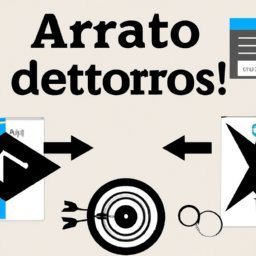
3.10. Dart Basics: Asynchronous Programming
Page 28 | Listen in audio
Asynchronous programming is a fundamental concept in Dart, especially when it comes to building apps with Flutter. Before diving into the details, let's understand what asynchronous programming is and why it's so important.
Programs normally run synchronously, which means that each statement is executed one after the other. However, some processes may take longer to complete, such as network requests, file reading, etc. During that time, the program is forced to wait, which can lead to a poor user experience, especially in mobile apps where fluidity and responsiveness are crucial.
Asynchronous programming solves this problem by allowing the program to continue performing other tasks while waiting for the lengthy operation to complete. This is done through 'Future' and 'async / await', two key concepts in asynchronous Dart programming.
Future
In Dart, a 'Future' represents a potential value or error that will be available at some point in the future. It is similar to 'Promise' in JavaScript. When you start an async operation, it returns a 'Future'. This 'Future' will be 'completed' when the operation completes or 'rejected' if an error occurs.
To handle the result of a 'Future', you can use the 'then()' method to specify what should be done when the 'Future' completes successfully, and the 'catchError()' method to handle any errors. Also, you can chain together multiple 'then()' and 'catchError()' calls, each of which returns a new 'Future'.
Async / Await
Although 'Future' and its 'then()' and 'catchError()' methods are powerful, they can lead to very nested and difficult to read code. This is where 'async' and 'await' come into play.
The 'async' keyword is used to declare an asynchronous function. Within an 'async' function, you can use the 'await' keyword before an expression to pause the execution of the function until the expression completes. The expression is usually a call to a function that returns a 'Future'.
The use of 'async' and 'await' results in cleaner and easier to understand code, as it allows you to write asynchronous code as if it were synchronous.
Example
Suppose you have a 'fetchData()' function that returns a 'Future'. Here's how you can use 'Future', 'async' and 'await' to handle this:
Future<String> fetchData() { // Simulates a network operation that takes 2 seconds return Future.delayed(Duration(seconds: 2), () => 'Hello, World!'); } void main() async { print('Fetching data...'); String data = await fetchData(); print('Date: $data'); }
When you run this code, it prints 'Fetching data...', waits for 2 seconds and then prints 'Data: Hello, World!'. During these 2 seconds, the program is not blocked, allowing other tasks to be executed.
In summary, asynchronous programming is a crucial concept in Dart that allows you to write responsive and fluid applications. By using 'Future' and 'async / await', you can perform time-consuming operations such as network requests without blocking the program, providing a better user experience.
Now answer the exercise about the content:
What does asynchronous programming enable in Dart and why is it important?
You are right! Congratulations, now go to the next page
You missed! Try again.
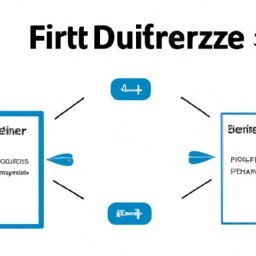
Next page of the Free Ebook: