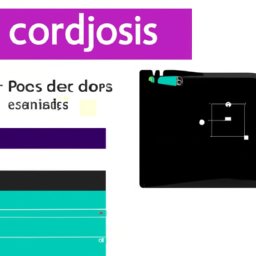
12. Working with CORS in NodeJS
Página 80
Chapter 12: Working with CORS in NodeJS
CORS, or Cross-Origin Resource Sharing, is a crucial technology for modern web developers. It's a way to allow a website to access resources from another website, even if they are on different domains. In simple terms, it is a policy that determines whether a browser should allow a script running on a page to access resources on another domain.
When working with APIs in NodeJS, it is common to encounter CORS issues. This is because, by default, the browser's Same-Origin Policy prevents sites from accessing resources on other sites. This policy is an important security measure, but it can be an obstacle when developing APIs that must be accessible from different domains.
To work around this problem, we can use the NodeJS CORS module. This module adds the necessary CORS headers to server responses, allowing browsers to access the API from different domains.
To start working with CORS in NodeJS, you first need to install the module. This can easily be done with npm, the NodeJS package manager. Just open the terminal and type the following command:
npm install cors
Once installed, you can start using the module in your code. First, you need to import it into your file. This is done with the command require:
const cors = require('cors')
Next, you need to use CORS middleware in your application. This is done with the use method of the Express application object:
app.use(cors())
With this, your NodeJS server is configured to accept requests from any domain. However, in a production environment you will probably want to restrict access to certain domains. This can be done by passing an options object to the function cors:
app.use(cors({
origin: 'http://mysite.com'
}))
This configuration will only allow the domain 'http://mysite.com' to access your API.
In addition to the home domain, there are many other options you can configure. For example, you can specify which HTTP methods are allowed with the methods option:
app.use(cors({
origin: 'http://mysite.com',
methods: ['GET', 'POST']
}))
This configuration will allow the domain 'http://mysite.com' to make GET and POST requests to your API, but not PUT or DELETE, for example.
Another useful option is credentials. If set to true, this will allow browsers to include cookies in requests to your API. This is useful if you are using sessions or token-based authentication:
app.use(cors({
origin: 'http://mysite.com',
methods: ['GET', 'POST'],
credentials: true
}))
Working with CORS in NodeJS is essential to create secure and accessible APIs. While it might seem complicated at first, the CORS module makes the process quite simple. With just a few lines of code, you can configure your API to accept requests from different domains, while still maintaining control over which domains have access and which HTTP methods they can use.
In short, CORS is a crucial part of web security and an important topic for any NodeJS developer to understand. By mastering CORS, you'll be one step closer to building robust and secure APIs in NodeJS.
Now answer the exercise about the content:
What is CORS and how is it used in NodeJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
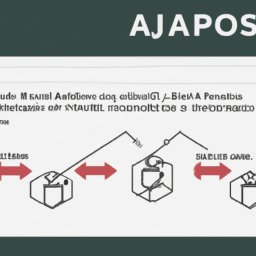
Next page of the Free Ebook: