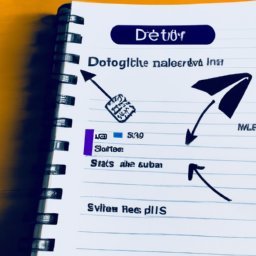
31.10. Data Persistence with SQLite in Flutter: Data Migration
Página 264
Data Persistence with SQLite in Flutter: Data Migration
A crucial part of application development is data management. In this course chapter, we are going to explore how to implement data persistence using SQLite in Flutter. SQLite is an embedded SQL database library in C that provides device-local storage for your application. We'll also cover an important aspect of data management: data migration.
SQLite in Flutter
SQLite is an embedded database that is very easy to use. It does not require a separate server process and allows accessing the database using a non-standard role-based interface. To use SQLite in Flutter, we need to add the 'sqflite' dependency in our pubspec.yaml file.
dependencies: flutter: sdk: flutter sqflite: ^1.3.0
After adding the dependency, we can import the sqflite package into our Dart file.
import 'package:sqflite/sqflite.dart';
Creating Database and Tables
To create a database, we use the openDatabase function and pass the database path as an argument. If the database does not exist, it will be created. We can also set the database version and implement the onCreate function to create tables.
Database database = await openDatabase( path, version: 1, onCreate: (Database db, int version) async { await db.execute('CREATE TABLE my_table (id INTEGER PRIMARY KEY, name TEXT)'); }, );
Data Migration
Data migration is a vital process to maintain data integrity when updating the database structure. When we change the structure of the database, like adding a new column or changing the data type of a column, we need to migrate the existing data to the new structure.
In SQLite, we can use the onUpgrade function to handle data migration. The onUpgrade function will be called when the current database version is higher than the previous version. Within this function, we can execute SQL commands to change the structure of the database.
Database database = await openDatabase( path, version: 2, onUpgrade: (Database db, int oldVersion, int newVersion) async { if (oldVersion < 2) { await db.execute('ALTER TABLE my_table ADD COLUMN age INTEGER'); } }, );
Conclusion
SQLite is an excellent option for storing data locally in a Flutter application. It offers an easy-to-use interface to manage data and supports data migration to maintain data integrity. By using SQLite in Flutter, we can build more robust and reliable applications.
This chapter covered the basics of data persistence with SQLite in Flutter and data migration. However, there is much more to learn about SQLite, such as transactions, complex queries, and performance optimization. We hope this chapter has provided a solid foundation for exploring more about SQLite in Flutter.
Now answer the exercise about the content:
What is SQLite in Flutter and how is it used?
You are right! Congratulations, now go to the next page
You missed! Try again.
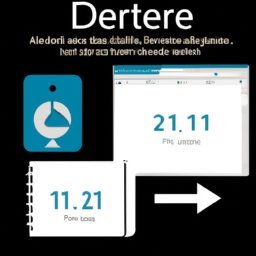
Next page of the Free Ebook: