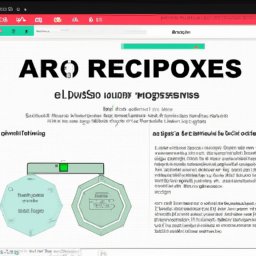
7.13. Working with Routes in ExpressJS: Working with Route Authorization
Página 59
ExpressJS is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. One of the most powerful features of ExpressJS is its ability to manage routes. In this chapter, we'll explore how to work with routes in ExpressJS, with a special focus on working with authorization in routes.
Routes are an essential aspect of any web application. They determine how an application responds to a client request for a specific address. In ExpressJS, routes are defined by attaching route handlers to application instances.
To begin with, let's first understand how to define a basic route. Suppose we want to create a route to our app's homepage. We would do it like this:
app.get('/', function(req, res) { res.send('Welcome to the home page!'); });
Here, 'app' is an instance of express, 'get' is the HTTP method we are dealing with, '/' is the route path, and the function is the route handler that is executed when the route is matched .
Now, let's talk about authorization. Authorization is a process that verifies that the user has permission to perform the requested action. In the context of routes, authorization can be used to restrict access to certain routes based on user authentication.
To implement authorization in our routes, we can use middleware. Middleware is a function that has access to the request object (req), the response object (res), and the next middleware function in the application's request-response cycle.
We can define an authorization middleware as follows:
function verifyAuthorization(req, res, next) { if (req.user.isAuthorized) { next(); } else { res.status(403).send('Access denied'); } }
Here, 'verificaAuthorizacao' is the middleware we are defining. It verifies that the user is authorized by checking the 'estaAuthorizado' property of the 'user' object in the request. If the user is authorized, it calls the next middleware function. Otherwise, it sends a response with status 403 and an 'Access Denied' message.
Now, we can use this middleware in our routes to restrict access. For example, if we wanted to restrict access to the '/secret' route, we'd do it like this:
app.get('/secret', verifyAuthorization, function(req, res) { res.send('Welcome to the secret page!'); });
Here, 'checkAuthorization' is passed as the second argument to 'app.get'. This means that it will run before the route handler whenever the '/secret' route is accessed. If the user is not authorized, he will receive a response with status 403 and the message 'Access Denied'. If the user is authorized, the route handler will run and the user will see the message 'Welcome to the secret page!'.
In summary, routes are an essential aspect of web application development with ExpressJS. They allow you to define how your application should respond to different requests. Authorization is an important aspect of routes that allows you to restrict access based on user authentication. By using middleware, you can easily implement authorization in your routes.
Now answer the exercise about the content:
What is ExpressJS and how does it manage routes in a web application?
You are right! Congratulations, now go to the next page
You missed! Try again.
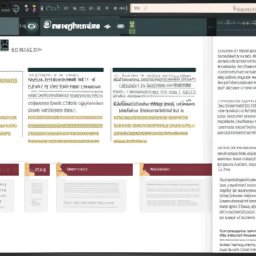
Next page of the Free Ebook: