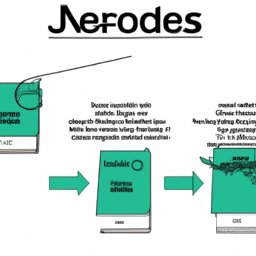
3.10. NodeJS Basics: Error Handling
Page 13 | Listen in audio
3.10. NodeJS Basics: Error Handling
One of the most critical aspects of software development is error handling. In NodeJS, this process is no different. This ebook chapter will explore the basics of NodeJS, with a special focus on error handling. Let's dive into how to identify, handle, and prevent errors in your NodeJS APIs.
What is error handling?
Error handling, also known as exception handling, is a process that responds to an error that occurs during the execution of a program. In NodeJS, error handling is done through a set of techniques and structures that allow the developer to deal with errors in an efficient and controlled way.
Why is error handling important?
Error handling is important because it allows developers to control the flow of execution of a program when an error occurs. Without proper error handling, a program can crash unexpectedly, resulting in a poor user experience and possible data loss. In addition, error handling is also essential for application security as it helps prevent attacks and exploits.
Types of errors in NodeJS
In NodeJS, errors can be classified into three main categories: standard errors, user errors, and system errors. Standard errors are errors that occur due to problems in the code, such as references to undefined variables. User errors occur when the user enters invalid data or performs disallowed actions. System errors, on the other hand, are errors that occur due to problems in the runtime environment, such as running out of memory or insufficient file permissions.
Handling errors in NodeJS
In NodeJS, error handling is mainly done through try-catch blocks and callback functions with error parameters. The try-catch block is used to catch and handle errors that occur during the execution of a block of code. The callback function with an error parameter, on the other hand, is used to handle errors that occur during the execution of asynchronous operations.
Error handling with try-catch blocks
A try-catch block in NodeJS has the following structure:
try { // code that might throw an error } catch (error) { // code that handles the error }
In the try block, you place code that might throw an error. If an error occurs while executing this code, execution of the try block stops and control is passed to the catch block, which contains the code that handles the error.
Error handling with callback functions
Callback functions in NodeJS usually have an error parameter as their first argument. If an error occurs during the execution of the callback function, that error is passed to the error parameter. The code that calls the callback function can then check if the error parameter is null or undefined to determine if an error has occurred.
fs.readFile('file.txt', function(error, data) { if (error) { // handle the error } else { // process the data } });
Conclusion
Error handling is a crucial component of software development. In NodeJS, error handling can be done in an efficient and controlled way through try-catch blocks and callback functions with error parameters. By understanding and applying these concepts, you'll be one step closer to building robust and reliable APIs in NodeJS.
Now answer the exercise about the content:
What are the three main categories of errors in NodeJS and how are they handled?
You are right! Congratulations, now go to the next page
You missed! Try again.
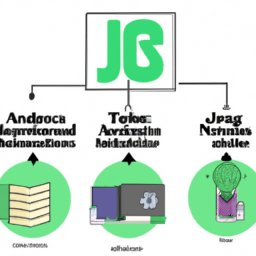
Next page of the Free Ebook: