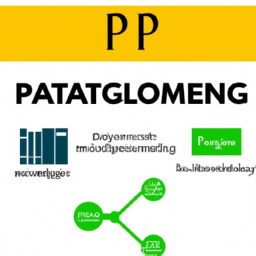
2.4. Python Language Fundamentals: Operators in Python
Página 6
2.4. Python Language Fundamentals: Operators in Python
The Python programming language is recognized for its simplicity and efficiency. One of the foundations of this language are operators, which are used to perform operations between variables and values. In this chapter, we will explore the different types of operators in Python and how they are used in backend development.
Arithmetic Operators
Arithmetic operators are used with numeric values to perform common mathematical operations. These include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), exponentiation (**), and integer division (//).
Examples:
x = 10 y = 3 print(x + y) # Output: 13 print(x - y) # Output: 7 print(x * y) # Output: 30 print(x / y) # Output: 3.3333333333333335 print(x % y) # Output: 1 print(x ** y) # Output: 1000 print(x // y) # Output: 3
These operators are fundamental to any mathematical calculation in Python.
Assignment Operators
Assignment operators are used to assign values to variables. In addition to the basic assignment operator (=), Python also supports compound assignment operators such as +=, -=, *=, /=, %=, **=, and //=.
Examples:
x = 10 x += 3 # Equivalent to x = x + 3 print(x) # Output: 13 x *= 2 # Equivalent to x = x * 2 print(x) # Output: 26
These operators are useful for modifying the value of a variable in a concise way.
Comparison Operators
Comparison operators are used to compare two values. These include equal (==), not equal (!=), greater than (>), less than (<), greater than or equal (>=), and less than or equal (<=).
Examples:
x = 10 y = 3 print(x == y) # Output: False print(x != y) # Output: True print(x > y) # Output: True print(x < y) # Output: False print(x >= y) # Output: True print(x <= y) # Output: False
These operators are essential for making comparisons and making decisions in our code.
Logical Operators
Logical operators are used to combine conditional statements. These include and, or and not.
Examples:
x = 10 print(x > 5 and x < 15) # Output: True print(x > 5 or x < 9) # Output: True print(not(x > 5 and x < 15)) # Output: False
These operators are vital for controlling program flow based on various conditions.
Identity Operators and Members
The identity (is, is not) and member (in, not in) operators are used to compare the identity of objects and check whether a value is a member of a sequence, respectively.
Examples:
x = ['apple', 'banana'] print('banana' in x) # Output: True print('orange' not in x) # Output: True y = x print(y is x) # Output: True print(y is not x) # Output: False
These operators are useful for manipulating and comparing complex objects, such as lists and dictionaries.
In summary, operators in Python are powerful tools that allow you to manipulate and compare values and variables in different ways. They are fundamental for building complex logic and advanced functionality into your Python programs.
Now answer the exercise about the content:
What are the different types of operators in Python and how are they used?
You are right! Congratulations, now go to the next page
You missed! Try again.
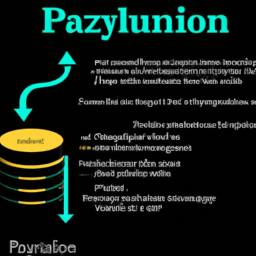
Next page of the Free Ebook: