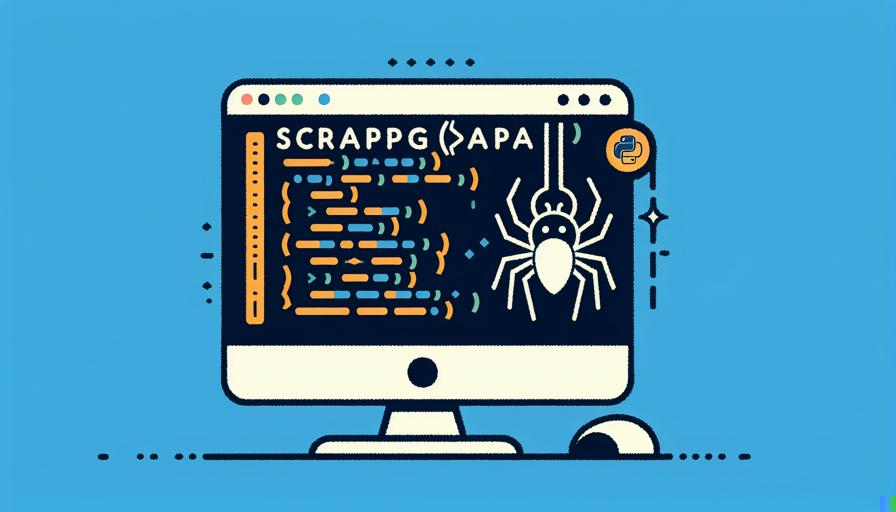
16. Scraping Data with Scrapy
Page 36 | Listen in audio
Scraping Data with Scrapy
Web scraping is an essential skill for anyone looking to automate data collection from the internet. One of the most powerful and efficient tools for this task is Scrapy, an open-source web crawling framework for Python. In this chapter, we will delve into the intricacies of using Scrapy to extract data from websites, covering everything from installation to advanced usage.
What is Scrapy?
Scrapy is a robust framework for building web spiders, which are programs that automatically browse the web and extract data. Unlike other web scraping tools, Scrapy provides a comprehensive toolkit for managing requests, handling responses, and storing extracted data. It is designed to be fast, efficient, and scalable, making it suitable for both small and large-scale scraping projects.
Installing Scrapy
Before you can start using Scrapy, you need to have Python installed on your system. Scrapy is compatible with Python 3.6 and above. To install Scrapy, you can use pip, the Python package manager, by running the following command:
pip install scrapy
Once installed, you can verify the installation by running:
scrapy version
This command should display the current version of Scrapy installed on your system.
Setting Up a Scrapy Project
Scrapy organizes its work into projects. To create a new Scrapy project, navigate to the directory where you want to store your project and run:
scrapy startproject project_name
This command will create a directory structure with files and folders necessary for your Scrapy project. The most important components of a Scrapy project are:
- spiders/: This directory contains your spider definitions. Spiders are classes that define how a certain site (or a group of sites) will be scraped.
- items.py: This file is where you define the data structures for the items you want to scrape.
- pipelines.py: This file is used to process the data after it has been scraped.
- settings.py: This file contains the settings for your Scrapy project.
Creating Your First Spider
Spiders are at the heart of any Scrapy project. They are responsible for making requests to websites and parsing the responses. To create a new spider, navigate to the spiders/
directory and create a new Python file. Here is a simple example of a spider that scrapes quotes from a website:
import scrapy
class QuotesSpider(scrapy.Spider):
name = "quotes"
start_urls = [
'http://quotes.toscrape.com/page/1/',
]
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('span small.author::text').get(),
'tags': quote.css('div.tags a.tag::text').getall(),
}
next_page = response.css('li.next a::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
In this example, the spider starts by making a request to the URL specified in start_urls
. The parse
method is called with the response object, and it extracts the desired data using CSS selectors. The spider then follows pagination links to continue scraping additional pages.
Running the Spider
To run your spider, use the following command:
scrapy crawl quotes
This command will execute the spider and output the scraped data to the console. To save the data to a file, you can use the -o
option:
scrapy crawl quotes -o quotes.json
This will save the scraped data in JSON format to a file named quotes.json
.
Handling Dynamic Content
Many modern websites use JavaScript to load content dynamically, which can be challenging for traditional web scrapers. However, Scrapy can be integrated with tools like Selenium to handle dynamic content. Selenium is a web automation tool that can control a web browser, allowing you to interact with dynamic elements before scraping the content.
To use Selenium with Scrapy, you'll need to install the Selenium package and the appropriate web driver for your browser. Here's a basic setup for integrating Selenium with Scrapy:
from scrapy import Spider
from selenium import webdriver
from scrapy.selector import Selector
class DynamicSpider(Spider):
name = 'dynamic'
def __init__(self):
self.driver = webdriver.Chrome(executable_path='path/to/chromedriver')
def parse(self, response):
self.driver.get(response.url)
sel = Selector(text=self.driver.page_source)
# Use sel to extract data with Scrapy selectors
self.driver.quit()
In this setup, Selenium opens a browser window and loads the page, allowing any JavaScript to execute. The page source is then passed to Scrapy's Selector
for data extraction.
Advanced Scrapy Features
Item Pipelines
Item pipelines are used to process items after they have been scraped. They provide a mechanism to clean, validate, and store the data. You can enable item pipelines in the settings.py
file and define them in pipelines.py
:
class MyPipeline:
def process_item(self, item, spider):
# Process the item here
return item
Middlewares
Scrapy middlewares are hooks into the Scrapy request/response processing. They allow you to modify requests and responses, handle exceptions, and more. Middlewares can be customized to add functionalities such as rotating user agents, handling retries, and more.
Scrapy Shell
The Scrapy shell is a powerful interactive tool for trying out your scraping logic. It allows you to test CSS and XPath selectors, inspect responses, and debug your spiders. You can launch the Scrapy shell with:
scrapy shell 'http://quotes.toscrape.com/page/1/'
Within the shell, you can experiment with different selectors and view the extracted data.
Best Practices for Web Scraping
While web scraping is a powerful tool, it's important to use it responsibly. Here are some best practices to follow:
- Respect Robots.txt: Always check the
robots.txt
file of a website to understand the scraping policies and adhere to them. - Avoid Overloading Servers: Be mindful of the load your scraping activities might place on a server. Implement delays between requests if necessary.
- Handle Errors Gracefully: Implement error handling to manage unexpected issues during scraping.
- Keep Your Code Maintainable: Write clean and modular code to make it easier to maintain and update your spiders.
Conclusion
Scrapy is a versatile and powerful tool for web scraping, offering a wide range of features to handle both simple and complex scraping tasks. By understanding its core components and capabilities, you can automate data extraction efficiently and effectively. Remember to always scrape responsibly and respect the terms of service of the websites you are targeting.
With the knowledge gained in this chapter, you are well-equipped to tackle a variety of web scraping projects and automate data collection tasks in your everyday workflow.
Now answer the exercise about the content:
What is one of the key advantages of using Scrapy over other web scraping tools?
You are right! Congratulations, now go to the next page
You missed! Try again.
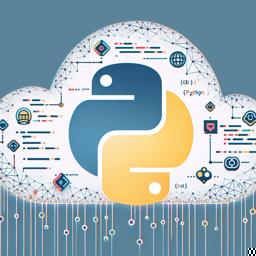
Next page of the Free Ebook: