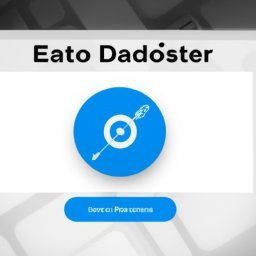
5.4. Introduction to object-oriented programming in Dart: Encapsulation
Page 60 | Listen in audio
5.4. Introduction to Object Oriented Programming in Dart: Encapsulation
Before we dive deep into the concept of encapsulation in Dart, it's critical that we understand object-oriented programming (OOP). OOP is a programming paradigm based on the concept of "objects", which can contain both data and code: data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods).< /p>
One of the main principles of Object Oriented Programming is Encapsulation. Encapsulation is a mechanism for hiding an object's implementation details. In other words, it protects the object's internal code and data from being accessed directly from outside the object.
In Dart, encapsulation is implemented using access modifiers. There are two types of access modifiers in Dart - 'public' and 'private'. By default, all classes, variables, methods, etc. are publicly accessible unless explicitly declared private. To make a variable or method private in Dart, we prefix its name with an underscore '_'.
Why is Encapsulation important?
Encapsulation is one of the four fundamental pillars of object-oriented programming (OOP), and is critical to code maintenance and error prevention. It helps control how data is accessed or modified. Furthermore, a well-encapsulated object is one that hides all its internal data and prevents it from being accessed directly, forcing access through methods. This is known as 'data hiding'.
Dart Encapsulation Example
To illustrate the concept of encapsulation, consider the example of a 'BankAccount' class. This class has two properties - 'accountNumber' and '_balance'. Here, '_balance' is a private variable, which means that it cannot be accessed or modified directly from outside the class.
class BankAccount { int accountNumber; double _balance; BankAccount(this.accountNumber, this._balance); double get balance => _balance; set balance(double newBalance) { if (newBalance >= 0) { _balance = newBalance; } else { print('Invalid balance'); } } }
In this example, the only way to access or modify the balance is through the 'get' and 'set' methods. This ensures that the balance cannot be set to a negative value, because the 'set' method checks whether the new balance is greater than or equal to zero before modifying the '_balance' variable.
Conclusion
In summary, encapsulation is a crucial principle of object-oriented programming in Dart. It allows us to hide an object's implementation details and control access to its data. This makes the code more secure and easier to maintain, as it prevents data from being modified inappropriately. When building apps with Flutter and Dart, understanding and correctly applying encapsulation can improve the quality of your code and the robustness of your app.
Now answer the exercise about the content:
What is encapsulation in Dart object-oriented programming and why is it important?
You are right! Congratulations, now go to the next page
You missed! Try again.
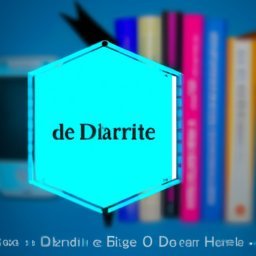
Next page of the Free Ebook: