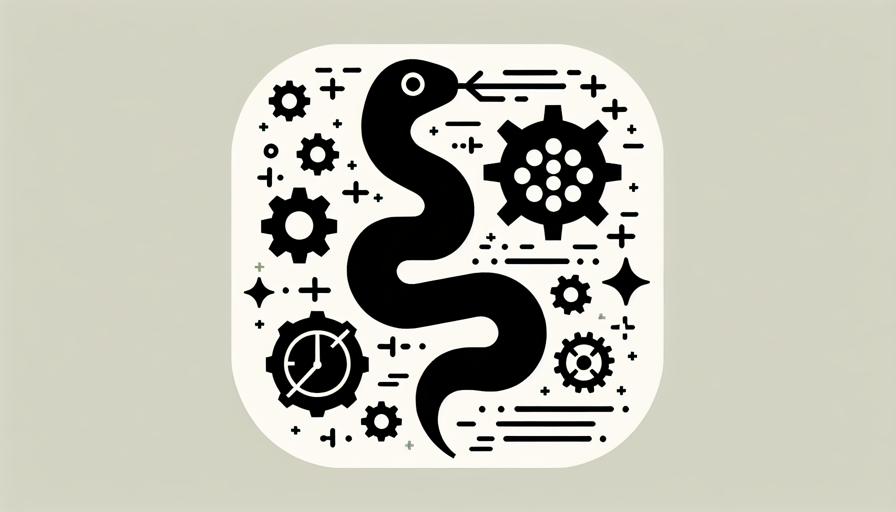
42. Performance Optimization for Automation
Page 92 | Listen in audio
Performance Optimization for Automation
In the realm of automating everyday tasks with Python, performance optimization is a crucial aspect that ensures your scripts run efficiently and complete tasks in a timely manner. As automation tasks become more complex and handle larger datasets, the need for optimization becomes increasingly important. This chapter will delve into various strategies and techniques to optimize the performance of your Python automation scripts.
Understanding Performance Bottlenecks
Before diving into optimization techniques, it's essential to identify the performance bottlenecks in your automation scripts. Bottlenecks are parts of your code that significantly slow down execution. Common bottlenecks include inefficient algorithms, excessive I/O operations, and poor data handling. Tools such as Python's built-in cProfile
and timeit
modules can help profile your code and pinpoint areas that need improvement.
Using cProfile
The cProfile
module provides a detailed report on the time spent in each function of your script. By analyzing this report, you can identify which functions are the most time-consuming and focus your optimization efforts there. Here's a basic example of how to use cProfile
:
import cProfile
def my_function():
# Your code here
cProfile.run('my_function()')
Using timeit
The timeit
module is useful for timing small code snippets and comparing the performance of different implementations. For instance, if you're unsure whether to use a list comprehension or a loop, timeit
can help you decide:
import timeit
list_comp_time = timeit.timeit('[x for x in range(1000)]', number=1000)
loop_time = timeit.timeit('for x in range(1000): pass', number=1000)
print(f"List comprehension time: {list_comp_time}")
print(f"Loop time: {loop_time}")
Optimizing Code Structure
Once you've identified the bottlenecks, the next step is to optimize the structure of your code. This involves choosing the right algorithms and data structures, minimizing redundant operations, and ensuring efficient use of resources.
Choosing the Right Algorithm
The choice of algorithm can have a significant impact on performance. For example, sorting a list using a built-in method is often faster than implementing your own sorting algorithm. Familiarize yourself with Python's standard library, which offers efficient implementations for many common tasks.
Using Efficient Data Structures
Choosing the right data structure can drastically improve performance. For example, if you need fast lookups, consider using a dictionary or a set instead of a list. Understanding the time complexity of different operations on various data structures will help you make informed decisions.
Minimizing Redundant Operations
Redundant operations can slow down your script significantly. Avoid unnecessary calculations and repetitive tasks. For example, if a value is computed multiple times, store it in a variable instead of recalculating it each time.
Leveraging Built-in Functions and Libraries
Python's standard library and third-party libraries are optimized for performance and should be utilized whenever possible. Functions from libraries like NumPy, Pandas, and itertools are often faster than custom implementations.
Using NumPy for Numerical Operations
NumPy is a powerful library for numerical processing. It provides efficient implementations for array operations, which can be orders of magnitude faster than pure Python loops. If your automation task involves heavy numerical computation, consider using NumPy:
import numpy as np
arr = np.array([1, 2, 3, 4])
result = np.sum(arr)
Utilizing Pandas for Data Manipulation
Pandas is an excellent library for data manipulation and analysis. It offers optimized data structures like DataFrames, which can handle large datasets efficiently. Pandas functions are vectorized, meaning they operate on entire arrays instead of element-wise, providing significant performance gains:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
result = df['A'] + df['B']
Parallel and Asynchronous Execution
For tasks that can be executed concurrently, parallel and asynchronous execution can lead to substantial performance improvements. Python offers several modules to facilitate this, such as concurrent.futures
, multiprocessing
, and asyncio
.
Using concurrent.futures
The concurrent.futures
module provides a high-level interface for asynchronously executing callables. It supports both thread and process pools, allowing you to choose the best option based on your task's nature:
from concurrent.futures import ThreadPoolExecutor
def task(n):
return n * n
with ThreadPoolExecutor() as executor:
results = executor.map(task, range(10))
Implementing Asynchronous Code with asyncio
For I/O-bound tasks, asyncio
allows you to write asynchronous code using the async
and await
keywords. This can significantly reduce the time spent waiting for I/O operations to complete:
import asyncio
async def fetch_data():
await asyncio.sleep(1)
return "Data"
async def main():
result = await fetch_data()
print(result)
asyncio.run(main())
Memory Management and Garbage Collection
Efficient memory management is another critical aspect of performance optimization. Python's garbage collector automatically manages memory, but understanding how it works can help you write more efficient code.
Reducing Memory Usage
Reduce memory usage by using generators instead of lists for large datasets. Generators yield items one at a time and do not store the entire dataset in memory:
def data_generator():
for i in range(1000):
yield i
for data in data_generator():
print(data)
Managing Garbage Collection
Python's garbage collector can sometimes introduce performance overhead. You can manually manage garbage collection using the gc
module to optimize performance:
import gc
gc.disable()
# Your code here
gc.collect()
gc.enable()
Conclusion
Performance optimization is a vital component of automating everyday tasks with Python. By identifying bottlenecks, choosing efficient algorithms and data structures, leveraging built-in functions, utilizing parallel and asynchronous execution, and managing memory effectively, you can ensure that your automation scripts run efficiently. As you continue to develop more complex automation tasks, these optimization techniques will become invaluable tools in your programming arsenal.
Now answer the exercise about the content:
What is a common method used to identify performance bottlenecks in Python automation scripts?
You are right! Congratulations, now go to the next page
You missed! Try again.
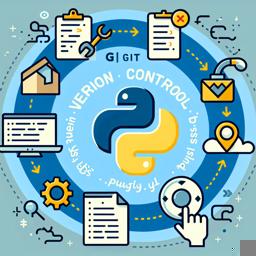
Next page of the Free Ebook: