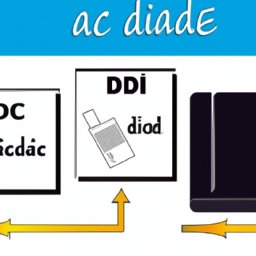
Variables and data types
Page 25 | Listen in audio
Variables are fundamental elements in any programming language. They are used to store values that will be used in calculations, comparisons and other operations. In programming languages, variables are defined by the programmer and can receive different types of data.
The most common data types in programming languages are:
- Integer: used to store whole numbers, such as 1, 2, 3, -4, -5, etc. In some programming languages, it is possible to define the size of the integer, such as 8 bits, 16 bits, 32 bits, etc.
- Floating point: used to store numbers with decimal places, such as 3.14, 2.5, -0.75, etc. In some programming languages, it is possible to set the floating point size, such as 32 bits, 64 bits, etc.
- Boolean: used to store true or false values. Usually represented by true (true) or false (false).
- Character: used to store a single character, such as 'a', 'b', 'c', '1', '2', '3', etc. In some programming languages, it is possible to set the character size, such as 8 bits, 16 bits, etc.
- String: used to store a sequence of characters, such as "Hello world!", "Basic computer course", etc.
To declare a variable in a programming language, it is necessary to define the name of the variable and the type of data it will store. For example, in C language, to declare an integer variable called "age", it is necessary to write:
int age;
In Python language, to declare a string variable called "name", it is necessary to write:
name = "John";
Once declared, the variable can receive a value. For example, to assign the value 25 to the variable "age" in C language, it is necessary to write:
age = 25;
In Python language, to assign the value "Maria" to the variable "name", it is necessary to write:
name = "Mary";
Variables can be used in calculations and other operations. For example, in C language, to add two integers and store the result in a variable called "sum", it is necessary to write:
int a = 10;
int b = 20;
int sum = a + b;
In Python language, to add two integers and store the result in a variable called "sum", it is necessary to write:
a = 10
b = 20
sum = a + b
Variables can also be used in control structures such as conditionals and loops. For example, in C language, to check if the variable "age" is greater than or equal to 18 years, it is necessary to write:
if (age >= 18) {
printf("You are of legal age.");
} else {
printf("You are a minor.");
}
In Python language, to check if the variable "age" is greater than or equal to 18 years, it is necessary to write:
if age >= 18:
print("You are of legal age.")
else:
print("You are a minor.")
In summary, variables are fundamental elements in any programming language. They are used to store values that will be used in calculations, comparisons and other operations. It is important to define the data type of the variable according to the value it will store. Variables can be used in control structures such as conditionals and loops to perform more complex operations.
Now answer the exercise about the content:
_What is the data type used to store true or false values in programming languages?
You are right! Congratulations, now go to the next page
You missed! Try again.
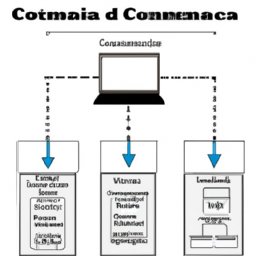
Next page of the Free Ebook: