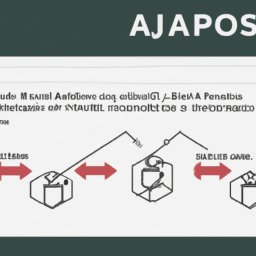
13. Error handling in NodeJS
Page 81 | Listen in audio
Handling errors in NodeJS is an essential skill for any developer. Errors can occur in any application, whether it's due to a problem in the code, a problem with the input data, or a problem with the runtime environment. Therefore, it is crucial that your application can handle errors gracefully and informatively.
In NodeJS, errors are represented by error objects. An error object is created using the keyword 'new' followed by the name 'Error'. For example: 'new Error('This is an error')'. This error object can then be thrown using the 'throw' keyword. For example: 'throw new Error('This is an error')'.
Once an error has been thrown, it can be caught and handled using a 'try/catch' block. The 'try' block contains code that can throw an error, while the 'catch' block contains code that handles the error. For example:
try { throw new Error('This is an error'); } catch (err) { console.error(err.message); }
The above code will print 'This is an error' to the console. Note that the error object is passed to the 'catch' block as 'err' and that the error message can be accessed as 'err.message'.
In addition to the error message, the error object also contains a 'stack' property that provides a stack trace of the error. The stack trace is a list of functions that were called, in order, up to the point where the error was thrown. This can be extremely useful for debugging errors.
In NodeJS, there are several built-in error classes that you can use to represent different types of errors. For example, 'RangeError' can be used to represent an error that occurs when a value is outside the range of acceptable values, and 'TypeError' can be used to represent an error that occurs when a value is not of the expected type. Each of these error classes has its own default error message, but you can also provide your own error message.
In addition to using the built-in error classes, you can also create your own custom error classes. This can be useful if you want to represent errors specific to your application. To create a custom error class, you can extend the 'Error' class. For example:
class CustomError extends Error { constructor(message) { super(message); this.name = 'CustomError'; } }
You can then throw and catch 'CustomError' just as you would any other error.
Another important thing to note about error handling in NodeJS is that uncaught errors will result in the termination of the NodeJS process. This means that if an error is thrown and not caught by a 'try/catch' block, your application will fail. To avoid this, you can add an 'uncaughtException' event handler to the 'process' object. This event handler will be called whenever an uncaught error is thrown. For example:
process.on('uncaughtException', (err) => { console.error('Uncaught error:', err); });
This will print the error message and stack trace to the console, but will not prevent the process from terminating. If you want your application to continue running even after an uncaught error, you can add 'process.exit(1)' to the end of the event handler. However, this must be done with care as it may result in an inconsistent application state.
In summary, error handling in NodeJS is done by throwing and catching error objects. NodeJS provides several built-in error classes that you can use, but you can also create your own custom error classes. Uncaught errors will result in termination of the NodeJS process unless an 'uncaughtException' event handler is added to the 'process' object.
Now answer the exercise about the content:
How is error handling done in NodeJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
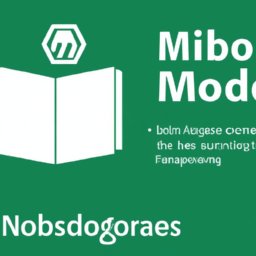
Next page of the Free Ebook: