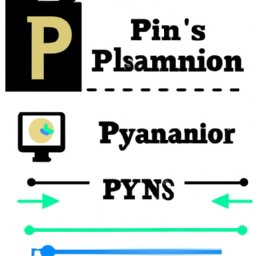
6. Functions in Python
Página 26
Python functions are reusable blocks of code that perform a specific task. They are a fundamental aspect of Python programming and are used to improve code modularity and code reuse. When creating a course on building systems with Python and Django, it's important to understand how functions in Python work and how they can be used to create efficient and effective systems.
Function Definition
A function in Python is defined using the 'def' keyword, followed by the function name and parentheses (). Within these parentheses, you can include any parameters that the function must accept. You then use a colon (:) to indicate the beginning of the block of code that forms the body of the function. For example:
def my_function(): print('Hello world!')
In this example, 'my_function' is the name of the function and 'print('Hello world!')' is the body of the function. When this function is called somewhere in the code, it will print the string 'Hello world!'.
Calling Functions
To call a function in Python, you simply use the name of the function followed by parentheses (). If the function accepts parameters, you would enclose them inside the parentheses. For example:
my function()
This would call the 'my_function' function we defined earlier and print 'Hello world!'.
Parameters and Arguments
Python functions can accept parameters, which are values that you can pass to the function when you call it. Parameters are defined in parentheses in the function definition and are separated by commas. For example:
def my_function(name): print('Hello, ' + name + '!')
In this example, the function 'my_function' accepts a parameter called 'name'. When we call this function, we need to provide an argument for this parameter:
my_function('World')
This would print 'Hello World!'.
Functions with Returns
Functions in Python can also return a value. This is done using the 'return' keyword, followed by the value or expression to be returned. For example:
def my_function(x): return x * x
In this example, the function 'my_function' accepts a parameter 'x' and returns the square of that number. If we call this function with the number 5 as an argument, it will return 25:
print(my_function(5)) # Prints: 25
Recursive Functions
Python also supports recursive functions, which are functions that call themselves. This can be useful for solving problems that can be broken down into smaller problems of a similar nature. For example, the factorial function is commonly implemented recursively in Python:
def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1)
In this example, the 'factorial' function calls itself to calculate the factorial of a number. The recursion ends when the number is 1, in which case the function returns 1.
In short, functions are an essential part of programming in Python. They allow you to organize your code in a logical and reusable way, improving the efficiency and readability of your code. When creating a course on building systems with Python and Django, it's important to cover functions in depth, as they are a powerful tool for building effective systems.
Now answer the exercise about the content:
What is the use of functions in Python?
You are right! Congratulations, now go to the next page
You missed! Try again.
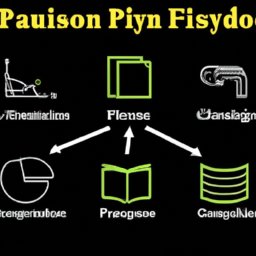
Next page of the Free Ebook: