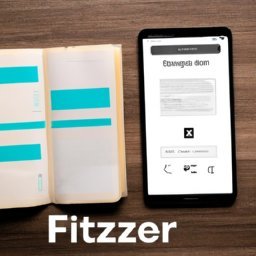
6.5. Widgets in Flutter: Navigating between screens
Page 77 | Listen in audio
Widgets are key components when building apps in Flutter. They are the basic building blocks of the UI in Flutter and each widget is an immutable part of the UI. In this context, an important aspect of widgets in Flutter is navigation between screens. Navigating between screens is a crucial aspect of creating mobile apps. In Flutter, navigation between screens is handled by something called a Navigator, which is a widget that manages a stack of Route objects.
The Navigator works similarly to how web site navigation works. When a user opens an app, they start at the "home page" and from there can navigate to other "pages" or screens. Each time a new screen is opened, it is stacked on top of the stack. When the user finishes interacting with a screen and closes it, that screen is popped from the stack and the previous screen becomes the active one again.
To navigate between screens in Flutter, you need to use method 'Navigator.push' to open a new screen, and 'Navigator.pop' to close the current screen and go back to the previous one. Here is an example of how you can do this:
Navigator.push( context, MaterialPageRoute(builder: (context) => SecondScreen()), );
And here's how you would close the current screen and go back to the previous one:
Navigator.pop(context);
Also, you can pass arguments between screens during navigation. For example, if you want to pass a value from one screen to another, you can do it like this:
Navigator.push( context, MaterialPageRoute( builder: (context) => SecondScreen(value: 'Hello from FirstScreen!'), ), );
And in SecondScreen, you can access the passed value like this:
class SecondScreen extends StatelessWidget { final String value; SecondScreen({Key key, @required this.value}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Second Screen'), ), body: Center( child: Text('Passed value: $value'), ), ); } }
This is just the basics of navigating between screens in Flutter. There are many other advanced features that you can use such as named navigation, sending data back to the previous screen, navigation with custom animations and much more. But to begin with, understanding how to use the Navigator to push and bust routes is a crucial first step.
To summarize, widgets in Flutter are the foundation for building the user interface. They are immutable and every time you want to change the appearance of a widget you need to create a new one. Navigation between screens is handled by a widget called Navigator, which stacks screens as they are opened and removes them from the stack when they are closed. You can use the 'push' method to open a new screen and 'pop' to close the current one. Also, you can pass arguments between screens during navigation, which can be useful for passing data from one screen to another.
I hope this text has helped you better understand widgets in Flutter and how to navigate between screens. Remember, practice makes perfect, so keep practicing and experimenting with different widgets and navigation methods to become more comfortable with Flutter.
Now answer the exercise about the content:
In the context of app development in Flutter, what is the function of the Navigator widget?
You are right! Congratulations, now go to the next page
You missed! Try again.
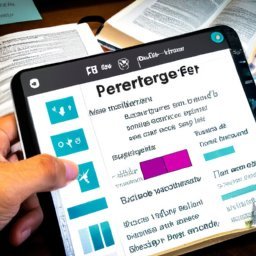
Next page of the Free Ebook: