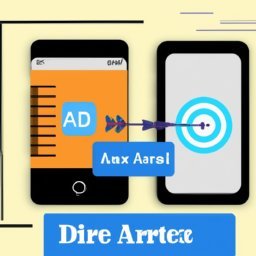
4.12. Advanced Dart Concepts: Integration with APIs
Página 50
To create advanced applications with Flutter and Dart, it is essential to understand how to integrate with APIs. APIs, or Application Programming Interfaces, are sets of rules and protocols that allow communication between different software. In other words, an API allows your application to interact with a server or database to retrieve, send, or update data.
When it comes to Dart, the programming language used in Flutter, there are several libraries and resources available to facilitate integration with APIs. This article will explore some of these advanced concepts, including how to make HTTP requests, decode JSON, handle bugs and more.
Making HTTP requests with Dart
To interact with an API, you will usually need to make an HTTP request. Dart provides a package called 'http' that makes this easy. First, you'll need to add the package to your 'pubspec.yaml' file and import it into your Dart file.
dependencies: http: ^0.13.3
You can then use the 'http.get()' function to make a GET request to a specific URL. The function returns a 'Future', which is an object representing a potential value or error that will become available at some point in the future. You can use the 'then()' method to handle the value once it is available, or the 'catchError()' method to handle any errors that may occur.
http.get('https://api.example.com/data') .then((response) { print(response.body); }) .catchError((error) { print(error); });
Decoding JSON with Dart
Many APIs return data in JSON format, which is a lightweight, easy-to-read way to represent structured data. Dart provides a package called 'dart:convert' which includes a 'jsonDecode()' function to convert a JSON string into a Dart object.
import 'dart:convert'; void main() { String jsonString = '{"name":"John", "age":30, "city":"New York"}'; Mapuser = jsonDecode(jsonString); print('Name: ${user['name']}'); print('Age: ${user['age']}'); print('City: ${user['city']}'); }
This example decodes a JSON string into a Map Dart, which is a collection of key-value pairs. You can then access the values using square bracket syntax with the corresponding brace.
Handling errors with Dart
When you are working with APIs, many things can go wrong. The HTTP request might fail due to a network issue, the response might not be valid JSON, or the API might return an error. It is important to handle these errors properly to prevent your application from crashing or behaving unexpectedly.
Dart provides several ways to handle errors, including 'try/catch', 'on', and 'finally' blocks. A 'try/catch' block allows you to "try" to run some code, and if an error occurs, "catch" the error and do something with it.
try { var response = await http.get('https://api.example.com/data'); var data = jsonDecode(response.body); } catch (e) { print('An error occurred: $e'); }
In this example, if the HTTP request fails or the response is not a valid JSON, Dart will throw an error. The 'catch' block will catch this error and print an error message.
Conclusion
Integration with APIs is a crucial part of developing advanced applications with Flutter and Dart. By understanding how to make HTTP requests, decode JSON, and handle errors, you can build applications that interact with servers, databases, and other APIs to provide a rich, dynamic experience for users.
Now answer the exercise about the content:
What is an API and what is its importance in app development with Flutter and Dart?
You are right! Congratulations, now go to the next page
You missed! Try again.
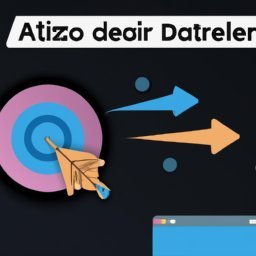
Next page of the Free Ebook: