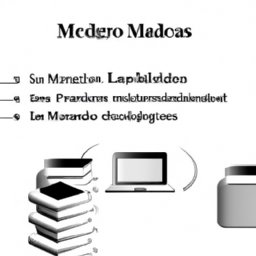
17.12. Classes and Objects: Method Overloading
Página 56
In object-oriented programming, classes are essential for creating objects. They are like a blueprint or blueprint that defines the characteristics (attributes) and behaviors (methods) that an object can have. Method overloading is a feature that allows a class to have more than one method with the same name but different parameter lists.
Before we dive into method overloading, it's important to understand what methods are. In simple terms, methods are functions defined within a class. They are used to perform operations on the attributes of our objects. Methods are a way to add behavior to our objects. The syntax for creating a method is similar to that of a function.
Now, let's discuss method overloading. Method overloading is a concept in object-oriented programming where a class can have multiple methods with the same name but different parameters. For example, consider a class called 'Calculator' that can perform various mathematical operations like addition, subtraction, multiplication and division. This class can have separate methods for adding two integers, adding three integers, adding two floating point numbers, etc. All these methods would have the same name 'add', but would have different parameters.
class Calculator { int add(int a, int b) { return a + b; } int add(int a, int b, int c) { return a + b + c; } float add(float a, float b) { return a + b; } }
In method overloading, the compiler determines which method to use based on the given parameters. If you call the 'add' method with two integers, the compiler will use the first method. If you call the 'add' method with three integers, it will use the second method. And if you call the 'add' method with two floating point numbers, it will use the third method.
It is important to note that method overhead is not determined by the return type of the method. Two methods with the same name but different return types are not considered method overloads. Furthermore, method overloading is not limited to just instance methods - you can also overload static methods.
Method overloading is useful for several reasons. First, it improves code readability. Instead of having different method names for similar operations (like add_int, add_float, etc.), you can simply have a single method name 'add'. Second, it also allows you to define different behaviors for different types of data.
In summary, method overloading is a powerful feature in object-oriented programming that allows a class to have multiple methods with the same name but different parameter lists. This improves code readability and allows you to define different behaviors for different data types.
As a beginner in programming logic, it is important to understand and apply this concept correctly. Practice remains the best way to learn and improve your skill in method overload. So try to implement this concept in your programming projects and see the difference it can make in the structure and readability of your code.
Now answer the exercise about the content:
What is method overloading in object-oriented programming?
You are right! Congratulations, now go to the next page
You missed! Try again.
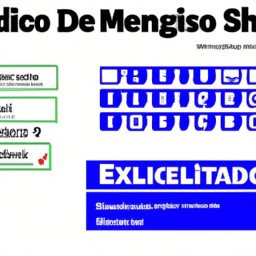
Next page of the Free Ebook: