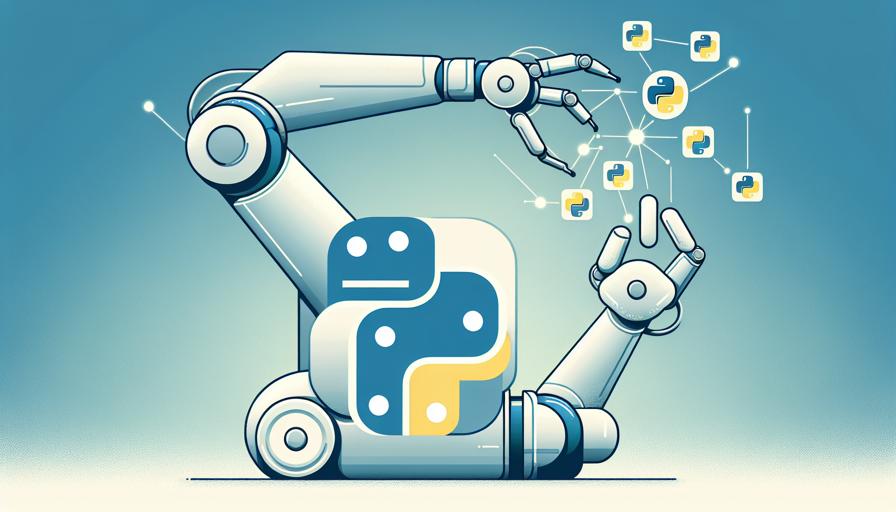
37. Enhancing Automation with Decorators
Page 87 | Listen in audio
In the realm of Python programming, decorators are a powerful tool that can be used to enhance and extend the functionality of functions or methods without modifying their actual code. When it comes to automating everyday tasks, decorators can play a pivotal role by adding layers of functionality, such as logging, access control, or even retry mechanisms, to existing functions. In this section, we delve into how decorators can be leveraged to enhance automation in Python.
Understanding Decorators
At its core, a decorator is a function that takes another function as an argument, adds some kind of functionality, and returns a new function. This allows you to "wrap" a function with additional behavior. Decorators are a form of metaprogramming, as they can manipulate the behavior of functions and methods at runtime.
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In the example above, the @my_decorator
syntax is a shorthand for say_hello = my_decorator(say_hello)
. When say_hello()
is called, it is actually executing the wrapper
function, which adds behavior before and after calling the original say_hello
function.
Using Decorators for Logging
One common use of decorators in automation is logging. By wrapping functions with a logging decorator, you can automatically log when a function is entered, exited, and any exceptions it raises. This can be incredibly useful for debugging automated scripts or monitoring their performance.
import logging
def log_function_call(func):
def wrapper(*args, **kwargs):
logging.info(f"Calling function {func.__name__} with arguments {args} and {kwargs}")
result = func(*args, **kwargs)
logging.info(f"Function {func.__name__} returned {result}")
return result
return wrapper
@log_function_call
def add(a, b):
return a + b
add(10, 20)
In this example, every call to the add
function will be logged, including the arguments passed and the result returned. This is particularly useful in automated systems where understanding the flow of data through functions is crucial.
Implementing Access Control
Another powerful application of decorators is access control. In an automated system, you may want to restrict access to certain functions based on user roles or permissions. Decorators can be used to enforce these rules at the function level.
def requires_permission(permission):
def decorator(func):
def wrapper(user, *args, **kwargs):
if user.has_permission(permission):
return func(user, *args, **kwargs)
else:
raise PermissionError(f"User {user.username} does not have {permission} permission.")
return wrapper
return decorator
@requires_permission('admin')
def delete_user(user, user_to_delete):
print(f"User {user_to_delete.username} deleted by {user.username}")
# Example usage
# delete_user(admin_user, target_user)
In this scenario, the requires_permission
decorator checks if the user has the necessary permission before allowing the function to execute. This is a clean and reusable way to enforce security policies in your automated tasks.
Retry Mechanisms for Fault Tolerance
Automated systems often need to interact with external services, which can be unreliable. To make your automation scripts more robust, you can use decorators to implement retry mechanisms, automatically retrying a function if it fails due to a transient error.
import time
import random
def retry(max_attempts=3, delay=1):
def decorator(func):
def wrapper(*args, **kwargs):
attempts = 0
while attempts < max_attempts:
try:
return func(*args, **kwargs)
except Exception as e:
attempts += 1
print(f"Attempt {attempts} failed: {e}. Retrying in {delay} seconds...")
time.sleep(delay)
raise Exception(f"Function {func.__name__} failed after {max_attempts} attempts.")
return wrapper
return decorator
@retry(max_attempts=5, delay=2)
def unreliable_task():
if random.choice([True, False]):
raise ValueError("Random failure occurred")
return "Task completed successfully"
# Example usage
# unreliable_task()
In this example, the retry
decorator attempts to execute the unreliable_task
function up to five times, with a two-second delay between attempts. This can significantly improve the reliability of automated tasks that depend on flaky external resources.
Caching Results with Decorators
Caching is another area where decorators can significantly enhance automation. By caching the results of expensive function calls, you can avoid redundant computations, saving time and resources.
from functools import lru_cache
@lru_cache(maxsize=32)
def expensive_computation(x):
print(f"Computing for {x}...")
return x * x
# Example usage
# expensive_computation(4)
# expensive_computation(4) # This call will return the cached result
The lru_cache
decorator from Python's functools
module is a simple way to add caching to your functions. In the example above, the result of expensive_computation
is cached, so subsequent calls with the same argument are nearly instantaneous.
Conclusion
Decorators are a versatile and powerful feature of Python that can greatly enhance the automation of everyday tasks. By abstracting common patterns like logging, access control, retry mechanisms, and caching into decorators, you can write cleaner, more maintainable code. As you continue to automate tasks with Python, consider how decorators can help you build more robust and flexible solutions.
Whether you're building a simple script or a complex automated system, understanding and utilizing decorators will undoubtedly enhance your ability to create efficient and effective Python applications.
Now answer the exercise about the content:
What is the primary role of decorators in Python programming as described in the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
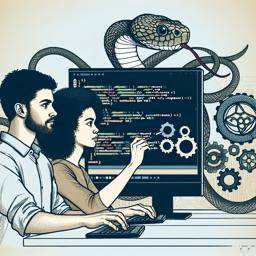
Next page of the Free Ebook: