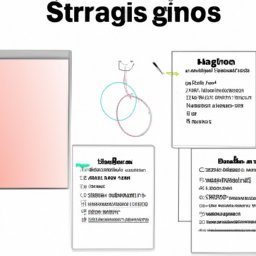
13. String Manipulation
Page 40 | Listen in audio
String manipulation is an essential part of programming logic and is a fundamental skill for any beginning programmer to learn. A string is a sequence of characters, which can include letters, numbers, spaces, and symbols. Strings are used in almost every program, from displaying messages on the screen to reading and writing text files.
There are many operations you can perform on strings. Some of the more common ones include concatenating (or joining) two or more strings, splitting a string into multiple parts, replacing parts of a string, converting a string to uppercase or lowercase, and checking whether a string contains another string.
The string concatenation is a very common operation. For example, you might want to combine a person's first and last name into a single string. In many programming languages, you can do this using the + operator. For example, in Python, you can write 'Alice' + ' ' + 'Smith' to get the string 'Alice Smith'.
string splitting is another common operation. For example, you might want to split a string containing a date in the format 'year-month-day' into three separate strings. In Python, you can do this using the split() method. For example, '2022-01-01'.split('-') returns the list ['2022', '01', '01'].
String replacement is useful when you want to replace all occurrences of a string with another. In Python, you can do this using the replace() method. For example, 'Hello, world!'.replace('world', 'Alice') returns the string 'Hello, Alice!'
The conversion of strings to uppercase or lowercase is useful when you want to compare two strings in a case-insensitive way (that is, ignoring whether the letters are uppercase or lowercase). In Python, you can do this using the upper() and lower() methods. For example, 'Alice'.upper() returns 'ALICE' and 'Alice'.lower() returns 'alice'.
The substring check is useful when you want to know if a string contains another string. In Python, you can do this using the in operator. For example, 'Alice' in 'Hello Alice!' returns True, while 'Bob' in 'Hello Alice!' returns False.
These are just a few of the many operations you can perform on strings. String manipulation is an essential part of programming logic, and it's a skill you'll use constantly as a programmer. Therefore, it is important that you practice these operations and feel comfortable using them.
Also, it's important to remember that strings are immutable in many programming languages, including Python. This means that once a string is created it cannot be changed. Instead, all string manipulation operations return a new string and leave the original unchanged. This can be a little confusing at first, but you'll get used to it with practice.
In short, string manipulation is an essential part of programming logic. It involves many different operations, including concatenation, division, substitution, case conversion, and checking substrings. Practice these operations and you'll be well on your way to becoming a competent programmer.
Now answer the exercise about the content:
Which of the following statements about string manipulation in Python is true?
You are right! Congratulations, now go to the next page
You missed! Try again.
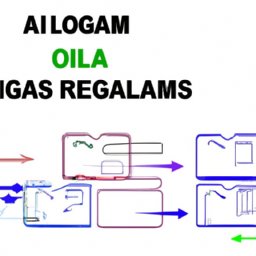
Next page of the Free Ebook: