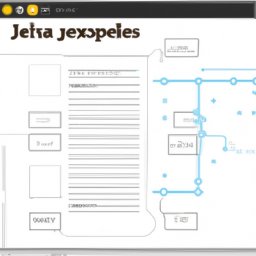
7.10. Working with routes in ExpressJS: Validating data in routes
Page 56 | Listen in audio
ExpressJS is a minimalist and flexible Node.js networking application framework that provides a robust set of features for building web and mobile applications. One of the main features of ExpressJS is its ease of configuring routes, which is crucial for creating APIs. When working with routes in ExpressJS, it is important to ensure that the data passed through these routes is validated. Data validation is an important step in ensuring data integrity and application security.
To start working with routes in ExpressJS, we first need to install and configure ExpressJS. This can easily be done using the npm package manager, which is installed along with Node.js. Once ExpressJS is installed, we can create a new JavaScript file, usually called 'app.js', and import ExpressJS into this file.
Routes in ExpressJS are defined using methods of the Express application object, where each method corresponds to an HTTP method. For example, app.get() is used to define a route for GET requests and app.post() for POST requests. Each route has a callback function that is called when the route is matched. This callback function takes two arguments: a request object and a response object.
To illustrate data validation in routes, let's consider an example of an API that receives a POST request to create a new user. User data is sent in the body of the request and may include fields such as name, email and password. Before processing this data and creating the new user, we must validate the data to ensure it is the correct type and format.
app.post('/users', function(req, res) { // Get user data from request body var user = req.body; // Validate user data if (!user.name || typeof user.name !== 'string' || user.name.length > 50) { // If the username is not valid, send an error response res.status(400).send('Invalid user name'); return; } if (!user.email || !validateEmail(user.email)) { // If the user's email is not valid, send an error response res.status(400).send('Invalid user email'); return; } if (!user.password || typeof user.password !== 'string' || user.password.length < 6) { // If the user's password is not valid, send an error response res.status(400).send('Invalid user password'); return; } // If all data is valid, create the new user var newUser = createUser(user); // Send the response with the new user res.status(201).send(newUser); });
In the example above, we validate the user data by checking that the name is a string and not too long, that the email is a valid email address, and that the password is a string and has at least 6 characters. If any of these tests fail, we send a response with a 400 (Bad Request) status code and an error message. If all tests pass, we create the new user and send a response with the new user and a 201 (Created) status code.
In addition to validating the data in routes, it is also good practice to handle route errors. This can be done using the app.use() method to add error handling middleware. This middleware must be added after all other routes and middleware, and it has four arguments: an error, a request object, a response object, and a next function. If an error is passed to the next function in any route or middleware, it will be handled by the error handling middleware.
Working with routes in ExpressJS and validating data in routes is a fundamental aspect of creating APIs in NodeJS. By ensuring that data passed through routes is validated, we can improve the security of our application and ensure that the data we are processing is the correct type and format.
Now answer the exercise about the content:
What is the role of data validation in routes in ExpressJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
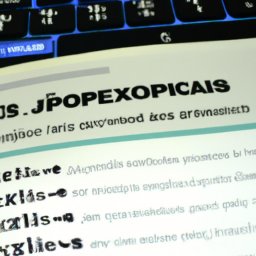
Next page of the Free Ebook: