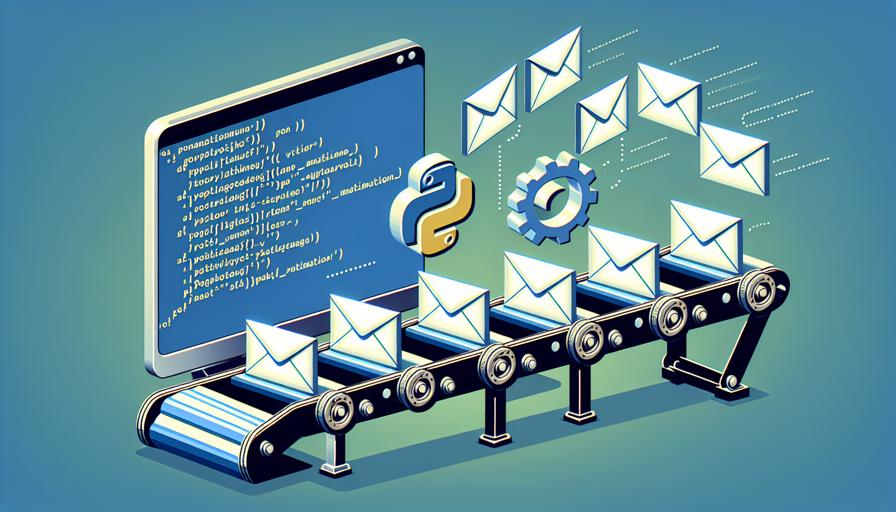
22.3. Sending Automated Emails with Python: Python Libraries for Email Automation
Page 45 | Listen in audio
22.3. Sending Automated Emails with Python: Python Libraries for Email Automation
In today's fast-paced digital world, email remains one of the most reliable and widely used forms of communication. Whether it's for business, personal use, or marketing purposes, the ability to send emails efficiently can save time and improve productivity. Python, with its extensive library support and ease of use, offers powerful tools for automating email tasks. In this section, we'll explore various Python libraries that can help you automate the process of sending emails, making your life easier and more efficient.
Understanding the Basics of Email Automation
Email automation involves using software to send emails automatically based on predefined triggers or schedules. This can include sending newsletters, notifications, reminders, or any other type of email without manual intervention. Python provides several libraries that make it easy to automate email sending, each with its own strengths and features.
Popular Python Libraries for Email Automation
1. smtplib
smtplib
is a built-in Python library that provides a simple way to send emails using the Simple Mail Transfer Protocol (SMTP). It is part of the Python standard library, which means you don't need to install any additional packages to use it. Here's a basic example of how to send an email using smtplib
:
import smtplib
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
# Define email sender and server details
from_email = '[email protected]'
smtp_server = 'smtp.example.com'
smtp_port = 587
smtp_user = '[email protected]'
smtp_password = 'your_password'
# Create the email content
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
# Connect to the SMTP server and send the email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(smtp_user, smtp_password)
server.sendmail(from_email, to_email, msg.as_string())
# Example usage
send_email('Test Subject', 'This is a test email.', '[email protected]')
While smtplib
is straightforward and easy to use for basic email sending, it requires manual handling of email content and lacks advanced features like handling attachments or HTML content.
2. email
The email
library, also part of the Python standard library, is used in conjunction with smtplib
to create more complex email messages. It provides tools to construct and parse email messages, including support for attachments and HTML content. Here's an example of sending an HTML email with an attachment:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(subject, body, to_email, attachment_path):
from_email = '[email protected]'
smtp_server = 'smtp.example.com'
smtp_port = 587
smtp_user = '[email protected]'
smtp_password = 'your_password'
# Create the email content
msg = MIMEMultipart()
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
# Attach the email body
msg.attach(MIMEText(body, 'html'))
# Attach a file
with open(attachment_path, 'rb') as attachment:
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename="{attachment_path}"')
msg.attach(part)
# Connect to the SMTP server and send the email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(smtp_user, smtp_password)
server.sendmail(from_email, to_email, msg.as_string())
# Example usage
send_email_with_attachment('Test Subject', 'This is a test email.
', '[email protected]', 'path/to/attachment.txt')
This example demonstrates how to send an email with HTML content and an attachment using the email
library. This library provides more flexibility and is better suited for more complex email automation tasks.
3. yagmail
yagmail
is a third-party library that simplifies the process of sending emails in Python. It is designed to make email sending as easy as possible, with built-in support for HTML content and attachments. To use yagmail
, you need to install it first:
pip install yagmail
Here's an example of using yagmail
to send an email:
import yagmail
def send_email(subject, body, to_email, attachment_path=None):
yag = yagmail.SMTP('[email protected]', 'your_password')
contents = [body]
if attachment_path:
contents.append(attachment_path)
yag.send(to=to_email, subject=subject, contents=contents)
# Example usage
send_email('Test Subject', 'This is a test email.
', '[email protected]', 'path/to/attachment.txt')
yagmail
automatically handles the complexities of email sending, such as formatting HTML content and attaching files. It is an excellent choice for users who want a simple and effective solution for email automation.
4. Flask-Mail
If you're developing a web application using Flask and need to integrate email functionality, Flask-Mail
is a great option. It provides seamless integration with Flask and simplifies the process of sending emails from your web app. Here's how to set it up:
from flask import Flask
from flask_mail import Mail, Message
app = Flask(__name__)
# Configure Flask-Mail
app.config['MAIL_SERVER'] = 'smtp.example.com'
app.config['MAIL_PORT'] = 587
app.config['MAIL_USERNAME'] = '[email protected]'
app.config['MAIL_PASSWORD'] = 'your_password'
app.config['MAIL_USE_TLS'] = True
mail = Mail(app)
@app.route('/send_email')
def send_email():
msg = Message('Test Subject', sender='[email protected]', recipients=['[email protected]'])
msg.body = 'This is a test email.'
msg.html = 'This is a test email.
'
mail.send(msg)
return 'Email sent!'
if __name__ == '__main__':
app.run()
With Flask-Mail
, you can easily send emails from your Flask routes, making it ideal for web applications that require email notifications or confirmations.
Conclusion
Automating email tasks with Python can significantly enhance your productivity and streamline communication processes. Whether you're sending simple notifications or complex HTML emails with attachments, Python's rich ecosystem of libraries provides the tools you need to automate these tasks efficiently. From the simplicity of smtplib
to the elegance of yagmail
and the web integration capabilities of Flask-Mail
, there's a solution to fit every use case. By leveraging these libraries, you can focus on what matters most while letting Python handle the repetitive tasks of email communication.
Now answer the exercise about the content:
Which Python library is part of the standard library and provides a simple way to send emails using the Simple Mail Transfer Protocol (SMTP)?
You are right! Congratulations, now go to the next page
You missed! Try again.
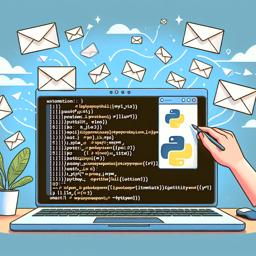
Next page of the Free Ebook: