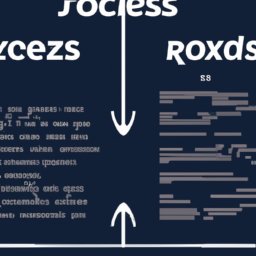
7.7. Working with routes in ExpressJS: Organizing routes in separate files
Página 53
One of the main advantages of using ExpressJS when working with NodeJS is how easy it is to handle routes. However, as your application grows, managing routes can become an overwhelming task. Fortunately, ExpressJS lets you organize routes into separate files, which makes the process much more manageable and scalable.
To begin with, let's understand what routes are. Routes are a fundamental part of any web application. They are responsible for directing users to different parts of the application based on URL and HTTP method. For example, if a user accesses 'www.yoursite.com/contact', the route '/contact' will be responsible for showing the contact page.
In ExpressJS, routes are defined using the 'app.route()' method, where 'app' is an instance of Express. For example, the '/contact' route can be defined as follows:
app.get('/contact', function(req, res){ res.send('Contact page'); });
This works well for small applications, but as the application grows and more routes are added, the main server file can become very large and difficult to manage. This is where organizing routes into separate files comes in handy.
To organize routes into separate files, you need to create a new file for each set of routes. For example, you might have a 'contact.js' file for all contact-related routes, a 'usuarios.js' file for all user-related routes, and so on.
In each route file, you need to create a new instance of the Express router using 'express.Router()'. After that, you can define the routes as you normally would. For example, the 'contact.js' file might look like this:
var express = require('express'); var router = express.Router(); router.get('/', function(req, res){ res.send('Contact page'); }); module.exports = router;
Notice that at the end of the file, we export the router using 'module.exports'. This allows us to import the router into our main server file.
In our main server file, we can import the router using 'require()'. After that, we can use the 'app.use()' method to add the routes to our application. For example:
var express = require('express'); var app = express(); var contactRoute = require('./contact'); app.use('/contact', routecontact);
This will add all the routes defined in 'contact.js' to our application, under the path '/contact'. So the route we defined in 'contact.js' as '/' will now be accessible from '/contact'.
Organizing routes this way has several advantages. First, it makes the code much cleaner and easier to manage. Second, it allows you to split your application into logical modules, which is good and recommended programming practice. Third, it makes your application more scalable as you can easily add more route files as your application grows.
In summary, working with routes in ExpressJS is a simple task, but it can become complex as your application grows. Fortunately, ExpressJS provides an easy way to organize your routes into separate files, making the process much more manageable and scalable.
Now answer the exercise about the content:
What is the main advantage of organizing routes in separate files in ExpressJS?
You are right! Congratulations, now go to the next page
You missed! Try again.
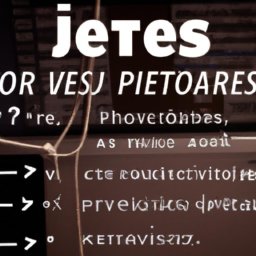
Next page of the Free Ebook: