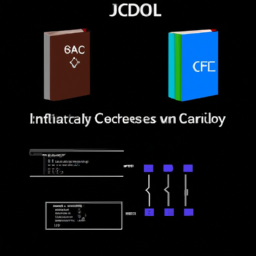
15. Functions and procedures in C#
Page 15 | Listen in audio
When we are developing games with Unity, the programming language we use is C#. Within C# programming, one of the fundamental concepts that we need to understand are functions and procedures. In this chapter of our e-book course, we will delve deeper into this topic.
Functions in C#
Functions in C#, also known as methods, are blocks of code that perform a specific task. They may or may not return a value. Functions help break code into smaller, more manageable segments, making it easier to read and maintain.
A function in C# is declared as follows:
public int Sum(int num1, int num2)
{
return num1 + num2;
}
In this example, 'public' is the access modifier that determines the visibility of the role. 'int' is the return type of the function, which specifies the type of value that the function returns. 'Sum' is the name of the function. 'int num1, int num2' are the function parameters, which are the values that the function receives when it is called.
Procedures in C#
Procedures in C# are a specific type of function that does not return a value. In other words, the return type of the procedure is 'void'. Just like functions, procedures also help break code into smaller, more manageable segments.
A procedure in C# is declared as follows:
public void DisplayMessage()
{
Console.WriteLine("Hello, World!");
}
In this example, 'public' is the access modifier, 'void' is the return type of the procedure, which indicates that the procedure does not return a value. 'DisplayMessage' is the name of the procedure. This procedure has no parameters.
Functions and Procedures Call
To use a function or procedure, we need to call that function or procedure. The function call is made by the function name followed by parentheses and any arguments the function may require.
Here is an example of how to call the 'Sum' function we declared earlier:
int result = Sum(5, 3);
And here is an example of how to call the 'ExibeMessage' procedure that we declared earlier:
DisplayMessage();
Conclusion
Functions and procedures are fundamental in C# programming, as they allow us to divide our code into smaller, more manageable blocks. This not only makes our code easier to read and maintain, but it also allows us to reuse the code, which can save a lot of time and effort.
We hope this chapter has given you a good understanding of functions and procedures in C#. In the next chapter, we'll delve deeper into other C# programming concepts important for game development with Unity.
Now answer the exercise about the content:
What is the main difference between functions and procedures in C# in the context of game development with Unity?
You are right! Congratulations, now go to the next page
You missed! Try again.
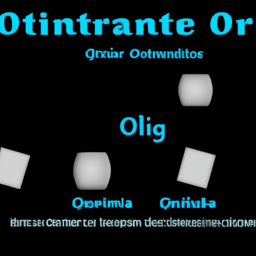
Next page of the Free Ebook: