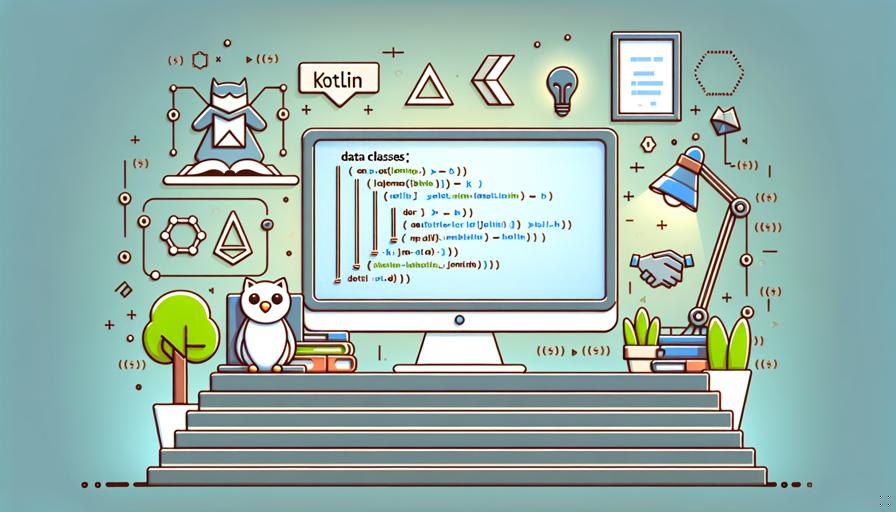
11. Kotlin Data Classes
Page 11 | Listen in audio
Kotlin, as a modern programming language, brings a lot of features that simplify Android app development. One of the most useful features is the data class. Data classes in Kotlin are specifically designed to hold data and provide utility functions automatically. This feature is especially beneficial in Android development, where data representation and manipulation are frequent tasks.
Data classes in Kotlin are defined using the data
keyword. They automatically generate several useful methods, such as toString()
, equals()
, hashCode()
, and copy()
. These methods are essential for handling data objects, making Kotlin data classes a powerful tool for developers.
To declare a data class in Kotlin, you simply use the data
keyword followed by the class declaration. For example:
data class User(val name: String, val age: Int)
In this example, User
is a data class with two properties: name
and age
. The primary constructor of a data class needs to have at least one parameter. The parameters of the primary constructor are automatically used to generate the equals()
, hashCode()
, toString()
, and copy()
methods.
One of the significant advantages of data classes is the automatic generation of the toString()
method. This method provides a string representation of the object, which is highly useful for debugging:
val user = User("Alice", 25)
println(user) // Output: User(name=Alice, age=25)
The equals()
and hashCode()
methods are also automatically generated. These methods are crucial for comparing objects and using them in collections like sets or as keys in maps. The equals()
method checks if two objects are equal by comparing their properties, while the hashCode()
method returns a hash code value for the object:
val user1 = User("Alice", 25)
val user2 = User("Alice", 25)
println(user1 == user2) // Output: true
The copy()
method is another powerful feature of data classes. It allows you to create a new instance of the data class with some properties modified. This is particularly useful when working with immutable objects:
val user3 = user1.copy(age = 26)
println(user3) // Output: User(name=Alice, age=26)
Data classes also support destructuring declarations, which enable you to unpack the values of an object into separate variables. This feature is handy when you want to work with individual properties of a data class:
val (name, age) = user
println("Name: $name, Age: $age") // Output: Name: Alice, Age: 25
One of the key considerations when using data classes is that they are designed for classes that primarily hold data. Therefore, they should not include complex logic or behavior. If a class requires significant functionality beyond data storage, it might be better suited as a regular class.
In addition to the basic features, data classes in Kotlin can implement interfaces. This allows you to design more complex data structures while still taking advantage of the benefits provided by data classes:
interface Identifiable {
val id: String
}
data class Product(override val id: String, val name: String, val price: Double) : Identifiable
In Android app development, data classes are often used in conjunction with libraries like Retrofit or Room. These libraries require data models to represent network responses or database entities, and data classes are an excellent fit for these purposes. For example, when working with Retrofit, you can define a data class to map JSON responses to Kotlin objects:
data class ApiResponse(val userId: Int, val id: Int, val title: String, val body: String)
Similarly, when using Room for database operations, data classes can be used to define entities. This makes it easier to work with database records as objects:
@Entity
data class UserEntity(
@PrimaryKey val userId: Int,
val userName: String,
val userEmail: String
)
It's important to note that while data classes provide a lot of functionality out of the box, they are not a one-size-fits-all solution. Developers should be mindful of the overhead introduced by the generated methods, especially in performance-critical applications.
Moreover, data classes are designed to be immutable by default, which aligns well with Kotlin's emphasis on immutability and functional programming principles. However, if mutability is necessary, Kotlin allows for mutable properties within data classes. This can be done by using var
instead of val
for the properties:
data class MutableUser(var name: String, var age: Int)
In conclusion, Kotlin data classes are a powerful feature that significantly simplifies the handling of data objects in Android app development. By providing automatic generation of essential methods and supporting destructuring declarations, data classes reduce boilerplate code and enhance code readability. They integrate seamlessly with libraries like Retrofit and Room, making them indispensable for tasks involving data representation and manipulation. However, developers should use them judiciously, ensuring that the simplicity and immutability of data classes align with the application's architectural needs.
Now answer the exercise about the content:
What is one of the most useful features of Kotlin that simplifies Android app development by automatically generating utility functions for handling data objects?
You are right! Congratulations, now go to the next page
You missed! Try again.
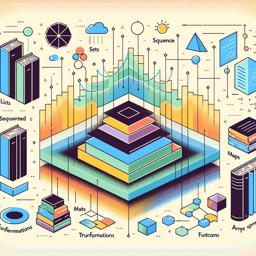
Next page of the Free Ebook: