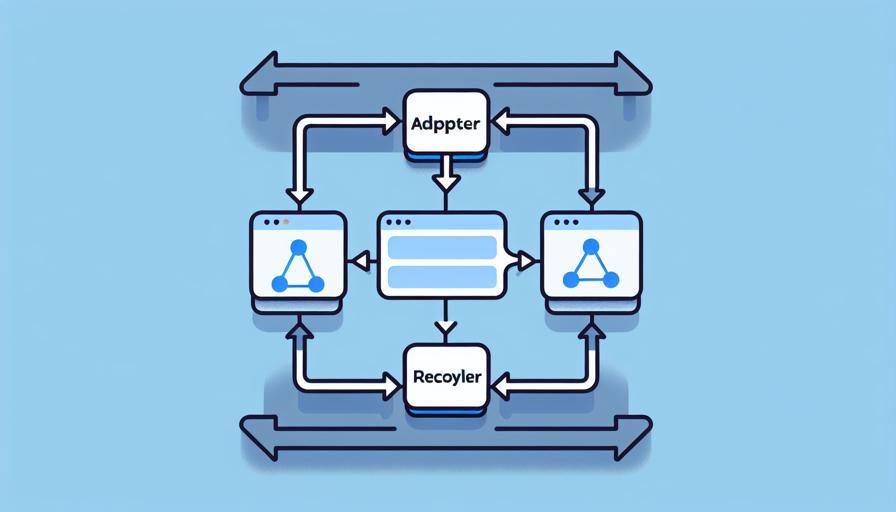
23. Adapters and RecyclerViews
Page 23 | Listen in audio
In Android app development, the ability to efficiently display large sets of data is crucial. Enter the RecyclerView, a more advanced and flexible version of the ListView. It is designed to be the most powerful and flexible component for displaying lists of data in Android applications. When combined with an Adapter, it allows developers to bind data efficiently to UI components.
The RecyclerView widget is a part of the androidx.recyclerview.widget package and is a descendant of the ViewGroup class. Unlike the ListView, the RecyclerView is not limited to a vertical list. It can be configured to display data in various layouts such as grids, horizontal lists, and more, thanks to its powerful layout managers.
Understanding Adapters
An Adapter in Android acts as a bridge between the UI component and the data source that fills data into the UI component. In the context of RecyclerViews, the Adapter is responsible for providing views that represent items in a data set. It creates ViewHolder objects, binds data to them, and returns them to the RecyclerView to display.
To implement an Adapter, you need to extend the RecyclerView.Adapter
class. This requires implementing the following key methods:
onCreateViewHolder(ViewGroup parent, int viewType)
: This method is called when the RecyclerView needs a new ViewHolder to represent an item. It is responsible for inflating the item layout and creating the ViewHolder.onBindViewHolder(ViewHolder holder, int position)
: Here, you bind the data to the ViewHolder. This involves setting the data for the item at the given position.getItemCount()
: This method returns the total number of items in the data set held by the adapter.
ViewHolder Pattern
The ViewHolder pattern is a design pattern used to improve the performance of list views by reducing the number of times that findViewById needs to be called. In RecyclerView, the ViewHolder pattern is built-in and mandatory. The ViewHolder is a static inner class in the Adapter that holds references to the views for each data item. This pattern helps in recycling views, hence the name RecyclerView.
Layout Managers
The LayoutManager is a crucial component of the RecyclerView. It is responsible for measuring and positioning item views within a RecyclerView and also for determining the policy for when to recycle item views that are no longer visible. RecyclerView comes with several built-in LayoutManagers:
- LinearLayoutManager: Displays items in a vertical or horizontal scrolling list.
- GridLayoutManager: Displays items in a grid format, similar to a grid of images.
- StaggeredGridLayoutManager: Similar to GridLayoutManager but allows for items with varying sizes.
Each LayoutManager can be customized further to suit specific needs, including creating custom LayoutManagers if the default ones do not meet your requirements.
Setting Up a RecyclerView
To set up a RecyclerView, follow these steps:
- Add the RecyclerView widget to your layout XML file.
- Create a layout file for the individual list items.
- Create a ViewHolder class to hold references to the views in each item layout.
- Extend the RecyclerView.Adapter class and implement the required methods.
- Instantiate your adapter and set it to the RecyclerView.
- Choose and set a LayoutManager on the RecyclerView.
Here’s a simple example:
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> {
private List<String> mDataSet;
public static class MyViewHolder extends RecyclerView.ViewHolder {
public TextView textView;
public MyViewHolder(View v) {
super(v);
textView = v.findViewById(R.id.textView);
}
}
public MyAdapter(List<String> dataSet) {
mDataSet = dataSet;
}
@Override
public MyViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View v = LayoutInflater.from(parent.getContext())
.inflate(R.layout.my_text_view, parent, false);
return new MyViewHolder(v);
}
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.textView.setText(mDataSet.get(position));
}
@Override
public int getItemCount() {
return mDataSet.size();
}
}
In this example, a simple Adapter is created for a list of strings. The ViewHolder class holds a reference to a TextView, and the Adapter binds each string from the data set to the TextView.
Optimizing RecyclerView Performance
RecyclerView is designed to be highly efficient, but there are still best practices to follow to ensure optimal performance:
- Use the ViewHolder pattern: This is already enforced in RecyclerView, but ensure your ViewHolder is efficient, holding all necessary references.
- Use DiffUtil: For large data sets, consider using DiffUtil to calculate the differences between old and new lists and update only the changed items.
- Recycling and Reusing Views: The RecyclerView reuses item views that are no longer visible, so avoid creating new views unnecessarily.
- Item Animation: Use item animations sparingly, as they can impact performance.
Handling User Interactions
Handling user interactions in a RecyclerView is straightforward. You can set listeners in the ViewHolder or directly in the Adapter’s onBindViewHolder
method. For example, you can handle item clicks like this:
@Override
public void onBindViewHolder(MyViewHolder holder, int position) {
holder.textView.setText(mDataSet.get(position));
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle item click
}
});
}
For more complex interactions, consider using interfaces or event buses to communicate between the Adapter and the rest of your application.
Conclusion
RecyclerView is a powerful and flexible tool for displaying lists of data in Android applications. By understanding how to implement Adapters, ViewHolders, and LayoutManagers, you can create efficient, responsive, and visually appealing lists. Additionally, by adhering to best practices and optimizing your RecyclerView usage, you can ensure that your applications remain performant even with large data sets. As you continue to develop with RecyclerView, you'll find it to be an indispensable component in your Android development toolkit.
Now answer the exercise about the content:
What is the primary advantage of using RecyclerView over ListView in Android app development?
You are right! Congratulations, now go to the next page
You missed! Try again.
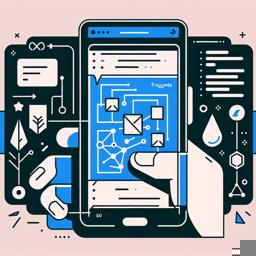
Next page of the Free Ebook: