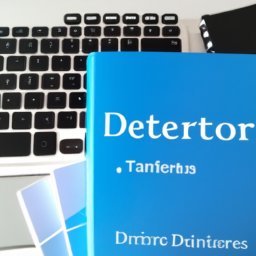
5.13. Introduction to object-oriented programming in Dart: Iterators
Página 69
Object-oriented programming (OOP) is a programming paradigm that uses the concept of "objects" to represent both data and procedures that can be applied to that data. In the context of Dart, a programming language developed by Google and used in Flutter app development, OOP is an essential part of the coding process. One of the most important aspects of OOP in Dart is the use of iterators. This article will explore the concept of iterators in Dart and how they are used in object-oriented programming.
An iterator, in simple terms, is an object that allows programmers to iterate through a collection, such as a list or a map. In Dart, iterators are provided by classes that implement the Iterator interface. This interface defines two main members: the moveNext() method and the current property. The moveNext() method advances the iterator to the next element in the collection and returns true if that element exists. The current property returns the current element in the collection.
To use an iterator in Dart, you would normally create a loop that continues as long as moveNext() returns true. Inside the loop, you can access the current element using the current property. Here is an example of how this can be done:
var list = [1, 2, 3, 4, 5]; var iterator = list.iterator; while (iterator.moveNext()) { print(iterator.current); }
This code will print each number in the list one at a time. Note that the iterator starts before the first element in the list, so you must call moveNext() once to advance to the first element.
While this is a simple example, iterators can be much more powerful. For example, you can define your own custom iterators by creating a class that implements the Iterator interface. This lets you control exactly how the iteration takes place. For example, you can create an iterator that traverses a list in reverse order, or one that skips certain elements.
Here is an example of a custom iterator that loops through a list in reverse order:
class ReverseIterator<T> implements Iterator<T> { List<T> _list; int _index; ReverseIterator(this._list) { _index = _list.length; } @override T get current => _list[_index]; @override bool moveNext() => --_index >= 0; } var list = [1, 2, 3, 4, 5]; var iterator = ReverseIterator(list); while (iterator.moveNext()) { print(iterator.current); }
This code will print the numbers in the list in reverse order.
In short, iterators are a vital part of object-oriented programming in Dart. They let you traverse collections in a flexible and custom way, making your code easier to read and maintain. Whether you're new to Dart or an experienced developer, understanding and using iterators correctly can help you write more efficient and effective code.
Now answer the exercise about the content:
What is an iterator in the Dart programming language and how is it used in object-oriented programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
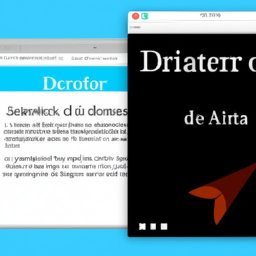
Next page of the Free Ebook: