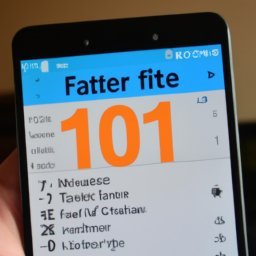
10.5. Internationalization and Localization in Flutter: Number Format
Page 138 | Listen in audio
Internationalization and localization are vital aspects of application development as they allow applications to be accessible and understandable to users from different regions and cultures. In Flutter, internationalization and localization are made easy through various libraries and packages, including the intl package. This package provides a lot of functionality, including number formatting, which is the focus of this text.
Number formatting is essential for presenting numeric information in a way that is easily understandable to users. For example, the way a number is formatted can vary depending on the region. In some countries, a dot is used to denote a decimal, while a comma is used to group thousands. In other countries, the opposite is true. Therefore, it is important to ensure that numbers are formatted according to the user's local preferences.
In Flutter, number formatting can be performed using the NumberFormat class from the intl package. This class provides a variety of methods for formatting numbers according to different standards and styles. For example, the NumberFormat.decimalPattern method can be used to format a number according to the local decimal pattern. Likewise, the NumberFormat.currency method can be used to format a number as a currency amount.
To use the NumberFormat class, you first need to add the intl package to your pubspec.yaml file. This can be done by adding the following line to your file:
dependencies: flutter: sdk: flutter intl: ^0.17.0
Once you've added the intl package, you can import it into your Dart file using the following line:
import 'package:intl/intl.dart';
You are now ready to use the NumberFormat class to format numbers. For example, to format a number according to the local decimal standard, you can use the following code:
var number = 1234567.89; var format = NumberFormat.decimalPattern('pt_BR'); var formattedNumber = format.format(number); print(formattedNumber); // Outputs: 1,234,567.89
This code creates a new NumberFormat object that uses the Brazilian decimal standard. It then uses this object to format the number 1234567.89, resulting in the string '1,234,567,89'.
Likewise, to format a number as a currency amount, you can use the following code:
var number = 1234567.89; var format = NumberFormat.currency(locale: 'pt_BR', symbol: 'R\$'); var formattedNumber = format.format(number); print(formattedNumber); // Outputs: BRL 1,234,567.89
This code creates a new NumberFormat object that uses the Brazilian currency format and the real symbol. It then uses this object to format the number 1234567.89, resulting in the string 'R$1,234,567.89'.
Therefore, internationalization and localization are key aspects in Flutter application development. By using the NumberFormat class from the intl package, you can ensure that numbers in your application are formatted in a way that is easily understandable to users, regardless of their region or culture.
In summary, internationalization and localization in Flutter, especially number formatting, is an essential skill for any Flutter developer. By learning and applying these skills, you can build apps that are truly global and accessible to users around the world.
Now answer the exercise about the content:
What is the function of the intl package in Flutter and how is it used in number formatting?
You are right! Congratulations, now go to the next page
You missed! Try again.
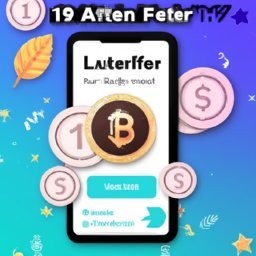
Next page of the Free Ebook: