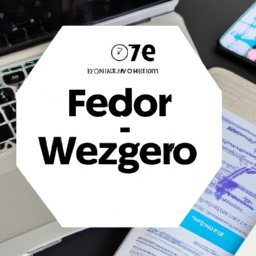
9.5. Integration with APIs and Web Services: Working with Websockets
Page 123 | Listen in audio
Integration with APIs and web services is a crucial part of modern application development. One of the most efficient ways to communicate with web services is through Websockets. In this chapter, we'll explore how to work with Websockets in Flutter and Dart.
Websockets is a communication protocol that provides real-time bidirectional communication between the client and the server. It is especially useful for applications that need instant updates, such as live chats, online games and stock trading applications.
Before we start working with Websockets, it is important to understand the difference between it and the traditional HTTP protocol. HTTP is a request-response based protocol, where the client makes a request to the server and waits for a response. This model is not ideal for situations where the server needs to send updates to the client without a specific request from the client.
On the other hand, Websockets allow real-time bidirectional communication. Once the connection is established, both the client and the server can send and receive data at any time. This makes Websockets an ideal choice for applications that need real-time updates.
To work with Websockets in Flutter and Dart, we need a library called 'web_socket_channel'. To add it to our project, we need to update the 'pubspec.yaml' file and include 'web_socket_channel' in the dependencies section.
dependencies: flutter: sdk: flutter web_socket_channel: ^1.1.0
Once the library is added, we can import it into our Dart file.
import 'package:web_socket_channel/web_socket_channel.dart';
Now we can create a WebSocket connection. To do this, we need to instantiate a 'WebSocketChannel' object and pass the WebSocket server URL to the constructor.
final channel = WebSocketChannel.connect( Uri.parse('wss://my-websocket-server.com'), );
Once the connection is established, we can send and receive data using the 'channel' object. To send data, we use the 'sink.add' method.
channel.sink.add('Hello, WebSocket Server!');
To receive data, we use the 'stream.listen' method. This method receives a callback function that is called whenever new data is available.
channel.stream.listen((message) { print('Received: $message'); });
It's important to remember to close the WebSocket connection when we're done using it. This can be done using the 'sink.close' method.
channel.sink.close();
Overall, working with Websockets in Flutter and Dart is pretty straightforward. The 'web_socket_channel' library provides an easy-to-use API that allows us to establish real-time two-way communication with the server. This allows us to create applications that can instantly respond to events on the server, making our applications more responsive and enjoyable for users.
Now answer the exercise about the content:
What is the main difference between the traditional HTTP protocol and the Websockets protocol?
You are right! Congratulations, now go to the next page
You missed! Try again.
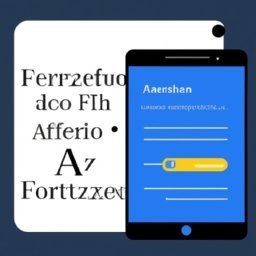
Next page of the Free Ebook: