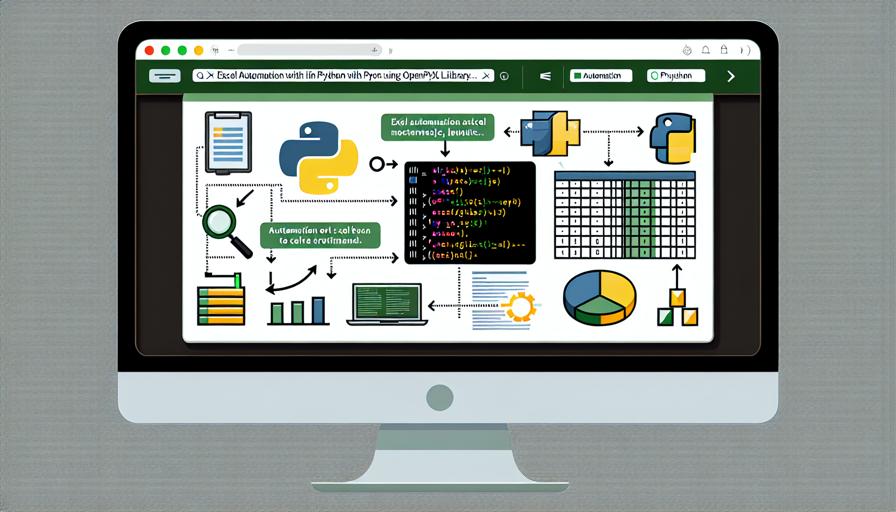
12.3. Excel Automation with Python: Using OpenPyXL for Excel Automation
Page 15 | Listen in audio
12.3. Excel Automation with Python: Using OpenPyXL for Excel Automation
In the realm of data management and analysis, Microsoft Excel remains a powerful and ubiquitous tool. However, manual data entry and manipulation can be time-consuming and error-prone. This is where Python, with its extensive libraries, steps in to automate and streamline these tasks. One such library is OpenPyXL, which provides a robust interface for interacting with Excel files in Python.
Introduction to OpenPyXL
OpenPyXL is a Python library for reading and writing Excel 2010 xlsx/xlsm/xltx/xltm files. It allows you to automate Excel tasks, such as creating new spreadsheets, updating existing ones, and even manipulating charts and images. OpenPyXL is particularly useful for automating repetitive tasks, such as data entry, data cleaning, and report generation.
Installing OpenPyXL
Before you can use OpenPyXL, you need to install it. You can easily install OpenPyXL using pip, the Python package manager. Run the following command in your terminal or command prompt:
pip install openpyxl
Basic Operations with OpenPyXL
Creating a New Workbook
To create a new Excel workbook, you first need to import the Workbook
class from OpenPyXL:
from openpyxl import Workbook
# Create a new workbook
wb = Workbook()
# Get the active worksheet
ws = wb.active
# Set the title of the worksheet
ws.title = "MySheet"
# Save the workbook
wb.save("my_workbook.xlsx")
This code snippet creates a new Excel file named my_workbook.xlsx
with a single worksheet titled "MySheet".
Loading an Existing Workbook
To load an existing workbook, you can use the load_workbook
function:
from openpyxl import load_workbook
# Load an existing workbook
wb = load_workbook("existing_workbook.xlsx")
# Select a sheet by name
ws = wb["Sheet1"]
This code loads an Excel file named existing_workbook.xlsx
and selects the worksheet named "Sheet1".
Reading and Writing Data
Reading and writing data to an Excel file is straightforward with OpenPyXL. You can access cells in a worksheet using the cell's row and column indices:
# Write data to a cell
ws['A1'] = "Hello, World!"
# Read data from a cell
print(ws['A1'].value)
This code writes the string "Hello, World!" to cell A1 and then reads it back.
Advanced Features of OpenPyXL
Working with Formulas
OpenPyXL supports Excel formulas, allowing you to automate complex calculations:
# Write a formula to a cell
ws['B1'] = "=SUM(A1:A10)"
# Save the workbook
wb.save("formulas.xlsx")
This code writes a SUM formula to cell B1, which calculates the sum of cells A1 through A10.
Styling Cells
OpenPyXL allows you to style cells to enhance the appearance of your Excel files. You can change fonts, colors, and borders:
from openpyxl.styles import Font, Color, Alignment
# Apply font style
ws['A1'].font = Font(name='Calibri', bold=True, color='00FF0000')
# Align text to the center
ws['A1'].alignment = Alignment(horizontal='center', vertical='center')
This code snippet sets the font of cell A1 to bold Calibri with a red color and centers the text both horizontally and vertically.
Adding Charts
Charts are an excellent way to visualize data, and OpenPyXL makes it easy to create and customize them:
from openpyxl.chart import BarChart, Reference
# Create a bar chart
chart = BarChart()
# Select data for the chart
data = Reference(ws, min_col=1, min_row=1, max_col=1, max_row=10)
# Add data to the chart
chart.add_data(data, titles_from_data=True)
# Add the chart to the worksheet
ws.add_chart(chart, "E5")
This code creates a bar chart using data from the first column (A1:A10) and places it at cell E5.
Practical Applications of Excel Automation
Excel automation with OpenPyXL can be applied in various domains, including finance, marketing, and data analysis. Here are a few practical examples:
- Financial Reports: Automate the generation of monthly or quarterly financial reports, including charts and summaries.
- Data Cleaning: Automatically clean and preprocess large datasets, removing duplicates and correcting errors.
- Inventory Management: Update inventory records and generate restocking alerts based on predefined thresholds.
Conclusion
OpenPyXL offers a powerful and flexible way to automate Excel tasks using Python. By leveraging this library, you can save time, reduce errors, and focus on more strategic activities. Whether you're generating reports, cleaning data, or creating complex spreadsheets, OpenPyXL provides the tools you need to enhance your productivity and efficiency.
Incorporating Excel automation into your workflow not only streamlines processes but also opens up new possibilities for data analysis and visualization. As you become more familiar with OpenPyXL, you'll find that the potential applications are limited only by your imagination.
Now answer the exercise about the content:
What Python library can be used to automate Excel tasks such as creating new spreadsheets and manipulating charts and images?
You are right! Congratulations, now go to the next page
You missed! Try again.
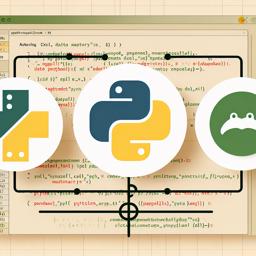
Next page of the Free Ebook: