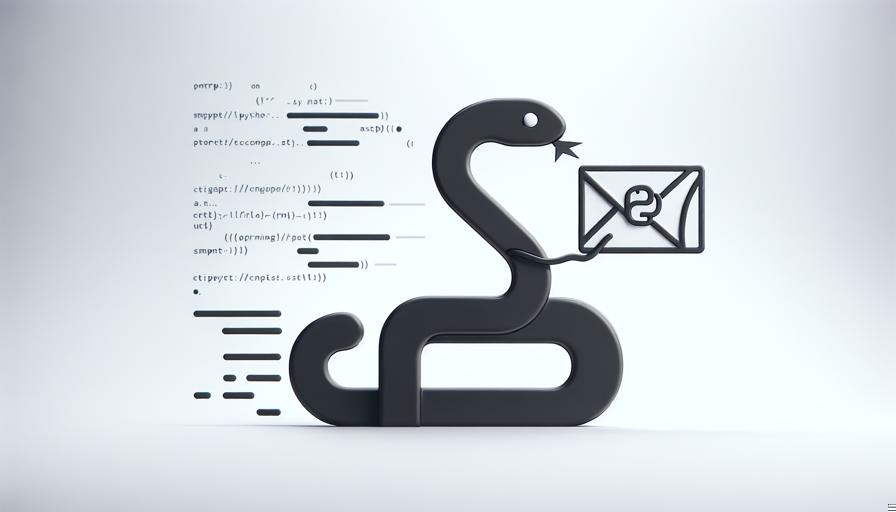
22.1. Sending Automated Emails with Python: Understanding SMTP Protocol
Page 43 | Listen in audio
22.1. Sending Automated Emails with Python: Understanding SMTP Protocol
In the modern world, email remains one of the most reliable and widely used forms of communication. Whether it's for personal correspondence, business communications, or marketing campaigns, the ability to send emails programmatically can save time and enhance efficiency. Python, with its robust libraries and straightforward syntax, offers a powerful toolset for automating email tasks. At the core of this capability is the Simple Mail Transfer Protocol (SMTP), a protocol used to send emails across the Internet.
Understanding SMTP Protocol
SMTP, or Simple Mail Transfer Protocol, is a communication protocol for sending emails between servers. It is a text-based protocol in which one or more recipients of a message are specified, and then the message text is transferred. SMTP operates over the Internet's TCP/IP protocol suite, typically using port 25, although other ports like 587 and 465 are also used for secure transmissions.
The protocol is designed to be simple and efficient, allowing for the reliable transmission of emails. SMTP works by establishing a connection between the sender's and the receiver's mail servers. Once connected, the sender's server sends a series of SMTP commands to the receiver's server to deliver the email. The receiver's server then acknowledges the receipt of the message, ensuring that it has been successfully delivered.
Setting Up Python for Sending Emails
Python provides several libraries to interact with SMTP servers, with smtplib
being the most commonly used. This library allows you to connect to an SMTP server and send emails using Python scripts. Additionally, the email
library is often used in conjunction with smtplib
to construct email messages with attachments, HTML content, and more.
Before diving into sending emails, it's crucial to ensure you have access to an SMTP server. Many email providers, like Gmail, Yahoo, and Outlook, offer SMTP services. You'll need the server address, port number, and login credentials to connect to these services.
Sending a Basic Email with Python
Let's start by sending a simple plain-text email using Python. Below is a basic script that demonstrates this process:
import smtplib
from email.mime.text import MIMEText
def send_email(subject, body, to_email, from_email, smtp_server, smtp_port, username, password):
# Create a MIMEText object to represent the email
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
# Connect to the SMTP server and send the email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls() # Secure the connection
server.login(username, password)
server.sendmail(from_email, to_email, msg.as_string())
# Usage example
send_email(
subject="Test Email",
body="This is a test email sent from Python.",
to_email="[email protected]",
from_email="[email protected]",
smtp_server="smtp.example.com",
smtp_port=587,
username="your_username",
password="your_password"
)
In this script, we use the MIMEText
class from the email.mime.text
module to create a simple text email. The smtplib.SMTP
class is used to connect to the SMTP server and send the email. The starttls()
method is called to secure the connection using TLS encryption.
Sending HTML Emails
Plain-text emails are straightforward, but sometimes you need to send more visually appealing messages. HTML emails allow for rich formatting, including images, links, and styles. Here's how you can send an HTML email with Python:
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
def send_html_email(subject, html_content, to_email, from_email, smtp_server, smtp_port, username, password):
# Create a MIMEMultipart object to represent the email
msg = MIMEMultipart('alternative')
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
# Attach the HTML content to the email
html_part = MIMEText(html_content, 'html')
msg.attach(html_part)
# Connect to the SMTP server and send the email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(username, password)
server.sendmail(from_email, to_email, msg.as_string())
# Usage example
html_content = """
Hello!
This is an HTML email sent from Python.
"""
send_html_email(
subject="HTML Email Test",
html_content=html_content,
to_email="[email protected]",
from_email="[email protected]",
smtp_server="smtp.example.com",
smtp_port=587,
username="your_username",
password="your_password"
)
In this example, we use the MIMEMultipart
class to create an email that can contain both plain-text and HTML parts. We attach the HTML content using the MIMEText
class, specifying 'html' as the second argument to indicate the content type.
Handling Attachments
Emails often include attachments such as documents, images, or other files. Python makes it easy to add attachments to your emails. Here's how you can send an email with an attachment:
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(subject, body, to_email, from_email, smtp_server, smtp_port, username, password, attachment_path):
# Create a MIMEMultipart object to represent the email
msg = MIMEMultipart()
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
# Attach the email body
msg.attach(MIMEText(body, 'plain'))
# Open the file to be sent
with open(attachment_path, 'rb') as attachment:
# Create a MIMEBase object
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
# Encode the file in ASCII characters to send by email
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={attachment_path}')
# Attach the file to the email
msg.attach(part)
# Connect to the SMTP server and send the email
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls()
server.login(username, password)
server.sendmail(from_email, to_email, msg.as_string())
# Usage example
send_email_with_attachment(
subject="Email with Attachment",
body="Please find the attachment below.",
to_email="[email protected]",
from_email="[email protected]",
smtp_server="smtp.example.com",
smtp_port=587,
username="your_username",
password="your_password",
attachment_path="/path/to/your/file.txt"
)
In this script, we use the MIMEBase
class to create an attachment object. We read the file in binary mode and encode it using Base64 encoding, which is necessary for email transmission. The Content-Disposition
header is set to indicate that the email contains an attachment, and the file is attached to the email using the attach()
method.
Conclusion
Automating email tasks with Python and the SMTP protocol can greatly enhance productivity and streamline communication processes. By understanding the basics of SMTP and leveraging Python's libraries, you can send plain-text and HTML emails, handle attachments, and integrate email functionalities into your applications. As you become more familiar with these tools, you can explore advanced features, such as sending emails to multiple recipients, scheduling emails, and integrating with email marketing platforms.
Whether you're a developer looking to automate notifications, a marketer aiming to reach a wider audience, or simply someone interested in Python's capabilities, mastering email automation with Python is a valuable skill that can open up numerous opportunities.
Now answer the exercise about the content:
What is the primary protocol used for sending emails across the Internet, which Python can interact with for automating email tasks?
You are right! Congratulations, now go to the next page
You missed! Try again.
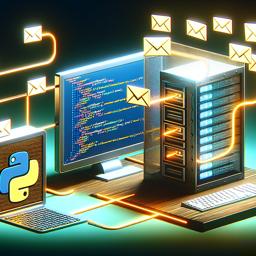
Next page of the Free Ebook: