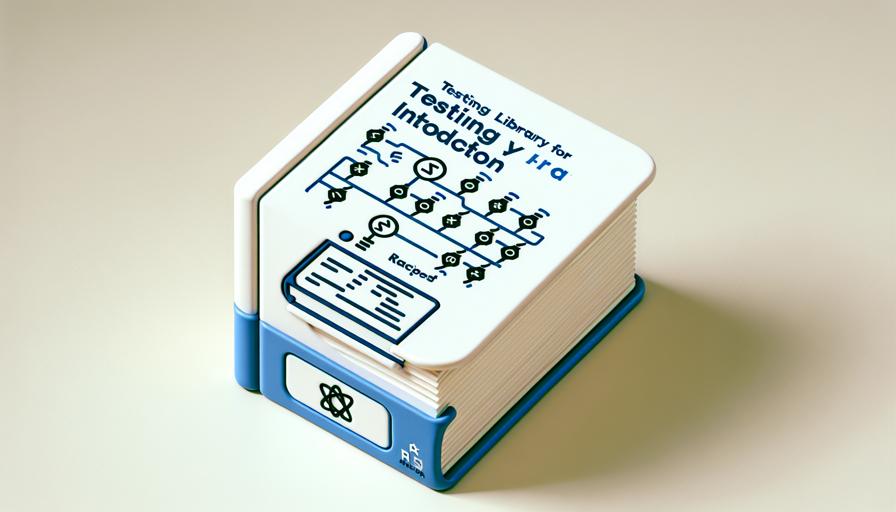
34. Testing Library for React: An Introduction
Page 78 | Listen in audio
When developing React applications, ensuring that your components work as expected is crucial. Testing is a key part of the development process, and the React Testing Library has become a popular choice for testing React components. It helps developers write tests that focus on how users interact with components, rather than the implementation details. In this section, we’ll dive into the React Testing Library, exploring its features, benefits, and how to integrate it into your React projects.
Understanding the Basics
The React Testing Library is a lightweight testing solution that encourages best practices by focusing on testing the behavior of your components from a user’s perspective. It’s built on top of the DOM Testing Library, which provides utilities to interact with the DOM in a way that resembles how users would interact with your app.
One of the core principles of the React Testing Library is to test the component’s public API. This means interacting with the component as a user would, through the DOM, rather than testing its internal state or methods. This approach results in tests that are more resilient to changes in the implementation and more aligned with the actual use cases of the application.
Setting Up React Testing Library
To get started with the React Testing Library, you need to install it along with Jest, which is a popular testing framework for JavaScript. You can install them using npm or yarn:
npm install --save-dev @testing-library/react @testing-library/jest-dom
or
yarn add --dev @testing-library/react @testing-library/jest-dom
Once installed, you can start writing tests for your React components. It’s recommended to create a __tests__
directory in your project where you can place all your test files. By convention, test files are named with a .test.js
or .spec.js
suffix.
Writing Your First Test
Let’s walk through writing a simple test for a React component using the React Testing Library. Consider a basic Button
component that renders a button element with a label:
import React from 'react';
function Button({ label, onClick }) {
return <button onClick={onClick}>{label}</button>;
}
export default Button;
Now, let’s write a test to ensure that this button renders correctly and responds to click events:
import React from 'react';
import { render, screen, fireEvent } from '@testing-library/react';
import '@testing-library/jest-dom/extend-expect';
import Button from './Button';
test('renders a button with the provided label', () => {
render(<Button label="Click Me" />);
const buttonElement = screen.getByText(/click me/i);
expect(buttonElement).toBeInTheDocument();
});
test('calls onClick handler when clicked', () => {
const handleClick = jest.fn();
render(<Button label="Click Me" onClick={handleClick} />);
const buttonElement = screen.getByText(/click me/i);
fireEvent.click(buttonElement);
expect(handleClick).toHaveBeenCalledTimes(1);
});
In the first test, we use the render
function to render the Button
component. The screen.getByText
method is used to query the DOM for an element with the text "Click Me". We then assert that this element is in the document using expect
and toBeInTheDocument
.
The second test checks whether the onClick
handler is called when the button is clicked. We use Jest’s jest.fn()
to create a mock function and pass it as the onClick
prop. After simulating a click event with fireEvent.click
, we assert that the mock function was called exactly once.
Querying the DOM
The React Testing Library provides several methods for querying the DOM, each with a specific use case. Here are some of the most commonly used query methods:
getByText
: Finds an element by its text content.getByRole
: Finds an element by its ARIA role.getByLabelText
: Finds a form element by its associated label.getByPlaceholderText
: Finds an input element by its placeholder text.getByTestId
: Finds an element by a custom data-testid attribute.
Each of these methods is designed to mimic how users would interact with the application, ensuring that your tests are both meaningful and robust. The library also provides queryBy*
and findBy*
variants for non-existent elements and asynchronous queries, respectively.
Best Practices
To make the most of the React Testing Library, consider the following best practices:
- Test User Interactions: Focus on testing how users interact with your components rather than their internal implementation. This leads to tests that are more maintainable and aligned with the user experience.
- Avoid Testing Implementation Details: Avoid testing internal state or private methods of your components. This can lead to brittle tests that break with refactoring, even if the component’s behavior remains unchanged.
- Use Screen for Queries: Use the
screen
object for querying elements. It helps make your tests more readable and aligns with the recommended testing patterns. - Leverage Jest’s Matchers: Use Jest’s built-in matchers, such as
toBeInTheDocument
,toHaveTextContent
, andtoHaveAttribute
, to write clear and expressive assertions. - Structure Tests Clearly: Organize your tests using
describe
blocks and separate logical parts of your tests usingbeforeEach
andafterEach
hooks when needed.
Advanced Topics
As you become more comfortable with the React Testing Library, you may encounter scenarios that require more advanced techniques. Here are a few topics to explore:
Mocking API Calls
When testing components that make API calls, it’s often necessary to mock these calls to avoid hitting the actual endpoints. You can use libraries like msw
(Mock Service Worker) to intercept network requests and provide mock responses, ensuring that your tests are fast and reliable.
Testing Asynchronous Code
React components often deal with asynchronous operations, such as fetching data or waiting for animations to complete. The React Testing Library provides utilities like waitFor
and findBy*
queries to handle asynchronous behavior in your tests.
Custom Render Functions
Sometimes, you may need to wrap your components with context providers or other higher-order components for testing. You can create custom render functions that include these wrappers, making it easier to test components in different contexts.
Snapshot Testing
Snapshot testing is a technique used to capture the rendered output of a component and compare it to a saved snapshot. This can be useful for catching unexpected changes in the UI. The React Testing Library can be used in conjunction with Jest’s snapshot testing capabilities to achieve this.
Conclusion
The React Testing Library is a powerful tool that encourages best practices by focusing on testing components from a user’s perspective. By writing tests that mimic real user interactions, you can create more reliable and maintainable test suites for your React applications. As you continue to develop your testing skills, you’ll find that the React Testing Library provides a solid foundation for ensuring the quality and stability of your code.
Remember that testing is an integral part of the development process, and investing time in writing meaningful tests will pay off in the long run. Whether you’re just starting with React or looking to improve your testing practices, the React Testing Library is a valuable addition to your toolkit.
Now answer the exercise about the content:
You are right! Congratulations, now go to the next page
You missed! Try again.
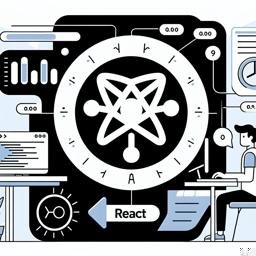
Next page of the Free Ebook: