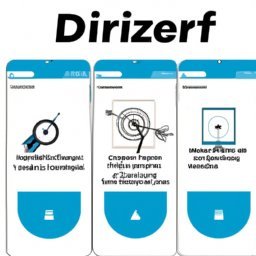
3.5. Dart Basics: Functions
Page 23 | Listen in audio
Dart is a modern, object-oriented programming language developed by Google that is used to create mobile, web, and desktop applications. One of the key features of Dart is that it supports first-class functions, which means that functions can be passed as arguments to other functions, assigned to variables, or embedded in data structures. In this article, we'll explore the basics of functions in Dart.
Defining Dart Functions
In Dart, a function is defined using the keyword 'void' followed by the function name, parentheses, and a block of code enclosed in braces. For example:
void main() { print('Hello World!'); }
This is the 'main' function, which is the entry point for all Dart applications. When the program is executed, the 'main' function is called first.
Functions with Parameters
Functions in Dart can have parameters, which are values that you can pass to the function when you call it. Parameters are defined in parentheses after the function name. For example:
void greeting(String name) { print('Hello, $name!'); }
To call this function, you would pass a value for the 'name' parameter:
greeting('Mary');
Functions with Return Values
Functions can also return values. To do this, replace the 'void' keyword with the type of value the function will return. For example, the following function returns an integer:
int sum(int a, int b) { return a + b; }
To use the value returned by this function, you can assign it to a variable:
int result = sum(5, 3);
Anonymous Functions
Dart also supports anonymous functions, also known as lambda functions or arrow functions. These are functions that have no name and are generally used for short-term operations. For example:
var list = ['apple', 'banana', 'orange']; list.forEach((item) { print(item); });
In this example, the anonymous function is passed as an argument to the 'forEach' method of the list. The function is called once for each item in the list.
High Order Functions
As mentioned earlier, Dart supports first-class functions, which means that functions can be passed as arguments to other functions. These are called higher-order functions. For example:
void executeFunction(Function function) { function(); } void sayHello() { print('Hello!'); } void main() { executeFunction(sayHi); }
In this example, the 'dizHello' function is passed as an argument to the 'executaFuncao' function, which then calls the 'dizHello' function.
In short, functions are a fundamental part of Dart programming. They let you organize your code into reusable blocks and handle data efficiently. Mastering the use of functions in Dart is essential for building complex applications with Flutter.
Now answer the exercise about the content:
Which of the following statements is true about the Dart programming language?
You are right! Congratulations, now go to the next page
You missed! Try again.
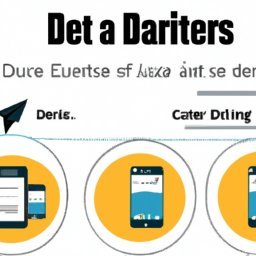
Next page of the Free Ebook: