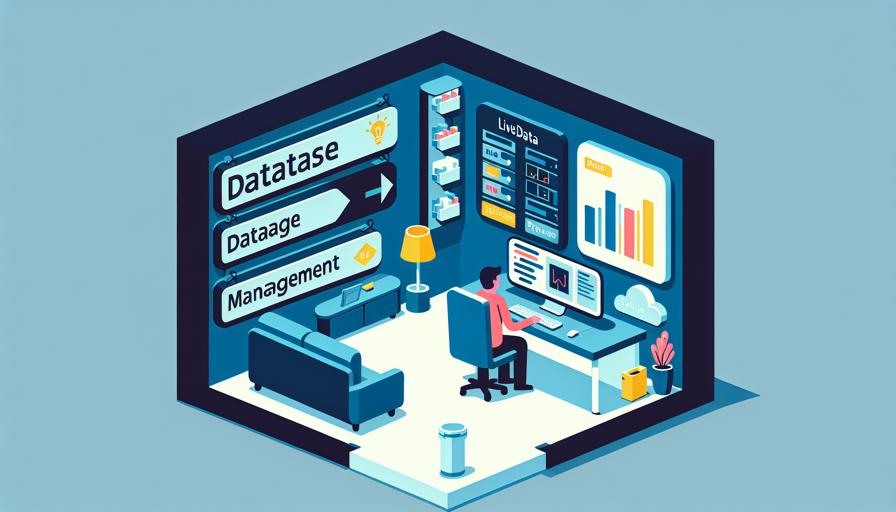
59.7. Using Room for Database Management: Using Room with LiveData
Page 84 | Listen in audio
In the realm of Android app development, managing data efficiently and effectively is crucial. One of the key components that facilitate this is the Room Persistence Library, which provides an abstraction layer over SQLite to allow for more robust database management. When combined with LiveData, Room becomes a powerful tool for building responsive and dynamic applications. This section delves into the intricacies of using Room for database management, specifically focusing on its integration with LiveData.
Room is part of the Android Jetpack suite and offers a more convenient and efficient way to handle databases compared to raw SQLite. It simplifies database operations by providing compile-time checks of SQL queries and reducing boilerplate code. The integration of Room with LiveData further enhances its capabilities by allowing developers to create applications that can respond to changes in the database in real-time.
Understanding Room
Room is structured around three major components:
- Entity: Represents a table within the database. Each entity is a class annotated with
@Entity
, and each field represents a column in the table. - DAO (Data Access Object): This is an interface or abstract class annotated with
@Dao
. It contains methods that offer abstract access to the database, such as querying data, inserting, updating, and deleting records. - Database: This is an abstract class annotated with
@Database
, extendingRoomDatabase
. It serves as the main access point for the underlying connection to the app's persisted data.
By defining these components, Room provides a structured way to interact with the database, ensuring that data operations are both type-safe and efficient.
Integrating LiveData
LiveData is an observable data holder class that is lifecycle-aware. This means it respects the lifecycle of other app components, such as activities, fragments, or services, ensuring that LiveData only updates observers that are in an active lifecycle state. This is particularly useful in Android development, where managing UI updates in response to data changes can be complex.
When LiveData is combined with Room, it allows for automatic updates to the UI when the data in the database changes. This eliminates the need for manual data refreshing and ensures that the user interface is always in sync with the underlying data.
Setting Up Room with LiveData
To integrate Room with LiveData, follow these steps:
- Define Your Entity: Start by defining a data class for your entity. This class will represent a table in your database. Use annotations to specify the table name and primary key.
- Create the DAO: Define an interface annotated with
@Dao
. Within this interface, define methods for accessing the database, such as queries and insertions. UseLiveData
as the return type for query methods to observe data changes. - Define the Database: Create an abstract class that extends
RoomDatabase
and annotate it with@Database
. Include the entities and DAOs in this class. - Observe LiveData in the UI: In your activity or fragment, observe the LiveData returned by the DAO. This will automatically update the UI when the database changes.
@Entity(tableName = "user_table")
data class User(
@PrimaryKey(autoGenerate = true) val id: Int,
val name: String,
val age: Int
)
@Dao
interface UserDao {
@Query("SELECT * FROM user_table")
fun getAllUsers(): LiveData>
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insert(user: User)
@Delete
suspend fun delete(user: User)
}
@Database(entities = [User::class], version = 1, exportSchema = false)
abstract class UserDatabase : RoomDatabase() {
abstract fun userDao(): UserDao
companion object {
@Volatile
private var INSTANCE: UserDatabase? = null
fun getDatabase(context: Context): UserDatabase {
return INSTANCE ?: synchronized(this) {
val instance = Room.databaseBuilder(
context.applicationContext,
UserDatabase::class.java,
"user_database"
).build()
INSTANCE = instance
instance
}
}
}
}
class UserActivity : AppCompatActivity() {
private lateinit var userViewModel: UserViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_user)
userViewModel = ViewModelProvider(this).get(UserViewModel::class.java)
userViewModel.allUsers.observe(this, Observer { users ->
// Update the UI
users?.let { updateUI(it) }
})
}
}
Benefits of Using Room with LiveData
The combination of Room and LiveData offers several advantages:
- Automatic UI Updates: By observing LiveData, the UI is automatically updated whenever the data in the database changes. This reduces the need for manual refreshes and ensures data consistency.
- Lifecycle Awareness: LiveData respects the lifecycle of app components, ensuring that updates are only sent to active observers. This prevents memory leaks and unnecessary updates to inactive components.
- Type Safety: Room provides compile-time verification of SQL queries, reducing runtime errors and improving code reliability.
- Reduced Boilerplate Code: The use of annotations in Room significantly reduces the amount of boilerplate code needed for database operations, making the codebase cleaner and more maintainable.
Considerations and Best Practices
While Room and LiveData offer powerful features, there are some considerations and best practices to keep in mind:
- Asynchronous Operations: Database operations can be time-consuming, so it's important to perform them asynchronously. Room supports this by allowing DAO methods to return
LiveData
orFlow
, and by using Kotlin coroutines for insert, update, and delete operations. - Handling Large Datasets: When dealing with large datasets, consider using
PagedList
withLiveData
to efficiently load and display data in chunks. - Testing: Room provides support for testing database operations. Use in-memory databases for unit tests to ensure that your database logic is correct without affecting the actual database.
- Migration Strategy: As your app evolves, you may need to change the database schema. Plan a migration strategy to handle schema changes gracefully without losing user data.
In conclusion, using Room with LiveData in Android app development provides a robust and efficient way to manage databases. It streamlines data operations, ensures UI consistency, and enhances app responsiveness. By following best practices and leveraging the strengths of both Room and LiveData, developers can create applications that are both performant and maintainable.
Now answer the exercise about the content:
You are right! Congratulations, now go to the next page
You missed! Try again.
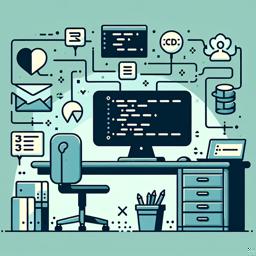
Next page of the Free Ebook: