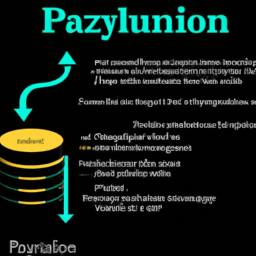
2.5. Python Language Fundamentals: Flow Control in Python
Página 7
Python is a high-level, interpreted, object-oriented programming language. It is known for its clear and readable syntax, which makes it easy to learn and understand. In this chapter, we will explore the fundamentals of the Python language, focusing on control flow.
Flow Control
Flow control in Python refers to the order in which code is executed. In Python, flow control is managed through conditional structures and loops. Conditional structures allow code to be executed based on certain conditions, while loops allow code to be executed repeatedly.
1. Conditional structures
In Python, conditional structures include the if statement, the elif statement, and the else statement. The if statement is used to test a condition and execute a block of code if the condition is true. The elif statement is used to test an additional condition if the if condition is false. The else statement is used to execute a block of code if all previous conditions are false.
ifcondition1:
# block of code to be executed if condition1 is true
elif condition2:
# block of code to be executed if condition2 is true
else:
# block of code to be executed if all previous conditions are false
2. Loops
Python supports two types of loops: for and while. The for loop is used to iterate over a sequence (such as a list, tuple, dictionary, set, or string) or an iterator. The while loop is used to repeat a block of code while a condition is true.
2.1. Loop For
The structure of the for loop in Python is as follows:
for item in sequence:
# block of code to be executed for each item in the sequence
The item can be any variable, and the sequence can be any iterable object. The code block inside the for loop will be executed once for each item in the sequence.
2.2. Loop While
The structure of the while loop in Python is as follows:
while condition:
# block of code to be executed while the condition is true
The condition can be any expression that evaluates to true or false. The code block inside the while loop will be executed repeatedly as long as the condition is true.
Conclusion
Understanding flow control in Python is essential for writing programs that perform complex tasks. Conditional structures and loops let you control the order and frequency in which code runs, allowing you to create programs that respond to different conditions and perform repetitive tasks efficiently.
In the next chapter, we'll dive deeper into Python functions, a powerful feature that lets you group and reuse blocks of code.
Now answer the exercise about the content:
What are the types of conditional structures and loops in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
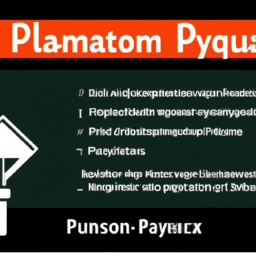
Next page of the Free Ebook: