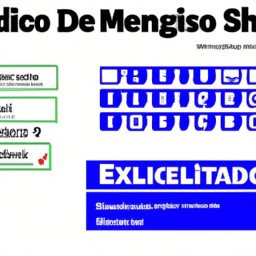
17.13. Classes and Objects: Overriding Methods
Página 57
17.13. Classes and Objects: Method Override
Object-oriented programming is a programming paradigm that uses abstraction to create models based on the real world. It uses several previous programming techniques, including inheritance, polymorphism, and encapsulation. In this chapter, we're going to focus on an important concept in object-oriented programming: method overriding.
What is method overriding?
Method overriding is a feature that allows a subclass to provide a specific implementation of a method that is already provided by one of its superclasses. When a method in a subclass has the same name, the same parameters or signature, and the same return type as a method in its superclass, then the method in the subclass is said to be an override of the method in the superclass.
It is used to achieve polymorphism in object-oriented programming. Overriding methods allows an inheriting class to change the behavior of methods inherited from the parent class. This is done to implement a specific behavior of the child class.
How does method override work?
When a method is invoked, the Java virtual machine (JVM) decides which version of the method to execute based on the type of object being referenced, not the type of reference. This means that if a superclass contains a method that has already been overridden by the subclass, then when the method is called, the version of the method in the subclass will be executed.
Method override example
Consider an example where we have a superclass called Animal and a subclass called Dog. The Animal class has a method called sound(), which is overridden by the Dog class.
class Animal { void sound() { System.out.println("The animal makes a sound"); } } class Dog extends Animal { void sound() { System.out.println("The dog barks"); } }
In the example above, the Dog class overrides the sound() method of the Animal class. So, if we create an object of the Dog class and call the sound() method, the version of the method in the Dog class will be executed instead of the version in the Animal class.
Rules for overriding methods
There are some rules that we must follow when overriding methods:
- The method in the subclass must have the same name, the same parameters, and the same return type as the method in the superclass.
- The method in the subclass must be at least as accessible as the method in the superclass.
- The method in the subclass cannot throw more checked exceptions than the method in the superclass.
- If the method in the superclass is marked as final, then it cannot be overridden.
Understanding method overriding is fundamental to understanding how object-oriented programming works, and it is a concept that is used frequently in practice. It allows subclasses to behave differently from their superclasses, which can be very useful in many situations.
Method overriding is one of many techniques that make object-oriented programming a powerful and flexible tool. Understanding this concept is an important step in becoming an effective Java programmer.
Now answer the exercise about the content:
What is method overriding in object-oriented programming and how does it work?
You are right! Congratulations, now go to the next page
You missed! Try again.
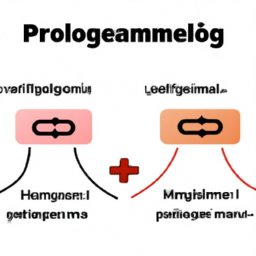
Next page of the Free Ebook: