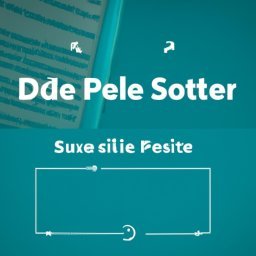
31.2. Data persistence with SQLite in Flutter: Configuration and installation of the SQLite plugin
Página 256
Data persistence is a crucial component of any application. In Flutter app development, SQLite is one of the most popular options for persisting data. SQLite is an embedded relational database, which means it is a C library that provides a lightweight database on disk that does not require a separate server process and allows access to the database using a non- SQL standard. Plus, SQLite is free and open source.
To start using SQLite in your Flutter app, you need to configure and install the SQLite plugin. This process involves several steps that will be discussed in detail in this chapter.
1. Adding SQLite plugin dependency
First, you need to add the SQLite plugin dependency in your Flutter project's pubspec.yaml file. This file is where you list all of your project's dependencies. Add the following line in the dependencies section:
dependencies: sqflite: ^1.3.0+2
After adding the dependency, run the "flutter pub get" command in the terminal to download and install the SQLite plugin.
2. Importing the SQLite plugin
After installing the plugin, you need to import it into your code to be able to use it. You can do this by adding the following line at the beginning of your Dart file:
import 'package:sqflite/sqflite.dart';
3. Creating the SQLite Database
With the SQLite plugin imported, you can now create your SQLite database. First, you need to define the path to the database. You can do this using the SQLite plugin's getDatabasesPath() method, which returns the path to the directory where the application can store databases. You can then open the database using the openDatabase() method and passing the database path as an argument.
var databasesPath = await getDatabasesPath(); String path = join(databasesPath, 'my_database.db'); // open the database Database database = await openDatabase(path, version: 1);
4. Creating tables
With the database open, you can now create tables. You can do this using the execute() method of the Database object and passing in an SQL string that defines the table. For example, to create a table called "Tasks" with columns for "id", "title" and "description", you could do the following:
await database.execute( 'CREATE TABLE Tasks(id INTEGER PRIMARY KEY, title TEXT, description TEXT)' );
5. Entering data
To insert data into the table, you can use the insert() method of the Database object. This method accepts the table name and a Map containing the data to be inserted. For example, to insert a new task in the "Tasks" table, you can do the following:
int id = await database.insert( 'Tasks', {'title': 'First Task', 'description': 'This is the first task'}, );
This is just a basic start on how to use SQLite in a Flutter app. There's so much more you can do, like updating and deleting data, running complex queries, and much more. However, with this start you should be able to start using SQLite in your Flutter app.
Data persistence is a crucial part of many applications, and with SQLite and Flutter, you have a powerful and flexible solution for managing and storing your data. We hope this chapter has provided a helpful introduction to this important topic.
Now answer the exercise about the content:
What is the process to start using SQLite in a Flutter app?
You are right! Congratulations, now go to the next page
You missed! Try again.
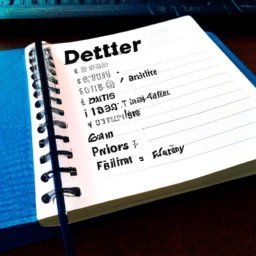
Next page of the Free Ebook: