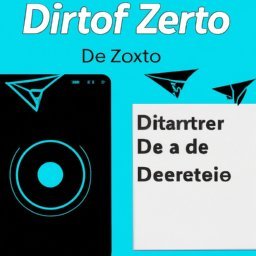
5. Introduction to object-oriented programming in Dart
Página 56
Object-oriented programming (OOP) is a programming paradigm that uses the idea of "objects" to represent data and methods. This is particularly useful when it comes to building complex applications, as it allows developers to better organize their code and make it more reusable. In this section, we'll introduce the concept of object-oriented programming in Dart, the programming language used by Flutter.
1. Classes and Objects
In object-oriented programming, a class is a blueprint or template from which objects are created. In Dart, you define a class using the class
keyword. For example, you might have a class called Person
that has properties like name
and age
. Once you have a class, you can create an object from it. For example:
class Person { String name; intage; } void main() { Person person = Person(); person.name = 'John'; person.age = 30; }
In this example, Person
is the class and person
is the object. Note that you can assign values to object properties using dot notation.
2. Methods
Methods are functions that belong to a class. They usually manipulate the properties of the class. For example, you might have a greet
method in the Person
class that prints out a personalized greeting:
class Person { String name; intage; void greet() { print('Hello, I am $name and I am $age years old.'); } } void main() { Person person = Person(); person.name = 'John'; person.age = 30; person.greet(); // prints: Hello, I am John and I am 30 years old. }
3. Constructors
A constructor is a special method that is called when an object is created from a class. In Dart, the constructor has the same name as the class. You can use the constructor to initialize the object's properties. For example:
class Person { String name; intage; Person(String name, int age) { this.name = name; this.age = age; } void greet() { print('Hello, I am $name and I am $age years old.'); } } void main() { Person person = Person('John', 30); person.greet(); // prints: Hello, I am John and I am 30 years old. }
In this example, the Person
constructor accepts two parameters and uses them to initialize the name
and age
properties.
4. Inheritance
Inheritance is a feature of object-oriented programming that allows a class to inherit properties and methods from another class. In Dart, you use the extends
keyword to create a child class. For example, you might have a Employee
class that inherits from Person
:
class Person { String name; intage; Person(String name, int age) { this.name = name; this.age = age; } void greet() { print('Hello, I am $name and I am $age years old.'); } } class Employee extends Person { String company; Employee(String name, int age, this.company) : super(name, age); } void main() { Employee employee = Employee('John', 30, 'Google'); employee.greet(); // prints: Hello, I am John and I am 30 years old. print(employee.company); // prints: Google }
In this example, Employee
is a child class of Person
and inherits its properties and methods. Note the use of super
in the Employee
constructor to call the Person
constructor.
5. Encapsulation
Encapsulation is the principle of hiding implementation details and allowing access only through methods. In Dart, you can use the _
(underscore) keyword to make a property or method private. For example:
class Person { String _name; int _age; Person(this._name, this._age); void greet() { print('Hello, I am $_name and I am $_age years old.'); } } void main() { Person person = Person('John', 30); person.greet(); // prints: Hello, I am John and I am 30 years old. }
In this example, the _name
and _age
properties are private, meaning they cannot be accessed outside the Person
class.< /p>
These are the basic concepts of object-oriented programming in Dart. They form the basis for building more complex and reusable Flutter apps.
Now answer the exercise about the content:
What is the purpose of a constructor in object-oriented programming in Dart?
You are right! Congratulations, now go to the next page
You missed! Try again.
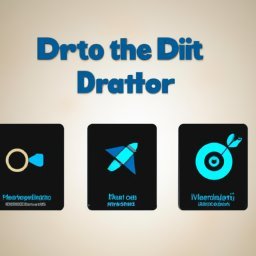
Next page of the Free Ebook: