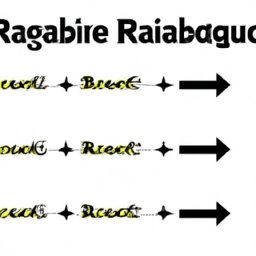
15. Recursion in programming
Página 15
Recursion is a fundamental concept in programming logic that may seem complex at first glance, but which, when well understood, can be a powerful tool for problem solving. In simple terms, recursion is the process by which a function, within its definition, calls itself. Recursion is used to solve problems that can be broken down into smaller problems of a similar nature.
A classic example of a problem that can be solved recursively is calculating the factorial of a number. The factorial of a number n is the product of all positive integers less than or equal to n. However, the factorial of n can also be defined as the product of n and the factorial of n-1. This gives us a recursive definition for calculating the factorial.
In pseudocode, the factorial function can be written recursively as follows:
function factorial(n) { if (n == 0) { return 1; } else { return n * factorial(n-1); } }
Note that the factorial function calls itself within its definition. This is what characterizes a recursive function. However, it is important to note that a recursive function must always have a stop condition, otherwise it will continue to call itself indefinitely, resulting in an infinite loop. In the case of the factorial function, the stopping condition is when n equals 0.
Another common example of a problem that can be solved recursively is the Fibonacci sequence. The Fibonacci sequence is a sequence of numbers in which each number is the sum of the two previous numbers. The first two numbers in the Fibonacci sequence are 0 and 1, and each subsequent number is the sum of the previous two numbers.
In pseudocode, the Fibonacci function can be written recursively as follows:
function fibonacci(n) { if (n == 0) { return 0; } else if (n == 1) { return 1; } else { return fibonacci(n-1) + fibonacci(n-2); } }
Like the factorial function, the Fibonacci function also calls itself within its definition and has a stopping condition to avoid an infinite loop.
Recursion can be a very powerful tool in solving programming problems. However, it's important to note that recursion can be more difficult to understand and track than an iterative approach. Also, recursion can be more inefficient in terms of memory usage and execution time than an iterative approach. Therefore, it is important to use recursion judiciously and only when it provides a clearer and more elegant solution to the problem at hand.
In summary, recursion is a fundamental concept in programming logic that involves a function calling itself within its definition. Recursion is used to solve problems that can be broken down into smaller problems of a similar nature. While recursion can be a powerful tool, it's important to use it judiciously, as it can be more difficult to understand and more inefficient than an iterative approach.
Now answer the exercise about the content:
What characterizes a recursive function in programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
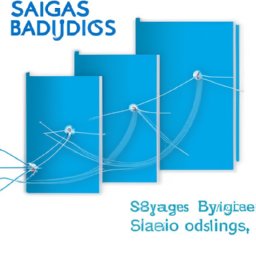
Next page of the Free Ebook: