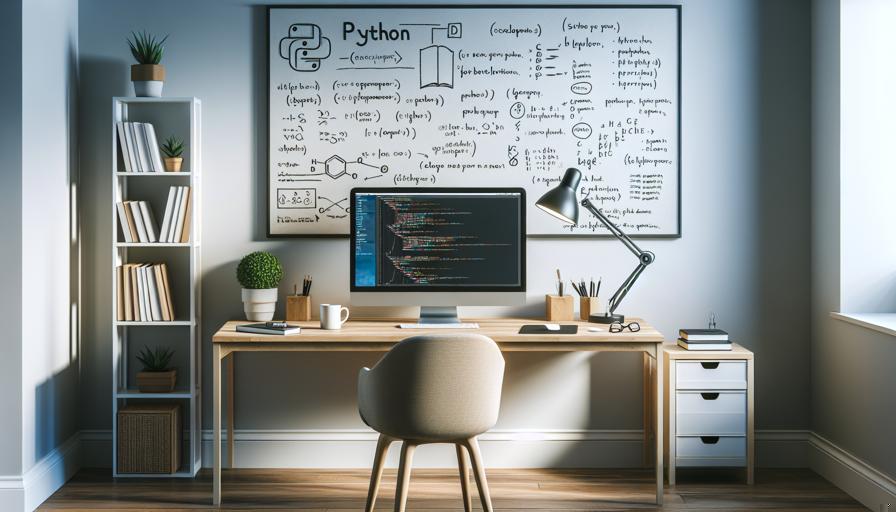
2. Setting Up a Python Development Environment
Page 2 | Listen in audio
Setting Up a Python Development Environment
As you embark on your journey to automate everyday tasks with Python, setting up a robust development environment is a critical first step. A well-configured environment not only streamlines your coding process but also enhances productivity and minimizes errors. In this chapter, we'll explore the essential components and steps involved in setting up a Python development environment that suits your needs.
1. Installing Python
The first step in setting up your development environment is to install Python. Python is a versatile language, and the installation process is straightforward. Here’s how you can get started:
Windows
- Visit the official Python website at python.org/downloads.
- Download the latest version of Python for Windows.
- Run the installer and ensure you check the box that says "Add Python to PATH" before clicking "Install Now". This step is crucial as it allows you to run Python from the command line.
- Once installed, open Command Prompt and type
python --version
to verify the installation.
macOS
- Open Terminal.
- Use Homebrew to install Python by executing the command:
brew install python
. If you don’t have Homebrew installed, you can find instructions on their official website. - Verify the installation by typing
python3 --version
in Terminal.
Linux
- Open Terminal.
- Use the package manager for your distribution. For example, on Ubuntu, type:
sudo apt update
followed bysudo apt install python3
. - Verify the installation with
python3 --version
.
2. Choosing a Code Editor
The choice of a code editor can significantly impact your coding experience. Here are some popular options:
Visual Studio Code (VS Code)
VS Code is a free, open-source editor developed by Microsoft. It is highly customizable and supports numerous extensions, making it a favorite among developers. To set up VS Code for Python development:
- Download and install VS Code from code.visualstudio.com.
- Open VS Code and navigate to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window.
- Search for "Python" and install the Python extension by Microsoft, which provides features like IntelliSense, linting, and debugging.
PyCharm
PyCharm is a feature-rich IDE specifically designed for Python development. It comes in two editions: Community (free) and Professional (paid). To set up PyCharm:
- Download PyCharm from jetbrains.com/pycharm.
- Install and launch PyCharm.
- Configure a new Python interpreter by navigating to File > Settings > Project: > Python Interpreter.
Sublime Text
Sublime Text is a lightweight and fast editor with Python support. To enhance its capabilities for Python development:
- Download and install Sublime Text from sublimetext.com.
- Install Package Control, a package manager for Sublime Text, by following the instructions on the official website.
- Use Package Control to install the "Anaconda" package, which provides code completion and linting for Python.
3. Setting Up a Virtual Environment
Virtual environments are essential for managing dependencies and ensuring that your projects have isolated environments. This prevents conflicts between different projects. To set up a virtual environment:
- Open your terminal or command prompt.
- Navigate to your project directory.
- Create a virtual environment by running:
python -m venv env
. Replaceenv
with your preferred name for the virtual environment. - Activate the virtual environment:
- On Windows:
.\env\Scripts\activate
- On macOS and Linux:
source env/bin/activate
- On Windows:
- Once activated, you can install packages using pip, and they will be confined to this environment.
4. Installing Essential Python Packages
Python’s strength lies in its vast ecosystem of libraries and frameworks. Here are some essential packages to get you started with automation:
- pip: The package installer for Python. Use it to install and manage software packages.
- requests: A simple and elegant HTTP library for making requests to web services.
- beautifulsoup4: A library for web scraping purposes to pull data out of HTML and XML files.
- pandas: A powerful data manipulation and analysis library.
- numpy: A fundamental package for scientific computing with Python.
- matplotlib: A plotting library for creating static, interactive, and animated visualizations.
To install these packages, ensure your virtual environment is activated and run:
pip install requests beautifulsoup4 pandas numpy matplotlib
5. Configuring Version Control with Git
Version control is an integral part of software development, allowing you to track changes and collaborate with others. Git is the most widely used version control system. Here's how to set it up:
- Download and install Git from git-scm.com.
- Open your terminal or command prompt and configure your Git username and email:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
- To create a new Git repository, navigate to your project directory and run:
git init
. - To connect your local repository to a remote one, such as GitHub, follow the instructions on the GitHub website to create a new repository and add the remote URL.
6. Setting Up a Debugging Tool
Debugging is a crucial part of the development process. Python has built-in support for debugging with the pdb
module, but using an IDE with integrated debugging tools can be more efficient. For example, in VS Code:
- Open the Debug view by clicking on the Debug icon in the Activity Bar.
- Configure a launch.json file to define how your application should be started and debugged.
- Set breakpoints in your code by clicking in the gutter next to the line numbers.
- Start the debugger by clicking the green play button in the Debug view.
Conclusion
Setting up a Python development environment is a foundational step in your programming journey. By carefully selecting your tools and configuring your environment, you lay the groundwork for efficient and enjoyable coding sessions. Whether you're automating small tasks or developing complex applications, a well-prepared environment will be your steadfast ally, enabling you to focus on writing clean, effective code.
With Python installed, a chosen code editor, virtual environments configured, essential packages ready, version control set up, and debugging tools in place, you're now equipped to tackle any automation project. In the next chapter, we will delve into the basics of Python programming, setting the stage for creating powerful automation scripts.
Now answer the exercise about the content:
What is the purpose of setting up a virtual environment when working with Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
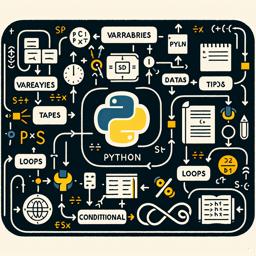
Next page of the Free Ebook: