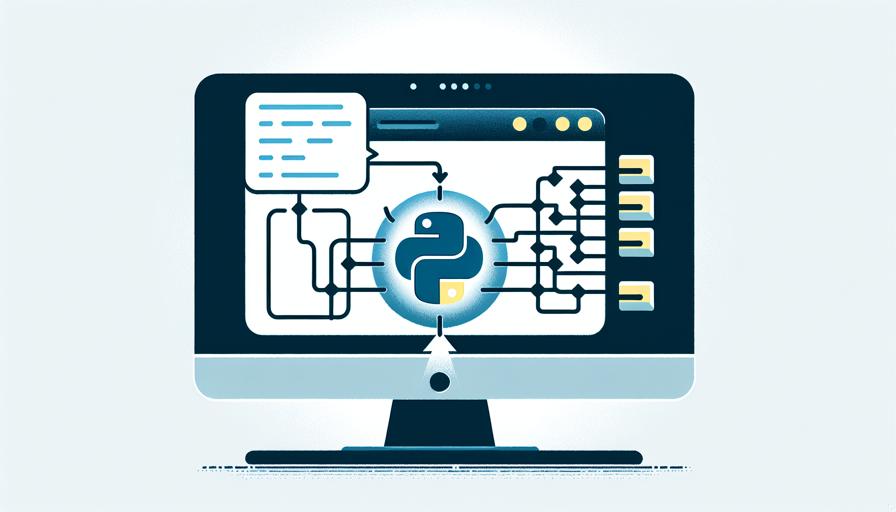
14. Using Requests to Fetch Web Data
Page 34 | Listen in audio
In the digital age, the internet is a treasure trove of information, and being able to programmatically access this data opens up a world of possibilities for automation and data analysis. One of the most powerful tools in Python for fetching web data is the requests
library. In this chapter, we will delve into how you can use the requests
library to automate the retrieval of web data, enabling you to harness the power of the internet in your Python programs.
Understanding HTTP and the Role of Requests
Before diving into the requests
library, it's important to understand the basics of HTTP, the protocol underlying the web. HTTP (Hypertext Transfer Protocol) is the protocol used by the World Wide Web for transmitting data. It is a request-response protocol where a client (often a web browser) makes a request to a server, which in turn sends back a response.
HTTP methods define the action to be performed on the resource. The most common HTTP methods are:
- GET: Retrieve data from a server.
- POST: Send data to a server to create/update a resource.
- PUT: Update a resource on the server.
- DELETE: Remove a resource from the server.
The requests
library in Python is a simple and elegant HTTP library for making HTTP requests. It abstracts the complexities of making requests behind a beautiful, simple API, allowing you to send HTTP requests with ease.
Installing the Requests Library
Before you can use the requests
library, you need to install it. You can install it using pip, Python's package manager, with the following command:
pip install requests
Once installed, you can start using the library in your Python scripts to fetch web data.
Making Your First Request
Let's start by making a simple GET request to fetch data from a web page. The following example demonstrates how to use the requests
library to retrieve the HTML content of a web page:
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
print('Success!')
print(response.text)
else:
print('Failed to retrieve the page')
In this example, we use the requests.get()
function to send a GET request to the specified URL. The response from the server is stored in the response
object. We then check the status code of the response to determine if the request was successful. A status code of 200 indicates success, and we print the HTML content of the page using response.text
.
Handling Different Response Codes
HTTP response codes are crucial for understanding the result of your requests. Here are some common response codes you might encounter:
- 200 OK: The request was successful.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: The server encountered an error.
- 403 Forbidden: You do not have permission to access the resource.
Handling different response codes is essential for building robust applications. You can use conditional statements to handle these codes appropriately.
Passing Parameters in URLs
When making GET requests, you often need to pass parameters in the URL. The requests
library makes this easy with the params
parameter. Here's an example:
url = 'https://api.example.com/data'
params = {'key1': 'value1', 'key2': 'value2'}
response = requests.get(url, params=params)
print(response.url)
In this example, the params
dictionary is passed to the requests.get()
function, which automatically appends the parameters to the URL. The final URL can be printed using response.url
.
Sending POST Requests
In addition to GET requests, you can also send POST requests using the requests
library. POST requests are used to send data to a server, often to submit forms or upload files. Here's an example:
url = 'https://api.example.com/submit'
data = {'username': 'user', 'password': 'pass'}
response = requests.post(url, data=data)
print(response.status_code)
In this example, we send a POST request to the specified URL with the data provided in the data
dictionary. The server's response status code is then printed.
Handling JSON Data
Many web APIs return data in JSON format, a lightweight data interchange format. The requests
library provides convenient methods for handling JSON data. Here's how you can work with JSON responses:
url = 'https://api.example.com/data'
response = requests.get(url)
if response.status_code == 200:
json_data = response.json()
print(json_data)
else:
print('Failed to retrieve JSON data')
In this example, we use the response.json()
method to parse the JSON data returned by the server. This method automatically decodes the JSON data into a Python dictionary.
Setting Headers and Cookies
Sometimes, you may need to set custom headers or cookies in your requests. This can be done using the headers
and cookies
parameters. Here's an example:
url = 'https://api.example.com/data'
headers = {'User-Agent': 'my-app'}
cookies = {'session_id': '12345'}
response = requests.get(url, headers=headers, cookies=cookies)
print(response.text)
In this example, we set a custom User-Agent header and a session cookie in the request. This can be useful for simulating requests from a specific browser or maintaining session state.
Error Handling and Exceptions
While using the requests
library, you may encounter various exceptions, such as connection errors or timeouts. It's essential to handle these exceptions to ensure your program doesn't crash unexpectedly. Here's an example of how to handle exceptions:
try:
response = requests.get('https://api.example.com/data', timeout=5)
response.raise_for_status()
except requests.exceptions.HTTPError as errh:
print('HTTP Error:', errh)
except requests.exceptions.ConnectionError as errc:
print('Error Connecting:', errc)
except requests.exceptions.Timeout as errt:
print('Timeout Error:', errt)
except requests.exceptions.RequestException as err:
print('OOps: Something Else', err)
In this example, we use a try-except
block to catch various exceptions that may occur during the request. The response.raise_for_status()
method raises an HTTPError for bad responses (4xx and 5xx status codes), allowing us to handle them appropriately.
Conclusion
The requests
library is a powerful tool for fetching web data in Python. With its simple and intuitive API, you can easily send HTTP requests, handle responses, and interact with web services. By automating the process of retrieving web data, you can unlock new possibilities for data analysis, web scraping, and building intelligent applications.
As you continue to explore the capabilities of the requests
library, remember to handle exceptions and response codes appropriately to build robust and reliable applications. With practice, you'll become proficient in automating everyday tasks with Python, making your work more efficient and effective.
Now answer the exercise about the content:
Which Python library is highlighted as a powerful tool for fetching web data and automating the retrieval of web data in the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
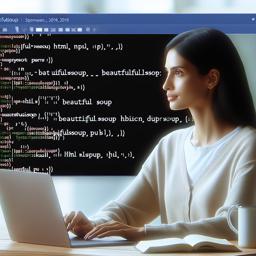
Next page of the Free Ebook: