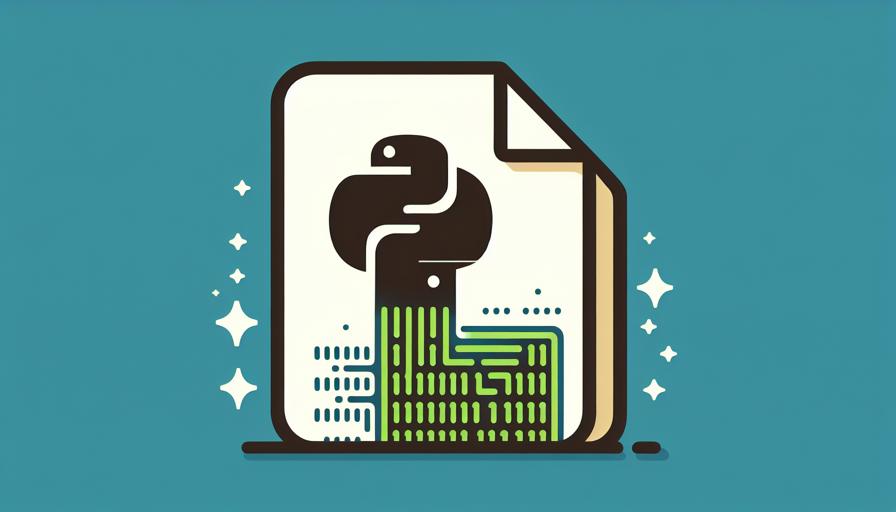
10. Manipulating PDF Files with Python
Page 10 | Listen in audio
Manipulating PDF Files with Python
PDF (Portable Document Format) files are ubiquitous in the digital world, offering a reliable way to present documents independent of software, hardware, or operating systems. However, working with PDFs programmatically can be challenging due to their complex structure. Fortunately, Python provides powerful libraries that simplify the task of manipulating PDF files, enabling automation of tasks ranging from simple extraction of text to complex editing operations.
Introduction to PDF Processing
Before diving into the specifics of manipulating PDFs with Python, it's essential to understand the basic structure of a PDF file. A PDF document is composed of a series of objects, including pages, fonts, annotations, and more. Each page contains a sequence of content streams that describe the visual elements on that page. These elements can include text, images, and vector graphics.
Manipulating PDFs involves reading these objects, altering them as needed, and writing the changes back to a PDF file. Python, with its rich ecosystem of libraries, provides tools to handle these tasks effectively.
Popular Python Libraries for PDF Manipulation
Several Python libraries are available for working with PDFs. Some of the most popular ones include:
- PyPDF2: A pure Python library capable of splitting, merging, and transforming PDF documents. It's a go-to library for basic PDF manipulation tasks.
- PDFMiner: A tool for extracting information from PDF documents, focusing on text extraction and layout analysis.
- ReportLab: A library for creating PDFs from scratch, offering extensive options for designing and generating complex PDF documents.
- pdfrw: A library for reading and writing PDF files, with support for merging and modifying existing documents.
- PyMuPDF (fitz): A fast and lightweight library that provides extensive functionality for PDF manipulation, including text extraction, annotations, and more.
Basic PDF Operations with PyPDF2
PyPDF2 is a versatile library that allows you to perform basic operations on PDF files. Here's a quick overview of how to use it for common tasks:
Installing PyPDF2
pip install PyPDF2
Reading PDF Files
To read a PDF file, you can use PyPDF2's PdfReader
class. Here's an example:
from PyPDF2 import PdfReader
# Open the PDF file
reader = PdfReader("example.pdf")
# Get the number of pages
num_pages = len(reader.pages)
# Extract text from each page
for page_num in range(num_pages):
page = reader.pages[page_num]
text = page.extract_text()
print(f"Page {page_num + 1}: {text}")
Splitting and Merging PDFs
PyPDF2 allows you to split a PDF into individual pages and merge multiple PDFs into a single document:
from PyPDF2 import PdfWriter
# Splitting a PDF
reader = PdfReader("example.pdf")
writer = PdfWriter()
# Add the first page to a new PDF
writer.add_page(reader.pages[0])
# Save the new PDF
with open("split_page.pdf", "wb") as output_pdf:
writer.write(output_pdf)
# Merging PDFs
merger = PdfWriter()
# Append PDFs to the merger
merger.append("example1.pdf")
merger.append("example2.pdf")
# Write the merged PDF
with open("merged.pdf", "wb") as output_pdf:
merger.write(output_pdf)
Rotating and Cropping Pages
PyPDF2 also provides methods to rotate and crop pages:
# Rotate a page
page = reader.pages[0]
page.rotate_clockwise(90)
# Crop a page
page.cropbox.lower_left = (0, 0)
page.cropbox.upper_right = (300, 300)
# Save the modified PDF
writer = PdfWriter()
writer.add_page(page)
with open("modified.pdf", "wb") as output_pdf:
writer.write(output_pdf)
Advanced PDF Text Extraction with PDFMiner
While PyPDF2 is great for basic PDF manipulation, it falls short when it comes to advanced text extraction. PDFMiner is a more powerful tool for this purpose, offering fine-grained control over text extraction and layout analysis.
Installing PDFMiner
pip install pdfminer.six
Extracting Text with PDFMiner
PDFMiner provides a command-line tool and a Python API for extracting text from PDFs. Here's how to use the Python API:
from pdfminer.high_level import extract_text
# Extract text from a PDF
text = extract_text("example.pdf")
print(text)
PDFMiner's strength lies in its ability to preserve the layout of the original document, making it ideal for extracting tabular data or text with complex formatting.
Creating PDFs with ReportLab
While PyPDF2 and PDFMiner focus on reading and modifying existing PDFs, ReportLab is designed for creating PDFs from scratch. It provides a comprehensive set of tools for generating complex documents with custom fonts, graphics, and layouts.
Installing ReportLab
pip install reportlab
Generating a Simple PDF
With ReportLab, you can create a PDF by defining a canvas and drawing on it:
from reportlab.pdfgen import canvas
# Create a PDF with a canvas
c = canvas.Canvas("hello.pdf")
# Draw text on the canvas
c.drawString(100, 750, "Hello, World!")
# Save the PDF
c.save()
Adding Graphics and Images
ReportLab allows you to add graphics and images to your PDFs:
# Draw a rectangle
c.rect(100, 700, 200, 100)
# Insert an image
c.drawImage("example.jpg", 100, 500, width=200, height=150)
# Save the PDF
c.save()
Conclusion
Manipulating PDF files with Python can significantly streamline your document processing tasks. Whether you need to extract text, modify existing PDFs, or generate new documents, Python's libraries provide robust solutions to meet your needs. By leveraging tools like PyPDF2, PDFMiner, and ReportLab, you can automate a wide range of PDF-related tasks, enhancing productivity and efficiency in your workflows.
As you delve deeper into PDF manipulation, you'll discover even more advanced capabilities, such as adding annotations, encrypting PDFs, and more. With Python's versatility and the power of its libraries, the possibilities for automating PDF tasks are virtually limitless.
Now answer the exercise about the content:
Which Python library is primarily used for creating PDFs from scratch, offering extensive options for designing and generating complex PDF documents?
You are right! Congratulations, now go to the next page
You missed! Try again.
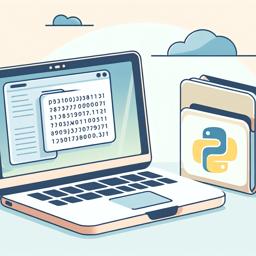
Next page of the Free Ebook: