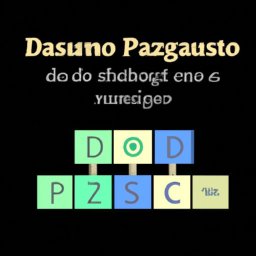
5.11. Data Structures in Python: Search
Página 25
Data structures in Python: Search
Python is a powerful and versatile programming language that offers a variety of built-in data structures. A solid understanding of these frameworks is crucial for any Python programmer, especially when building complex systems with Python and Django. In this chapter, we're going to focus on a fundamental aspect of Python data structures: searching.
Introduction to Quest
Searching is a fundamental operation that involves finding a specific element in a data structure. Python offers several efficient ways to perform searches, depending on the specific data structure you are using. Let's explore some of the more common data structures and how to perform searches on them.
Search in Lists
A list in Python is an ordered data structure that can contain any number of items, and those items can be of any type. The simplest way to fetch an item from a list is using the 'in' operator.
list = [1, 2, 3, 4, 5] if 3 in list: print("The number 3 is in the list") else: print("The number 3 is not in the list")
This search operation has a time complexity of O(n), where n is the number of elements in the list. This means that, in the worst case, Python will have to check every element in the list to find what we're looking for.
Search in Sets
A set in Python is an unordered data structure that stores single elements. Searching a set is significantly faster than searching a list, with an average time complexity of O(1).
set = {1, 2, 3, 4, 5} if 3 in set: print("The number 3 is in the set") else: print("The number 3 is not in the set")
This efficiency is due to the way sets are implemented in Python. They use a hash table, which allows Python to quickly find an element regardless of the size of the set.
Search in Dictionaries
A Python dictionary is a data structure that stores key-value pairs. A dictionary lookup is similar to a set lookup in that it also uses a hash table for efficiency. However, we are looking for a key instead of a value.
dictionary = {'one': 1, 'two': 2, 'three': 3, 'four': 4, 'five': 5} if 'three' in dictionary: print("The key 'three' is in the dictionary") else: print("The key 'three' is not in the dictionary")
Dictionary lookup also has an average time complexity of O(1), making it an efficient choice for large amounts of data.
Binary Search
For ordered lists, Python offers an even more efficient way to search for an element: binary search. This is a divide-and-conquer strategy that cuts the number of necessary comparisons in half at every step.
import bisect ordered_list = [1, 2, 3, 4, 5] index = bisect.bisect_left(ordered_list, 3) if index != len(sorted_list) and sorted_list[index] == 3: print("The number 3 is in the list") else: print("The number 3 is not in the list")
Binary search has a time complexity of O(log n), making it extremely efficient for large lists.
Conclusion
Understanding how to perform efficient searches on different data structures is an essential skill for any Python programmer. Each data structure has its own advantages and disadvantages in terms of search efficiency, so it's important to choose the right data structure for the job at hand. As we continue to explore building systems with Python and Django, we'll see how these data structures and query techniques can be applied to solve complex problems.
Now answer the exercise about the content:
What is the time complexity of the fetch operation on different data structures in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
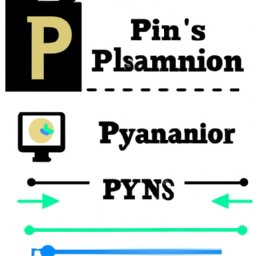
Next page of the Free Ebook: