectively managing schema changes, you can build robust and efficient applications that meet the needs of your users.
In the next sections, we will explore more advanced topics related to SQLite in Android, including working with Content Providers, using Room persistence library, and optimizing database performance. These concepts will further enhance your ability to manage data in Android applications effectively.
Now answer the exercise about the content:
What is a primary key in the context of a database schema?
You are right! Congratulations, now go to the next page
You missed! Try again.
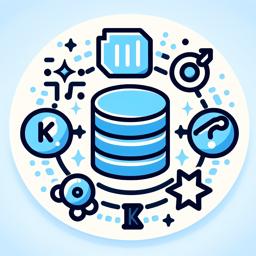
Next page of the Free Ebook: