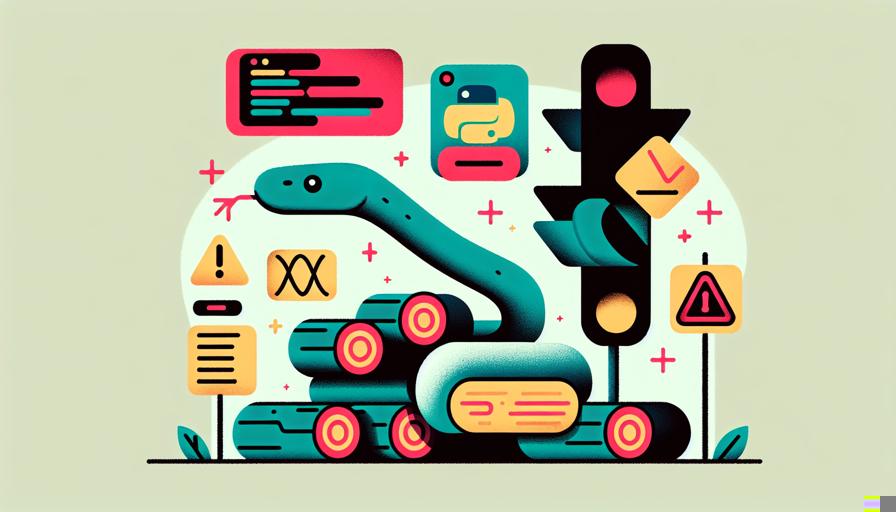
34. Logging and Error Handling in Automated Scripts
Page 84 | Listen in audio
Logging and Error Handling in Automated Scripts
In the world of automation, particularly when using Python to automate everyday tasks, logging and error handling play a crucial role. They ensure that your scripts run smoothly and provide valuable insights when things go awry. This chapter delves into the importance of these two components and how to implement them effectively in your Python scripts.
Understanding Logging
Logging is the process of recording information about your program's execution. When you automate tasks, it's essential to have a mechanism that allows you to track what your script is doing, identify where it might be failing, and gather data for debugging and analysis. Python's logging
module provides a flexible framework for emitting log messages from Python programs.
Why Use Logging?
- Debugging: Logs can help you understand the flow of your program and pinpoint where things might be going wrong.
- Monitoring: In automated systems, logs can serve as a record of what happened, when, and why. This is crucial for monitoring the health and performance of your scripts.
- Audit and Compliance: Logs can provide a historical record of actions taken by your scripts, which is essential for auditing and compliance purposes.
- Performance Optimization: By analyzing logs, you can identify bottlenecks and optimize your scripts for better performance.
Setting Up Logging in Python
The logging
module in Python is powerful and highly configurable. Here's a simple example of how to set up logging in your script:
import logging
# Configure logging
logging.basicConfig(filename='app.log', filemode='a', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
# Log messages
logging.debug('This is a debug message')
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
logging.critical('This is a critical message')
In this example, we configure the logger to write messages to a file named app.log
. The log level is set to INFO
, which means that all messages with a severity of INFO and above will be logged. The format of the log messages includes the timestamp, the level of the message, and the actual message.
Log Levels
Python's logging module defines several levels of severity for log messages:
- DEBUG: Detailed information, typically of interest only when diagnosing problems.
- INFO: Confirmation that things are working as expected.
- WARNING: An indication that something unexpected happened, or indicative of some problem in the near future (e.g., ‘disk space low’). The software is still working as expected.
- ERROR: Due to a more serious problem, the software has not been able to perform some function.
- CRITICAL: A very serious error, indicating that the program itself may be unable to continue running.
Error Handling
Error handling is the process of responding to and managing errors that occur in your programs. In automated scripts, robust error handling is vital to ensure that your scripts can deal with unexpected situations gracefully and continue to operate or fail safely.
Understanding Exceptions
In Python, errors are typically managed with exceptions. An exception is an event that occurs during the execution of a program that disrupts the normal flow of instructions. Python provides a rich framework for handling exceptions, allowing you to catch and respond to errors in a controlled manner.
Basic Exception Handling
The basic structure for handling exceptions in Python is the try
and except
block. Here's a simple example:
try:
# Code that might cause an exception
result = 10 / 0
except ZeroDivisionError:
# Code to handle the exception
print("You can't divide by zero!")
In this example, the code attempts to divide by zero, which raises a ZeroDivisionError
. The except
block catches this specific exception and handles it by printing a message.
Advanced Exception Handling
Python allows you to handle multiple exceptions, use an else
block to execute code if no exceptions occur, and use a finally
block to execute code regardless of whether an exception occurred:
try:
# Code that might cause an exception
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
except Exception as e:
print(f"An unexpected error occurred: {e}")
else:
print("The operation was successful.")
finally:
print("This will run no matter what.")
In this example, the finally
block is used to perform cleanup actions or release resources, ensuring that certain critical operations are completed regardless of whether an error occurred.
Raising Exceptions
Sometimes, you might want to raise an exception intentionally. This can be useful for enforcing constraints or signaling that an error condition has occurred:
def check_positive(number):
if number < 0:
raise ValueError("The number must be positive.")
return number
try:
check_positive(-10)
except ValueError as e:
print(e)
In this example, the function check_positive
raises a ValueError
if the input number is negative, and the calling code handles this exception appropriately.
Integrating Logging and Error Handling
Logging and error handling are complementary techniques. By integrating them, you can create scripts that not only handle errors gracefully but also provide detailed logs that can be used for debugging and analysis. Here's how you might combine logging and error handling in a script:
import logging
# Configure logging
logging.basicConfig(filename='app.log', filemode='a', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
logging.error("Attempted to divide by zero.")
raise
else:
logging.info(f"Division successful: {a} / {b} = {result}")
return result
finally:
logging.info("Division operation attempted.")
try:
divide_numbers(10, 0)
except ZeroDivisionError:
print("Handled division by zero.")
In this example, the function divide_numbers
attempts to divide two numbers. If a ZeroDivisionError
occurs, it logs an error message and re-raises the exception. The finally
block logs that a division operation was attempted, regardless of the outcome.
Best Practices
- Use Descriptive Log Messages: Ensure that your log messages are descriptive and provide enough context to understand what happened.
- Log at Appropriate Levels: Use the correct log level for your messages to ensure that critical issues are highlighted and less important information is available for debugging.
- Handle Specific Exceptions: Catch specific exceptions rather than using a general
except
clause. This makes your error handling more precise and meaningful. - Use Finally for Cleanup: Use the
finally
block to release resources or perform cleanup actions, ensuring that they are executed regardless of whether an exception occurred. - Regularly Review Logs: Make it a habit to review your logs regularly to identify patterns, potential issues, and opportunities for optimization.
By implementing robust logging and error handling in your automated scripts, you can create reliable, maintainable, and efficient solutions that handle unexpected situations gracefully and provide valuable insights into their operation.
Now answer the exercise about the content:
What is the purpose of using the `logging` module in Python scripts as described in the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
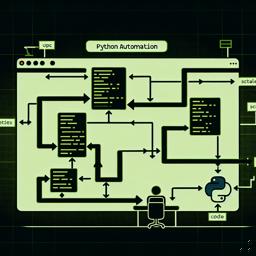
Next page of the Free Ebook: