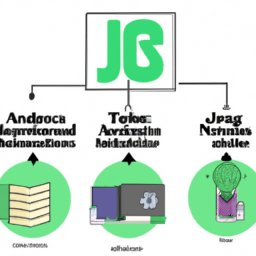
3.11. NodeJS Basics: Automated Testing
Página 14
3.11. NodeJS Basics: Automated Testing
Automated testing is a crucial part of software development, and NodeJS is no exception. The ability to write automated tests for your code allows you to quickly check if recent changes have broken anything and helps ensure your code is working properly.
Automated tests in NodeJS can be divided into several categories, including unit tests, integration tests, and user acceptance tests. Each of these types of tests has a specific role in the process of assuring software quality.
Unit Tests
Unit tests are written to test a single unit of code, such as a function or a method. The purpose of a unit test is to verify that the unit of code is working correctly in a variety of scenarios.
In NodeJS, unit tests are usually written using a test library like Mocha or Jest. These libraries provide a framework for defining and running tests, as well as helper functions for making assertions about code behavior.
For example, a unit test for a function that adds two numbers might look like this:
const assert = require('assert'); const sum = require('./sum'); it('should sum two numbers', () => { const result = sum(1, 2); assert.equal(result, 3); });
In this example, the 'sum' function is tested to see if it returns the correct sum of two numbers. If the function is not working correctly, the test will fail and the developer will be informed of the problem.
Integration Tests
Integration tests are used to verify that different parts of a system work correctly together. In NodeJS, this can involve testing how different modules interact with each other, or how your code interacts with external services or the file system.
Integration tests are typically more complex than unit tests because they involve configuring and cleaning up external resources. However, they are vital to ensuring that the entire system is working properly.
For example, an integration test for a REST API might look like this:
const request = require('supertest'); const app = require('./app'); it('should return a list of users', async () => { const response = await request(app).get('/users'); assert.equal(response.status, 200); assert(Array.isArray(response.body)); });
In this example, the API is tested to verify that it returns a list of users when the '/users' route is accessed. If the API is not working correctly, the test will fail and the developer will be informed of the problem.
User Acceptance Tests
User acceptance testing, also known as end-to-end testing or E2E testing, is used to verify that a system is functioning correctly from the user's point of view. In NodeJS, this can involve testing a complete web application, including the UI and server interaction.
User acceptance tests are often the most complex to write and run, as they involve simulating user actions in a production environment. However, they are the best way to ensure the system is working properly for end users.
For example, a user acceptance test for a web application might use a tool like Puppeteer or Cypress to simulate user actions and verify that the application is responding correctly. If the application is not working correctly, the test will fail and the developer will be informed of the problem.
In short, automated testing is a vital part of NodeJS development. They help ensure that code is working correctly, allow developers to confidently refactor code, and help prevent bugs from being introduced into software. Mastering the concepts of automated testing in NodeJS is an essential skill for any NodeJS developer.
Now answer the exercise about the content:
_Which of the following is the purpose of a unit test in NodeJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
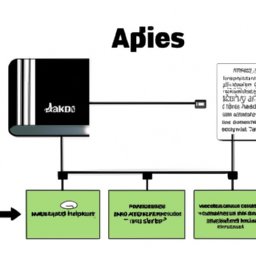
Next page of the Free Ebook: