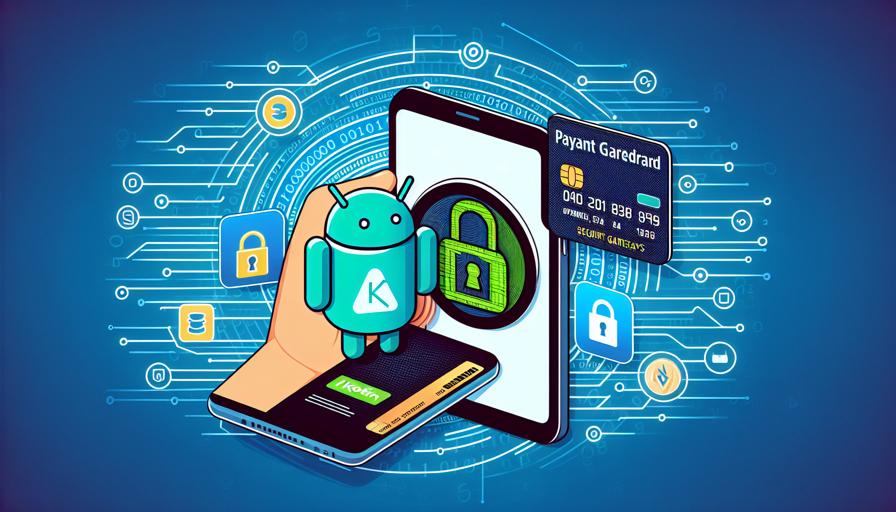
70. Integrating Payment Gateways in Android Apps
Page 101 | Listen in audio
Integrating payment gateways into Android apps is a crucial step for developers looking to monetize their applications or offer seamless in-app purchasing experiences. With the rise of e-commerce and digital payments, providing users with secure and efficient payment options can significantly enhance user satisfaction and drive revenue growth. This process involves several steps, from choosing the right payment gateway to implementing and testing it within your app. In this detailed guide, we will explore the intricacies of integrating payment gateways in Android apps using Kotlin, focusing on best practices and common challenges.
Understanding Payment Gateways
Payment gateways are services that facilitate online transactions by transferring information between a payment portal (such as a mobile app) and the acquiring bank. They ensure secure data transmission through encryption and fraud detection mechanisms. Popular payment gateways include PayPal, Stripe, Square, and Razorpay, each offering unique features and integration options.
Choosing the Right Payment Gateway
The first step in integrating a payment gateway is selecting the one that best suits your app’s requirements. Consider factors such as transaction fees, supported payment methods, geographic availability, currency support, and ease of integration. For instance, if your app targets a global audience, you might prefer a gateway with extensive international support like Stripe or PayPal.
Setting Up a Payment Gateway Account
Once you’ve chosen a payment gateway, the next step is to set up an account. This typically involves providing business details, verifying your identity, and setting up a merchant account. Each gateway has its own onboarding process, so refer to their documentation for specific instructions.
Integrating the Payment Gateway in Your Android App
With an account ready, you can begin integrating the payment gateway into your Android app. This process generally involves the following steps:
1. Add the SDK
Most payment gateways offer an SDK that simplifies integration. To add the SDK to your Kotlin-based Android project, you’ll typically need to include the necessary dependencies in your build.gradle
file. For example, to integrate Stripe, you would add:
dependencies {
implementation 'com.stripe:stripe-android:VERSION'
}
Replace VERSION
with the latest version number available.
2. Initialize the SDK
After adding the SDK, initialize it in your application. This often involves setting up configuration parameters such as API keys, which are unique identifiers provided by the payment gateway. For example, with Stripe, you would initialize it as follows:
Stripe.apiKey = "YOUR_PUBLISHABLE_KEY"
Ensure that you keep your API keys secure and never expose secret keys in your client-side code.
3. Design the Payment UI
Create an intuitive and user-friendly payment interface. This typically includes fields for entering payment details like card number, expiration date, and CVV. Many SDKs provide pre-built UI components to streamline this process. For instance, Stripe offers CardInputWidget
for collecting card information.
4. Handle Payment Processing
Once the user enters their payment details, you’ll need to handle the transaction process, which involves creating a payment intent or charge request. This is usually done by making an API call to the payment gateway’s server from your app’s backend, ensuring sensitive information is handled securely. Here’s a basic example using Stripe:
val paymentIntentParams = PaymentIntentParams
.createConfirmPaymentIntentWithPaymentMethodId(
paymentMethodId,
clientSecret
)
stripe.confirmPayment(this, paymentIntentParams)
The clientSecret
is obtained from your server after creating a payment intent.
5. Test the Integration
Testing is a critical phase to ensure the payment gateway works as expected. Use the gateway’s sandbox or test environment to simulate transactions without processing real payments. Test various scenarios, such as successful payments, declined transactions, and error handling, to ensure a robust integration.
Security Considerations
Security is paramount when dealing with payments. Follow best practices such as using HTTPS for all network communications, tokenizing payment information, and adhering to PCI DSS (Payment Card Industry Data Security Standard) guidelines. Additionally, leverage the security features provided by the payment gateway, such as fraud detection and 3D Secure authentication.
Handling Errors and Edge Cases
Prepare for potential errors and edge cases by implementing comprehensive error handling and user feedback mechanisms. Display clear messages for common issues like network errors, invalid payment details, or insufficient funds. This not only improves user experience but also helps in troubleshooting and support.
Maintaining and Updating the Integration
Payment gateways frequently update their APIs and SDKs to introduce new features, enhance security, or comply with regulatory changes. Regularly check for updates and maintain your integration to ensure continued functionality and security. Subscribe to the payment gateway’s developer updates or newsletters to stay informed.
Conclusion
Integrating a payment gateway into your Android app using Kotlin can significantly enhance its functionality and monetization potential. By carefully selecting the right gateway, following best practices for integration and security, and rigorously testing your implementation, you can provide users with a seamless and secure payment experience. As digital payments continue to evolve, staying updated with the latest trends and technologies will position your app for success in the competitive mobile landscape.
Now answer the exercise about the content:
What is the first step in integrating a payment gateway into an Android app according to the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
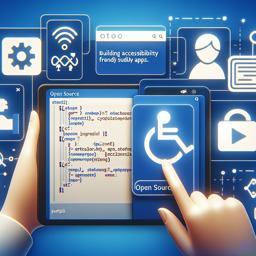
Next page of the Free Ebook: