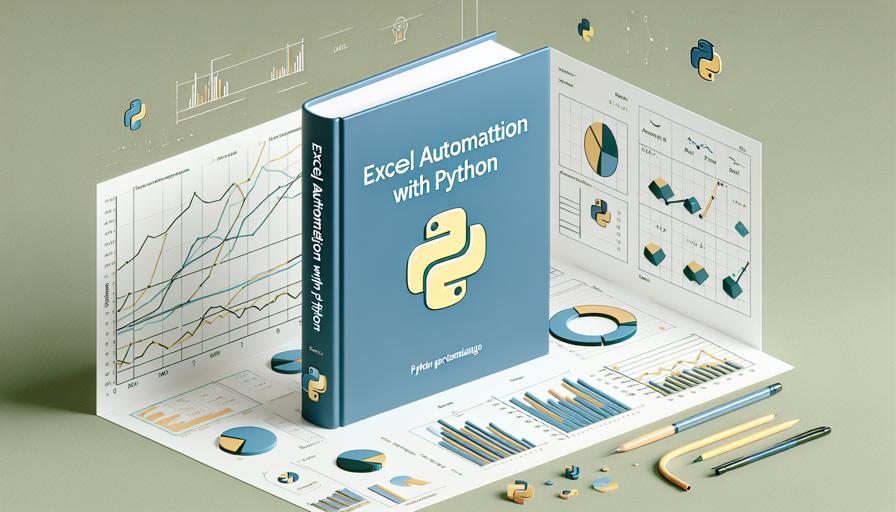
12.14. Excel Automation with Python: Excel Automation for Financial Analysis
Page 26 | Listen in audio
Excel is a staple tool in the world of finance, offering powerful capabilities for data organization, analysis, and visualization. However, as datasets grow and financial models become more complex, manual processing in Excel can become time-consuming and error-prone. This is where Python comes into play, offering robust libraries that can automate Excel tasks, streamline workflows, and enhance the accuracy of financial analysis.
Why Automate Excel for Financial Analysis?
Financial analysts often deal with large volumes of data, requiring repetitive tasks such as data cleaning, transformation, and report generation. Automating these tasks with Python not only saves time but also reduces the risk of human error. Python can handle complex calculations, create dynamic reports, and integrate data from multiple sources seamlessly.
Moreover, Python's open-source nature and extensive community support provide access to numerous libraries and tools tailored for financial analysis. This allows analysts to build custom solutions that cater specifically to their needs, enhancing productivity and insights.
Getting Started with Excel Automation in Python
To automate Excel tasks using Python, the primary library to consider is pandas. Pandas is a powerful data manipulation library that provides data structures and functions needed to work with structured data seamlessly. Additionally, openpyxl and xlrd are popular libraries for reading and writing Excel files.
Setting Up Your Environment
Before diving into automation, ensure that you have Python installed on your system along with the necessary libraries. You can install pandas and openpyxl using pip:
pip install pandas openpyxl
Once installed, you are ready to start automating Excel tasks. Let's explore some common financial analysis tasks that can be automated using Python.
Automating Data Import and Cleaning
Data cleaning is a crucial step in financial analysis. Python can automate the import and cleaning of data from Excel files, ensuring that the data is ready for analysis. Here's an example of how to read an Excel file, clean the data, and prepare it for analysis:
import pandas as pd
# Load the Excel file
df = pd.read_excel('financial_data.xlsx', sheet_name='Sheet1')
# Drop missing values
df.dropna(inplace=True)
# Convert data types if necessary
df['Date'] = pd.to_datetime(df['Date'])
df['Revenue'] = df['Revenue'].astype(float)
# Filter data for a specific time period
filtered_data = df[(df['Date'] >= '2023-01-01') & (df['Date'] <= '2023-12-31')]
In this example, we load data from an Excel file, handle missing values, convert data types, and filter the data for a specific time period. Automating these steps ensures consistency and accuracy in data preparation.
Automating Financial Calculations
Python can perform complex financial calculations that are often required in analysis. For instance, calculating key financial metrics like the compound annual growth rate (CAGR) or net present value (NPV) can be automated with Python:
def calculate_cagr(start_value, end_value, periods):
return (end_value / start_value) ** (1 / periods) - 1
# Example usage
start_revenue = 100000
end_revenue = 150000
years = 5
cagr = calculate_cagr(start_revenue, end_revenue, years)
print(f'CAGR: {cagr:.2%}')
By defining functions for financial calculations, you can reuse them across different analyses, ensuring consistency and reducing manual errors.
Automating Report Generation
Generating reports is a routine task in financial analysis. Python can automate the creation of Excel reports, complete with charts and tables, using libraries like openpyxl:
from openpyxl import Workbook
from openpyxl.chart import LineChart, Reference
# Create a new workbook and select the active worksheet
wb = Workbook()
ws = wb.active
# Add data to the worksheet
data = [
['Year', 'Revenue'],
[2018, 100000],
[2019, 120000],
[2020, 130000],
[2021, 150000],
[2022, 170000],
]
for row in data:
ws.append(row)
# Create a line chart
chart = LineChart()
chart.title = "Revenue Growth"
chart.x_axis.title = "Year"
chart.y_axis.title = "Revenue"
# Reference the data for the chart
data_ref = Reference(ws, min_col=2, min_row=1, max_row=6, max_col=2)
categories_ref = Reference(ws, min_col=1, min_row=2, max_row=6)
chart.add_data(data_ref, titles_from_data=True)
chart.set_categories(categories_ref)
# Add the chart to the worksheet
ws.add_chart(chart, "E5")
# Save the workbook
wb.save("financial_report.xlsx")
This script demonstrates how to create an Excel report with a line chart, showcasing revenue growth over the years. Automating report generation can significantly reduce the time spent on repetitive tasks, allowing analysts to focus on interpreting the results.
Integrating Data from Multiple Sources
Financial analysis often requires data integration from various sources such as databases, APIs, and other Excel files. Python's versatility allows seamless integration of these data sources, ensuring comprehensive analysis:
# Example of combining data from multiple Excel files
df1 = pd.read_excel('financial_data_1.xlsx')
df2 = pd.read_excel('financial_data_2.xlsx')
# Merge the dataframes on a common column
merged_df = pd.merge(df1, df2, on='ID')
By automating data integration, analysts can ensure that they are working with the most up-to-date and complete datasets, leading to more accurate insights.
Conclusion
Automating Excel tasks with Python can transform the way financial analysis is conducted. By leveraging Python's powerful libraries, financial analysts can streamline workflows, reduce errors, and focus on deriving actionable insights from data. As the financial industry continues to evolve, embracing automation will be key to staying competitive and efficient.
Whether you are a seasoned analyst or just starting in the field, mastering Excel automation with Python is a valuable skill that can enhance your analytical capabilities and open new opportunities in the world of finance.
Now answer the exercise about the content:
What is the primary library recommended for automating Excel tasks in Python according to the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
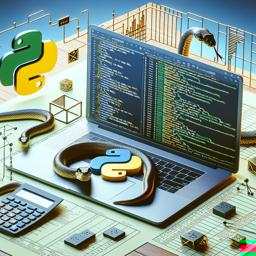
Next page of the Free Ebook: