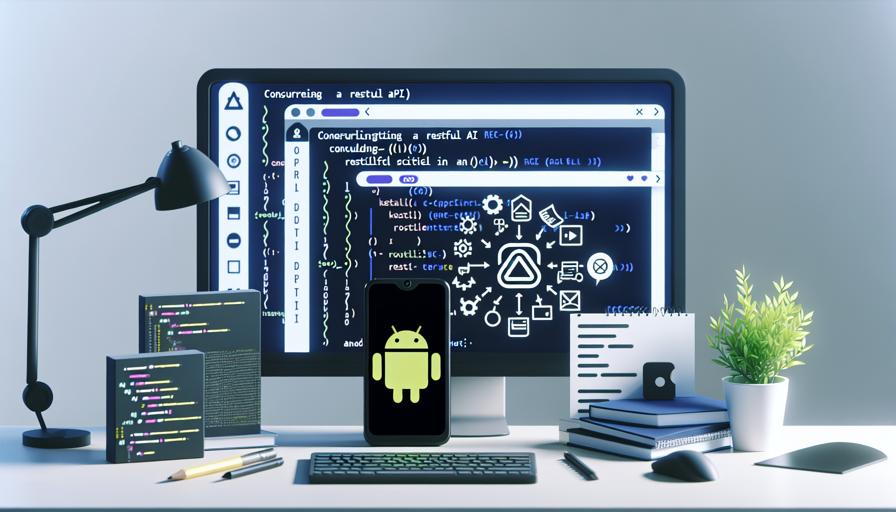
33. Consuming RESTful API in Android
Page 51 | Listen in audio
In the world of Android app development, interacting with RESTful APIs is a fundamental skill that every developer needs to master. RESTful APIs allow mobile applications to communicate with servers over the web, enabling them to fetch and send data, which is crucial for dynamic and data-driven applications. In this section, we will explore how to consume RESTful APIs in Android using Kotlin, covering everything from making network requests to parsing JSON responses.
Understanding RESTful APIs
REST, which stands for Representational State Transfer, is an architectural style for designing networked applications. RESTful APIs use HTTP requests to perform CRUD (Create, Read, Update, Delete) operations on resources. These resources are often represented in JSON format, which is lightweight and easy to parse in Android applications.
Setting Up Your Android Project
Before we dive into consuming RESTful APIs, ensure that your Android project is set up with the necessary dependencies. We will use Retrofit, a popular HTTP client for Android and Java, which makes networking easier by providing a type-safe way to consume RESTful APIs.
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
implementation 'com.squareup.okhttp3:logging-interceptor:4.9.1'
These dependencies include Retrofit and a Gson converter for JSON parsing. The logging interceptor is optional but helpful for debugging network requests.
Creating a Retrofit Instance
To start making network requests, we first need to create a Retrofit instance. This instance will be responsible for configuring the base URL and the converters used to parse the API responses.
val retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build()
Replace https://api.example.com/
with the base URL of the API you are consuming. The GsonConverterFactory
is used here to automatically convert JSON responses into Kotlin data objects.
Defining API Endpoints
Next, define the API endpoints using interfaces. Retrofit uses annotations to describe the HTTP requests:
interface ApiService {
@GET("users")
suspend fun getUsers(): List<User>
@POST("users")
suspend fun createUser(@Body user: User): Response<User>
}
In this example, we define two endpoints: one for getting a list of users and another for creating a new user. The @GET
and @POST
annotations specify the HTTP methods, and the @Body
annotation is used to pass the request body.
Creating Data Models
To handle the JSON responses, create data models that match the structure of the JSON data. For example, if the API returns a list of users:
data class User(
val id: Int,
val name: String,
val email: String
)
This User
data class represents a user object with an ID, name, and email. Retrofit will use Gson to map JSON data to this class automatically.
Making Network Requests
With the Retrofit instance and API interface set up, you can now make network requests. In a Kotlin coroutine scope, you can call the API methods:
val apiService = retrofit.create(ApiService::class.java)
GlobalScope.launch(Dispatchers.IO) {
try {
val users = apiService.getUsers()
withContext(Dispatchers.Main) {
// Update UI with the list of users
}
} catch (e: Exception) {
e.printStackTrace()
// Handle error
}
}
This example uses GlobalScope.launch
to perform the network request in a background thread. The withContext(Dispatchers.Main)
block is used to update the UI on the main thread after fetching the data.
Error Handling
Handling errors is crucial when working with network requests. You can catch exceptions to handle network errors, such as connectivity issues or server errors:
try {
val response = apiService.createUser(newUser)
if (response.isSuccessful) {
// Handle successful response
} else {
// Handle error response
}
} catch (e: IOException) {
// Handle network error
}
In this example, we check if the response is successful before processing it. If not, you can access the error body or status code to determine the cause of the failure.
Using OkHttp Interceptors
OkHttp interceptors can be used to modify requests and responses, add headers, or log network activity. For example, to add a logging interceptor:
val loggingInterceptor = HttpLoggingInterceptor().apply {
level = HttpLoggingInterceptor.Level.BODY
}
val okHttpClient = OkHttpClient.Builder()
.addInterceptor(loggingInterceptor)
.build()
val retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com/")
.client(okHttpClient)
.addConverterFactory(GsonConverterFactory.create())
.build()
This setup will log the details of each network request and response, which is useful for debugging.
Conclusion
Consuming RESTful APIs in Android using Kotlin and Retrofit is a straightforward process once you understand the components involved. By setting up a Retrofit instance, defining API endpoints, creating data models, and handling network requests and errors, you can effectively integrate web services into your Android applications. As you become more familiar with these concepts, you'll be able to build more complex and feature-rich apps that leverage the power of RESTful APIs.
Now answer the exercise about the content:
What is the purpose of using Retrofit in Android app development according to the text?
You are right! Congratulations, now go to the next page
You missed! Try again.
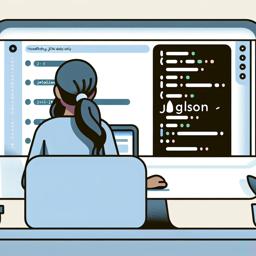
Next page of the Free Ebook: