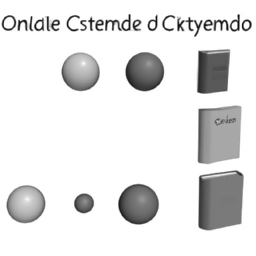
16.9. Object Orientation in C#: Method Overloading
Page 25 | Listen in audio
In the context of game programming with Unity, a fundamental concept is Object Orientation in C#, particularly Method Overloading. Method overloading is a feature of object-oriented programming that allows a programmer to use the same name for different methods that perform similar tasks but are differentiated by the number and/or type of arguments.
In C#, methods are blocks of code that perform a specific task. They are defined within classes and can be called or invoked in other parts of the code. Method overloading, also known as method overloading, is a technique that allows multiple methods in the same class to have the same name but different parameter lists.
For example, you might have a method called Add
that adds two integers. But what if you want to add two floating point numbers? Or three whole numbers? Instead of creating new methods with different names, like AddFloats
or AddThreeInts
, you can simply overload the Add
method to accept different numbers and types of parameters.
An example of code in C# that demonstrates method overloading is the following:
public class Calculator { public int Add(int a, int b) { return a + b; } public float Add(float a, float b) { return a + b; } public int Add(int a, int b, int c) { return a + b + c; } }
In this example, the Add
method is overloaded three times: once to add two integers, once to add two floating-point numbers, and once to add three integers.
Method overloading is very useful for keeping code clean and organized. Instead of having to remember different method names for similar tasks, you can simply remember one method name and use the appropriate parameter list for the task you want to perform.
In addition, method overloading can also improve code readability. If you see the Add
method being called, you immediately know that its purpose is to add numbers, regardless of the number or type of parameters.
It is important to note that method overriding is different from method overriding, also known as method overriding. Method overriding is a technique that allows a method in a subclass to have the same name and parameter list as a method in its superclass, but with a different implementation. Method overloading, on the other hand, occurs within the same class and the overloaded methods have the same implementation but different parameter lists.
In summary, method overloading is a powerful tool in object-oriented programming in C#, allowing you to write cleaner, more organized, and more readable code. It's a fundamental concept to understand when programming games with Unity and C#.
Now answer the exercise about the content:
What is method overloading in C# in the context of game programming with Unity?
You are right! Congratulations, now go to the next page
You missed! Try again.
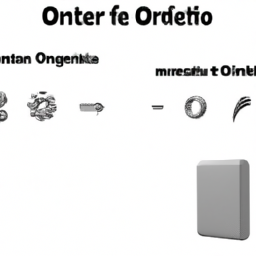
Next page of the Free Ebook: