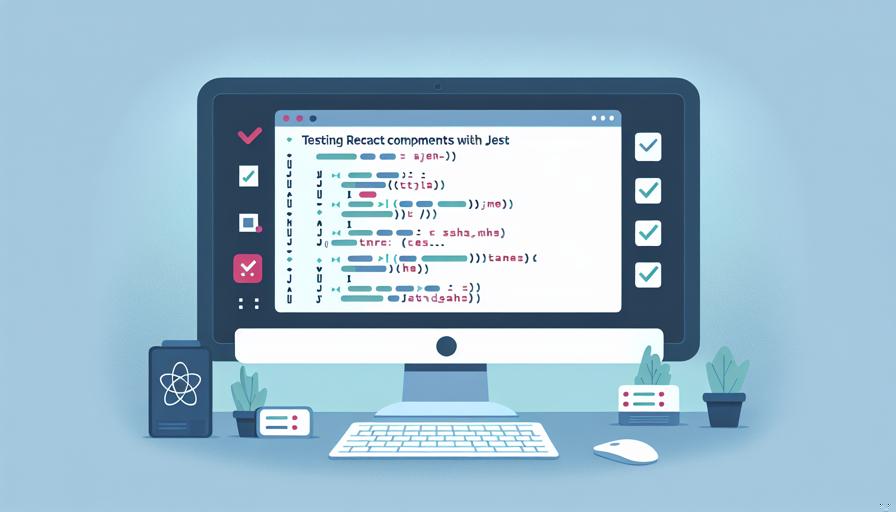
33. Testing React Components with Jest
Page 77 | Listen in audio
Testing is a crucial part of the software development lifecycle, ensuring that your application behaves as expected and remains robust over time. In the context of React applications, testing components effectively can prevent bugs and improve the quality of your codebase. Jest, a delightful JavaScript testing framework developed by Facebook, is widely used for testing React applications due to its ease of use, powerful features, and seamless integration with React. This section will guide you through the process of testing React components using Jest, covering various aspects and best practices.
Before diving into testing, it's essential to understand the types of tests you can perform on your React components. Generally, there are three main types of testing: unit testing, integration testing, and end-to-end (E2E) testing. Unit testing focuses on testing individual components or functions in isolation. Integration testing evaluates how different modules or components work together. E2E testing simulates real user interactions to ensure the entire application works as expected. In this section, we will primarily focus on unit testing React components with Jest.
To get started with Jest, you'll first need to set it up in your React project. If you created your project using Create React App, Jest is already included. Otherwise, you can install it by running:
npm install --save-dev jest
Once Jest is installed, you can create a test file for your React component. It's a common convention to place test files alongside the components they test, naming them with a .test.js
or .spec.js
extension. For instance, if you have a Button.js
component, you might create a Button.test.js
file in the same directory.
Jest provides a global test
function that you can use to define your tests. A basic test case looks like this:
import { render } from '@testing-library/react';
import Button from './Button';
test('renders the button with correct text', () => {
const { getByText } = render(<Button>Click me</Button>);
const buttonElement = getByText(/click me/i);
expect(buttonElement).toBeInTheDocument();
});
In this example, we're using the render
function from the @testing-library/react
package to render the Button
component. This library is a popular choice for testing React components because it encourages testing components in a way that resembles how users interact with them. The getByText
function is used to query the DOM for an element with the specified text content. Finally, we use Jest's expect
function to assert that the button element is present in the document.
Jest also supports snapshot testing, a feature that allows you to capture the rendered output of a component and compare it to a reference snapshot stored alongside your tests. This can be useful for ensuring that your component's output doesn't change unexpectedly. Here's how you can create a snapshot test:
import renderer from 'react-test-renderer';
import Button from './Button';
test('Button component matches snapshot', () => {
const tree = renderer.create(<Button>Click me</Button>).toJSON();
expect(tree).toMatchSnapshot();
});
In this example, we're using the react-test-renderer
package to render the component and generate a JSON representation of its output. The toMatchSnapshot
matcher compares the generated output to a previously saved snapshot, alerting you if there are any differences.
While snapshot testing is powerful, it's essential to use it judiciously. Over-reliance on snapshots can lead to brittle tests that are difficult to maintain. Instead, focus on writing meaningful tests that verify the behavior and logic of your components.
Jest also provides a rich set of matchers and utilities to help you write more comprehensive tests. For instance, you can use the fireEvent
utility from @testing-library/react
to simulate user interactions:
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
test('calls onClick handler when clicked', () => {
const handleClick = jest.fn();
const { getByText } = render(<Button onClick={handleClick}>Click me</Button>);
const buttonElement = getByText(/click me/i);
fireEvent.click(buttonElement);
expect(handleClick).toHaveBeenCalledTimes(1);
});
In this example, we're testing that the onClick
handler is called when the button is clicked. We use jest.fn()
to create a mock function, which allows us to track how many times it's been called. The fireEvent.click
function simulates a click event on the button, and we use expect
to assert that the mock function was called once.
Another valuable feature of Jest is its ability to mock modules and functions. This can be particularly useful when testing components that rely on external dependencies or asynchronous operations. Jest provides a jest.mock
function to replace a module with a mock implementation:
jest.mock('./api', () => ({
fetchData: jest.fn(() => Promise.resolve({ data: 'mock data' })),
}));
import { render, waitFor } from '@testing-library/react';
import App from './App';
import { fetchData } from './api';
test('fetches and displays data', async () => {
const { getByText } = render(<App />);
await waitFor(() => expect(fetchData).toHaveBeenCalled());
expect(getByText(/mock data/i)).toBeInTheDocument();
});
In this example, we're mocking the fetchData
function from an api
module to return a resolved promise with mock data. This allows us to test the App
component's behavior without making actual network requests. We use the waitFor
function to wait for asynchronous operations to complete before making assertions.
Testing React components with Jest is a powerful way to ensure your application remains reliable and maintainable. By writing tests that verify both the structure and behavior of your components, you can catch bugs early and refactor code with confidence. As you continue to build and expand your React applications, incorporating Jest into your development workflow will undoubtedly contribute to higher quality software and a smoother development process.
In conclusion, mastering Jest for testing React components involves understanding the different types of tests, setting up your testing environment, and utilizing Jest's features effectively. By following best practices and writing meaningful tests, you can significantly enhance the stability and reliability of your React applications. As you gain more experience with Jest, you'll find that it becomes an indispensable tool in your development toolkit, helping you deliver robust and high-quality React applications.
Now answer the exercise about the content:
What is the primary focus of this section when testing React components with Jest?
You are right! Congratulations, now go to the next page
You missed! Try again.
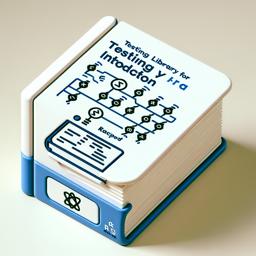
Next page of the Free Ebook: