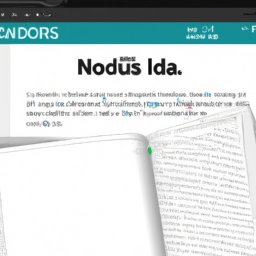
16.6. Creating a CRUD with NodeJS and MongoDB: Creating routes for the CRUD
Página 90
This chapter is dedicated to one of the most important parts of API development: creating a CRUD (Create, Read, Update, Delete) with NodeJS and MongoDB. We will create routes for CRUD operations and learn how to interact with the MongoDB database.
First, let's understand what CRUD is. CRUD is an acronym for Create, Read, Update, and Delete. These are the four basic operations a system must have to manage data. In our API, we are going to create routes to perform these operations on our MongoDB database.
To get started, we need a NodeJS development environment set up and a running MongoDB instance. If you don't already have this set up, refer to the previous chapters of this eBook.
1. Configuring Express and Mongoose
First, let's configure Express, which is a framework for NodeJS that helps us manage HTTP routes and requests. Also, we are going to use Mongoose, which is a NodeJS library for MongoDB that allows us to work with the database in an easier and safer way.
To install these dependencies, run the following command in the terminal:
npm install express mongoose
After installing these dependencies, let's configure them in our main NodeJS file. Let's create an instance of express and connect to MongoDB using Mongoose.
const express = require('express');
const mongoose = require('mongoose');
const app = express();
mongoose.connect('mongodb://localhost:27017/myapp', {useNewUrlParser: true, useUnifiedTopology: true});
2. Creating the MongoDB Model
Before creating the routes, we need to define the data model that we are going to use in MongoDB. To do this, we're going to use Mongoose to create a data schema.
const UserSchema = new mongoose.Schema({
name: String,
email: String,
password: String
});
const User = mongoose.model('User', UserSchema);
3. Creating routes for CRUD
Now that we have the data model, we can create the routes for the CRUD operations. Let's create a route for each operation: create, read, update and delete.
app.post('/users', async (req, res) => {
const user = new User(req.body);
await user.save();
res.send(user);
});
app.get('/users', async (req, res) => {
const users = await User.find();
res.send(users);
});
app.get('/users/:id', async (req, res) => {
const user = await User.findById(req.params.id);
res.send(user);
});
app.put('/users/:id', async (req, res) => {
const user = await User.findByIdAndUpdate(req.params.id, req.body);
res.send(user);
});
app.delete('/users/:id', async (req, res) => {
await User.findByIdAndDelete(req.params.id);
res.send('User deleted');
});
These routes allow us to create, read, update, and delete users in our MongoDB database. Note that we are using asynchronous operations (async/await) to handle the database operations as they may take some time to complete.
4. Testing routes
To test the routes, we can use a tool like Postman or Insomnia. Just make a request to the corresponding HTTP route and method and check if the operation was successful.
With that, we finish creating a CRUD with NodeJS and MongoDB. This is a fundamental step for creating APIs, as it allows us to manage data efficiently and securely. Keep following the next chapters to learn more about developing APIs with NodeJS.
Now answer the exercise about the content:
What is CRUD in the context of developing APIs with NodeJS and MongoDB?
You are right! Congratulations, now go to the next page
You missed! Try again.
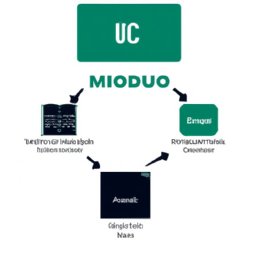
Next page of the Free Ebook: